A collection of Analog Devices drivers for the mbed platform
Embed:
(wiki syntax)
Show/hide line numbers
ADT7310.h
00001 00002 #ifndef ADT7310_H 00003 #define ADT7310_H 00004 00005 #include "mbed.h" 00006 00007 /** 00008 * @brief Analog Devices ADT7310 temperature sensor 00009 */ 00010 class ADT7310 00011 { 00012 public: 00013 00014 00015 #define ADT7310_READ (1<<6) 00016 #define ADT7310_WRITE (0) 00017 #define ADT7310_DUMMY (0) 00018 #define ADT7310_STATUS (0) 00019 #define ADT7310_CONFIG (1) 00020 #define ADT7310_TEMP (2) 00021 #define ADT7310_ID (3) 00022 #define ADT7310_TCRIT (4) 00023 #define ADT7310_THYST (5) 00024 #define ADT7310_THIGH (6) 00025 #define ADT7310_TLOW (7) 00026 00027 00028 /** 00029 * @brief ADT7310 class 00030 * @param CS - chipselect pin 00031 * @param MOSI - MOSI pin 00032 * @param MISO - MISO pin 00033 * @param SCK - Clock pin 00034 */ 00035 ADT7310(PinName CS = SPI_CS, PinName MOSI = SPI_MOSI, PinName MISO = SPI_MISO, PinName SCK = SPI_SCK); 00036 00037 /** 00038 * @brief resets the ADT7310 00039 */ 00040 void reset(); 00041 00042 /** 00043 * @brief reads status register of the temperature sensor 00044 * @return value of the status register 00045 */ 00046 uint8_t read_status(); 00047 00048 /** 00049 * @brief writes configuration register of the temperature sensor 00050 * @param data - data to be written 00051 */ 00052 void write_config(uint8_t data); 00053 00054 /** 00055 * @brief issues a conversion to the temperature sensor 00056 */ 00057 void start_single_conversion(); 00058 00059 /** 00060 * @brief reads configuration register 00061 * @return configuration register value 00062 */ 00063 uint8_t read_config(); 00064 00065 /** 00066 * @brief reads the temperature 00067 * @return temperature 00068 */ 00069 uint16_t read_temp(); 00070 00071 /** 00072 * @brief writes temperature setpoints 00073 * @param setpoint - setpoint register 00074 * @param data - data to be written to the setpoint register 00075 */ 00076 void write_temp_setpoint(uint8_t setpoint, uint16_t data); 00077 00078 void spi_write(uint8_t *data, uint8_t size); 00079 void spi_read(uint8_t *data, uint8_t size); 00080 //DigitalIn miso; 00081 DigitalOut cs; 00082 SPI adt7310; 00083 private: 00084 const uint8_t SPI_MODE = 0x03; 00085 }; 00086 00087 #endif
Generated on Tue Jul 12 2022 17:59:52 by
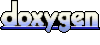