A collection of Analog Devices drivers for the mbed platform
Embed:
(wiki syntax)
Show/hide line numbers
CN0391.h
00001 #ifndef _CN0391_H_ 00002 #define _CN0391_H_ 00003 #include "AD7124.h" 00004 #include "Thermocouple.h" 00005 00006 00007 /** 00008 * @brief Thermocouple_Channel class 00009 */ 00010 class Thermocouple_Channel 00011 { 00012 private: 00013 public: 00014 /** 00015 * @brief Thermocouple channel constructor 00016 */ 00017 Thermocouple_Channel(); 00018 /** 00019 * @brief Constructs thermocouple channel using thermocouple type t 00020 */ 00021 Thermocouple_Channel(Thermocouple *t); 00022 00023 Thermocouple *t; 00024 uint16_t thermocouple_channel; 00025 uint16_t rtd_channel; 00026 uint16_t calibration_channel; 00027 float calibration_current; 00028 00029 /** 00030 * @brief gets thermocouple type 00031 * @return thermocouple type 00032 */ 00033 Thermocouple* get_thermocouple_type(); 00034 00035 /** 00036 * @brief sets new thermocouple type 00037 * @param new_t new thermocouple type 00038 */ 00039 void set_thermocouple_type(Thermocouple* new_t); 00040 00041 /** 00042 * @brief sets up thermocouple channel 00043 * @param new_t thermocouple type 00044 * @param thermocouple_channel - thermocouple ADC channel 00045 * @param rtd_channel - RTD ADC channel 00046 * @param calibration_channel - ADC channel used in calibration 00047 * 00048 */ 00049 void setup_channel(Thermocouple* new_t, uint16_t thermocouple_channel, uint16_t rtd_channel, uint16_t calibration_channel); 00050 }; 00051 00052 class CN0391 00053 { 00054 private: 00055 public: 00056 CN0391(PinName cs); 00057 00058 typedef enum { 00059 CHANNEL_P1 = 0, 00060 CHANNEL_P2, 00061 CHANNEL_P3, 00062 CHANNEL_P4 00063 } channel_t; 00064 00065 Thermocouple_Channel tc[4]; 00066 00067 /** 00068 * @brief sets channel with thermocouple type 00069 * @param ch - channel 00070 * @param new_t - thermocouple type 00071 */ 00072 void set_thermocouple_type(channel_t ch, Thermocouple* new_t); 00073 00074 /** 00075 * @brief Reads thermocouple channel 00076 * @param ch - channel 00077 */ 00078 float read_channel(channel_t ch); 00079 00080 /** 00081 * @brief Calibrate channel 00082 * @param ch - channel 00083 */ 00084 float calibrate(channel_t ch); 00085 00086 00087 /** 00088 * @brief converts ADC counts to voltage 00089 * @param data - ADC counts 00090 * @return voltage 00091 */ 00092 float data_to_voltage(uint32_t data); 00093 00094 /** 00095 * @brief enables thermocouple channel 00096 * @param channel 00097 */ 00098 void enable_channel(int channel); 00099 00100 /** 00101 * @brief disables thermocouple channel 00102 * @param channel 00103 */ 00104 void disable_channel(int channel); 00105 00106 /** 00107 * @brief enables ADC current source 00108 * @param current_source_channel 00109 */ 00110 void enable_current_source(int current_source_channel); 00111 00112 /** 00113 * @brief starts ADC single conversion 00114 */ 00115 void start_single_conversion(); 00116 00117 /** 00118 * @brief resets the ADC 00119 */ 00120 void reset(); 00121 00122 /** 00123 * @brief Performs ADC setup 00124 */ 00125 void setup(); 00126 00127 /** 00128 * @brief Initializes the CN0391 shield 00129 */ 00130 void init(); 00131 00132 AD7124 ad7124; 00133 00134 00135 }; 00136 #endif
Generated on Tue Jul 12 2022 17:59:52 by
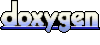