A collection of Analog Devices drivers for the mbed platform
Embed:
(wiki syntax)
Show/hide line numbers
AD7124.h
Go to the documentation of this file.
00001 /** 00002 * @file AD7124.h 00003 * @brief Header file for AD7790 ADC 00004 * @author Analog Devices Inc. 00005 * 00006 * For support please go to: 00007 * Github: https://github.com/analogdevicesinc/mbed-adi 00008 * Support: https://ez.analog.com/community/linux-device-drivers/microcontroller-no-os-drivers 00009 * Product: http://www.analog.com/AD7124 00010 * More: https://wiki.analog.com/resources/tools-software/mbed-drivers-all 00011 00012 ******************************************************************************** 00013 * Copyright 2016(c) Analog Devices, Inc. 00014 * 00015 * All rights reserved. 00016 * 00017 * Redistribution and use in source and binary forms, with or without 00018 * modification, are permitted provided that the following conditions are met: 00019 * - Redistributions of source code must retain the above copyright 00020 * notice, this list of conditions and the following disclaimer. 00021 * - Redistributions in binary form must reproduce the above copyright 00022 * notice, this list of conditions and the following disclaimer in 00023 * the documentation and/or other materials provided with the 00024 * distribution. 00025 * - Neither the name of Analog Devices, Inc. nor the names of its 00026 * contributors may be used to endorse or promote products derived 00027 * from this software without specific prior written permission. 00028 * - The use of this software may or may not infringe the patent rights 00029 * of one or more patent holders. This license does not release you 00030 * from the requirement that you obtain separate licenses from these 00031 * patent holders to use this software. 00032 * - Use of the software either in source or binary form, must be run 00033 * on or directly connected to an Analog Devices Inc. component. 00034 * 00035 * THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00036 * IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00037 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00038 * IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00039 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00040 * LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00041 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00042 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00043 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00044 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00045 * 00046 ********************************************************************************/ 00047 00048 #ifndef AD7790_H 00049 #define AD7790_H 00050 00051 #include "mbed.h" 00052 00053 /** 00054 * Comment this line if you want to turn off the debug mode. 00055 * The debug mode will send a message if an exception occurs within AD7790 driver 00056 */ 00057 00058 #define AD7124_DEBUG_MODE 00059 00060 /** 00061 * @brief Analog Devices AD7790 SPI 16-bit Buffered Sigma-Delta ADC 00062 */ 00063 class AD7124 00064 { 00065 public: 00066 enum ad7124_registers { 00067 AD7124_Status = 0x00, 00068 AD7124_ADC_Control, 00069 AD7124_Data, 00070 AD7124_IOCon1, 00071 AD7124_IOCon2, 00072 AD7124_ID, 00073 AD7124_Error, 00074 AD7124_Error_En, 00075 AD7124_Mclk_Count, 00076 AD7124_Channel_0, 00077 AD7124_Channel_1, 00078 AD7124_Channel_2, 00079 AD7124_Channel_3, 00080 AD7124_Channel_4, 00081 AD7124_Channel_5, 00082 AD7124_Channel_6, 00083 AD7124_Channel_7, 00084 AD7124_Channel_8, 00085 AD7124_Channel_9, 00086 AD7124_Channel_10, 00087 AD7124_Channel_11, 00088 AD7124_Channel_12, 00089 AD7124_Channel_13, 00090 AD7124_Channel_14, 00091 AD7124_Channel_15, 00092 AD7124_Config_0, 00093 AD7124_Config_1, 00094 AD7124_Config_2, 00095 AD7124_Config_3, 00096 AD7124_Config_4, 00097 AD7124_Config_5, 00098 AD7124_Config_6, 00099 AD7124_Config_7, 00100 AD7124_Filter_0, 00101 AD7124_Filter_1, 00102 AD7124_Filter_2, 00103 AD7124_Filter_3, 00104 AD7124_Filter_4, 00105 AD7124_Filter_5, 00106 AD7124_Filter_6, 00107 AD7124_Filter_7, 00108 AD7124_Offset_0, 00109 AD7124_Offset_1, 00110 AD7124_Offset_2, 00111 AD7124_Offset_3, 00112 AD7124_Offset_4, 00113 AD7124_Offset_5, 00114 AD7124_Offset_6, 00115 AD7124_Offset_7, 00116 AD7124_Gain_0, 00117 AD7124_Gain_1, 00118 AD7124_Gain_2, 00119 AD7124_Gain_3, 00120 AD7124_Gain_4, 00121 AD7124_Gain_5, 00122 AD7124_Gain_6, 00123 AD7124_Gain_7, 00124 AD7124_REG_NO 00125 }; 00126 private: 00127 enum { 00128 AD7124_RW = 1, /* Read and Write */ 00129 AD7124_R = 2, /* Read only */ 00130 AD7124_W = 3, /* Write only */ 00131 } ad7124_reg_access; 00132 00133 /*! Device register info */ 00134 typedef struct _ad7124_st_reg { 00135 int32_t addr; 00136 int32_t value; 00137 int32_t size; 00138 int32_t rw; 00139 } ad7124_st_reg; 00140 00141 00142 /*! Array holding the info for the ad7124 registers - address, initial value, 00143 size and access type. */ 00144 ad7124_st_reg ad7124_regs[57] = { 00145 {0x00, 0x00, 1, 2}, /* AD7124_Status */ 00146 {0x01, 0x0000, 2, 1}, /* AD7124_ADC_Control */ 00147 {0x02, 0x0000, 3, 2}, /* AD7124_Data */ 00148 {0x03, 0x0000, 3, 1}, /* AD7124_IOCon1 */ 00149 {0x04, 0x0000, 2, 1}, /* AD7124_IOCon2 */ 00150 {0x05, 0x12, 1, 2}, /* AD7124_ID */ 00151 {0x06, 0x0000, 3, 2}, /* AD7124_Error */ 00152 {0x07, 0x0400, 3, 1}, /* AD7124_Error_En */ 00153 {0x08, 0x00, 1, 2}, /* AD7124_Mclk_Count */ 00154 {0x09, 0x8001, 2, 1}, /* AD7124_Channel_0 */ 00155 {0x0A, 0x0001, 2, 1}, /* AD7124_Channel_1 */ 00156 {0x0B, 0x0001, 2, 1}, /* AD7124_Channel_2 */ 00157 {0x0C, 0x0001, 2, 1}, /* AD7124_Channel_3 */ 00158 {0x0D, 0x0001, 2, 1}, /* AD7124_Channel_4 */ 00159 {0x0E, 0x0001, 2, 1}, /* AD7124_Channel_5 */ 00160 {0x0F, 0x0001, 2, 1}, /* AD7124_Channel_6 */ 00161 {0x10, 0x0001, 2, 1}, /* AD7124_Channel_7 */ 00162 {0x11, 0x0001, 2, 1}, /* AD7124_Channel_8 */ 00163 {0x12, 0x0001, 2, 1}, /* AD7124_Channel_9 */ 00164 {0x13, 0x0001, 2, 1}, /* AD7124_Channel_10 */ 00165 {0x14, 0x0001, 2, 1}, /* AD7124_Channel_11 */ 00166 {0x15, 0x0001, 2, 1}, /* AD7124_Channel_12 */ 00167 {0x16, 0x0001, 2, 1}, /* AD7124_Channel_13 */ 00168 {0x17, 0x0001, 2, 1}, /* AD7124_Channel_14 */ 00169 {0x18, 0x0001, 2, 1}, /* AD7124_Channel_15 */ 00170 {0x19, 0x0860, 2, 1}, /* AD7124_Config_0 */ 00171 {0x1A, 0x0860, 2, 1}, /* AD7124_Config_1 */ 00172 {0x1B, 0x0860, 2, 1}, /* AD7124_Config_2 */ 00173 {0x1C, 0x0860, 2, 1}, /* AD7124_Config_3 */ 00174 {0x1D, 0x0860, 2, 1}, /* AD7124_Config_4 */ 00175 {0x1E, 0x0860, 2, 1}, /* AD7124_Config_5 */ 00176 {0x1F, 0x0860, 2, 1}, /* AD7124_Config_6 */ 00177 {0x20, 0x0860, 2, 1}, /* AD7124_Config_7 */ 00178 {0x21, 0x060180, 3, 1}, /* AD7124_Filter_0 */ 00179 {0x22, 0x060180, 3, 1}, /* AD7124_Filter_1 */ 00180 {0x23, 0x060180, 3, 1}, /* AD7124_Filter_2 */ 00181 {0x24, 0x060180, 3, 1}, /* AD7124_Filter_3 */ 00182 {0x25, 0x060180, 3, 1}, /* AD7124_Filter_4 */ 00183 {0x26, 0x060180, 3, 1}, /* AD7124_Filter_5 */ 00184 {0x27, 0x060180, 3, 1}, /* AD7124_Filter_6 */ 00185 {0x28, 0x060180, 3, 1}, /* AD7124_Filter_7 */ 00186 {0x29, 0x800000, 3, 1}, /* AD7124_Offset_0 */ 00187 {0x2A, 0x800000, 3, 1}, /* AD7124_Offset_1 */ 00188 {0x2B, 0x800000, 3, 1}, /* AD7124_Offset_2 */ 00189 {0x2C, 0x800000, 3, 1}, /* AD7124_Offset_3 */ 00190 {0x2D, 0x800000, 3, 1}, /* AD7124_Offset_4 */ 00191 {0x2E, 0x800000, 3, 1}, /* AD7124_Offset_5 */ 00192 {0x2F, 0x800000, 3, 1}, /* AD7124_Offset_6 */ 00193 {0x30, 0x800000, 3, 1}, /* AD7124_Offset_7 */ 00194 {0x31, 0x500000, 3, 1}, /* AD7124_Gain_0 */ 00195 {0x32, 0x500000, 3, 1}, /* AD7124_Gain_1 */ 00196 {0x33, 0x500000, 3, 1}, /* AD7124_Gain_2 */ 00197 {0x34, 0x500000, 3, 1}, /* AD7124_Gain_3 */ 00198 {0x35, 0x500000, 3, 1}, /* AD7124_Gain_4 */ 00199 {0x36, 0x500000, 3, 1}, /* AD7124_Gain_5 */ 00200 {0x37, 0x500000, 3, 1}, /* AD7124_Gain_6 */ 00201 {0x38, 0x500000, 3, 1}, /* AD7124_Gain_7 */ 00202 }; 00203 00204 00205 /* AD7124 Register Map */ 00206 enum AD7124_reg_map { 00207 COMM_REG = 0x00, 00208 STATUS_REG = 0x00, 00209 ADC_CTRL_REG = 0x01, 00210 DATA_REG = 0x02, 00211 IO_CTRL1_REG = 0x03, 00212 IO_CTRL2_REG = 0x04, 00213 ID_REG = 0x05, 00214 ERR_REG = 0x06, 00215 ERREN_REG = 0x07, 00216 CH0_MAP_REG = 0x09, 00217 CH1_MAP_REG = 0x0A, 00218 CH2_MAP_REG = 0x0B, 00219 CH3_MAP_REG = 0x0C, 00220 CH4_MAP_REG = 0x0D, 00221 CH5_MAP_REG = 0x0E, 00222 CH6_MAP_REG = 0x0F, 00223 CH7_MAP_REG = 0x10, 00224 CH8_MAP_REG = 0x11, 00225 CH9_MAP_REG = 0x12, 00226 CH10_MAP_REG = 0x13, 00227 CH11_MAP_REG = 0x14, 00228 CH12_MAP_REG = 0x15, 00229 CH13_MAP_REG = 0x16, 00230 CH14_MAP_REG = 0x17, 00231 CH15_MAP_REG = 0x18, 00232 CFG0_REG = 0x19, 00233 CFG1_REG = 0x1A, 00234 CFG2_REG = 0x1B, 00235 CFG3_REG = 0x1C, 00236 CFG4_REG = 0x1D, 00237 CFG5_REG = 0x1E, 00238 CFG6_REG = 0x1F, 00239 CFG7_REG = 0x20, 00240 FILT0_REG = 0x21, 00241 FILT1_REG = 0x22, 00242 FILT2_REG = 0x23, 00243 FILT3_REG = 0x24, 00244 FILT4_REG = 0x25, 00245 FILT5_REG = 0x26, 00246 FILT6_REG = 0x27, 00247 FILT7_REG = 0x28, 00248 OFFS0_REG = 0x29, 00249 OFFS1_REG = 0x2A, 00250 OFFS2_REG = 0x2B, 00251 OFFS3_REG = 0x2C, 00252 OFFS4_REG = 0x2D, 00253 OFFS5_REG = 0x2E, 00254 OFFS6_REG = 0x2F, 00255 OFFS7_REG = 0x30, 00256 GAIN0_REG = 0x31, 00257 GAIN1_REG = 0x32, 00258 GAIN2_REG = 0x33, 00259 GAIN3_REG = 0x34, 00260 GAIN4_REG = 0x35, 00261 GAIN5_REG = 0x36, 00262 GAIN6_REG = 0x37, 00263 GAIN7_REG = 0x38, 00264 }; 00265 00266 /* Communication Register bits */ 00267 #define AD7124_COMM_REG_WEN (0 << 7) 00268 #define AD7124_COMM_REG_WR (0 << 6) 00269 #define AD7124_COMM_REG_RD (1 << 6) 00270 #define AD7124_COMM_REG_RA(x) ((x) & 0x3F) 00271 00272 /* Status Register bits */ 00273 #define AD7124_STATUS_REG_RDY (1 << 7) 00274 #define AD7124_STATUS_REG_ERROR_FLAG (1 << 6) 00275 #define AD7124_STATUS_REG_POR_FLAG (1 << 4) 00276 #define AD7124_STATUS_REG_CH_ACTIVE(x) ((x) & 0xF) 00277 00278 /* ADC_Control Register bits */ 00279 #define AD7124_ADC_CTRL_REG_DOUT_RDY_DEL (1 << 12) 00280 #define AD7124_ADC_CTRL_REG_CONT_READ (1 << 11) 00281 #define AD7124_ADC_CTRL_REG_DATA_STATUS (1 << 10) 00282 #define AD7124_ADC_CTRL_REG_CS_EN (1 << 9) 00283 #define AD7124_ADC_CTRL_REG_REF_EN (1 << 8) 00284 #define AD7124_ADC_CTRL_REG_POWER_MODE(x) (((x) & 0x3) << 6) 00285 #define AD7124_ADC_CTRL_REG_MODE(x) (((x) & 0xF) << 2) 00286 #define AD7124_ADC_CTRL_REG_CLK_SEL(x) (((x) & 0x3) << 0) 00287 00288 /* IO_Control_1 Register bits */ 00289 #define AD7124_IO_CTRL1_REG_GPIO_DAT2 (1 << 23) 00290 #define AD7124_IO_CTRL1_REG_GPIO_DAT1 (1 << 22) 00291 #define AD7124_IO_CTRL1_REG_GPIO_CTRL2 (1 << 19) 00292 #define AD7124_IO_CTRL1_REG_GPIO_CTRL1 (1 << 18) 00293 #define AD7124_IO_CTRL1_REG_PDSW (1 << 15) 00294 #define AD7124_IO_CTRL1_REG_IOUT1(x) (((x) & 0x7) << 11) 00295 #define AD7124_IO_CTRL1_REG_IOUT0(x) (((x) & 0x7) << 8) 00296 #define AD7124_IO_CTRL1_REG_IOUT_CH1(x) (((x) & 0xF) << 4) 00297 #define AD7124_IO_CTRL1_REG_IOUT_CH0(x) (((x) & 0xF) << 0) 00298 00299 /*IO_Control_1 AD7124-8 specific bits */ 00300 #define AD7124_8_IO_CTRL1_REG_GPIO_DAT4 (1 << 23) 00301 #define AD7124_8_IO_CTRL1_REG_GPIO_DAT3 (1 << 22) 00302 #define AD7124_8_IO_CTRL1_REG_GPIO_DAT2 (1 << 21) 00303 #define AD7124_8_IO_CTRL1_REG_GPIO_DAT1 (1 << 20) 00304 #define AD7124_8_IO_CTRL1_REG_GPIO_CTRL4 (1 << 19) 00305 #define AD7124_8_IO_CTRL1_REG_GPIO_CTRL3 (1 << 18) 00306 #define AD7124_8_IO_CTRL1_REG_GPIO_CTRL2 (1 << 17) 00307 #define AD7124_8_IO_CTRL1_REG_GPIO_CTRL1 (1 << 16) 00308 00309 /* IO_Control_2 Register bits */ 00310 #define AD7124_IO_CTRL2_REG_GPIO_VBIAS7 (1 << 15) 00311 #define AD7124_IO_CTRL2_REG_GPIO_VBIAS6 (1 << 14) 00312 #define AD7124_IO_CTRL2_REG_GPIO_VBIAS5 (1 << 11) 00313 #define AD7124_IO_CTRL2_REG_GPIO_VBIAS4 (1 << 10) 00314 #define AD7124_IO_CTRL2_REG_GPIO_VBIAS3 (1 << 5) 00315 #define AD7124_IO_CTRL2_REG_GPIO_VBIAS2 (1 << 4) 00316 #define AD7124_IO_CTRL2_REG_GPIO_VBIAS1 (1 << 1) 00317 #define AD7124_IO_CTRL2_REG_GPIO_VBIAS0 (1) 00318 00319 /*IO_Control_2 AD7124-8 specific bits */ 00320 #define AD7124_8_IO_CTRL2_REG_GPIO_VBIAS15 (1 << 15) 00321 #define AD7124_8_IO_CTRL2_REG_GPIO_VBIAS14 (1 << 14) 00322 #define AD7124_8_IO_CTRL2_REG_GPIO_VBIAS13 (1 << 13) 00323 #define AD7124_8_IO_CTRL2_REG_GPIO_VBIAS12 (1 << 12) 00324 #define AD7124_8_IO_CTRL2_REG_GPIO_VBIAS11 (1 << 11) 00325 #define AD7124_8_IO_CTRL2_REG_GPIO_VBIAS10 (1 << 10) 00326 #define AD7124_8_IO_CTRL2_REG_GPIO_VBIAS9 (1 << 9) 00327 #define AD7124_8_IO_CTRL2_REG_GPIO_VBIAS8 (1 << 8) 00328 #define AD7124_8_IO_CTRL2_REG_GPIO_VBIAS7 (1 << 7) 00329 #define AD7124_8_IO_CTRL2_REG_GPIO_VBIAS6 (1 << 6) 00330 #define AD7124_8_IO_CTRL2_REG_GPIO_VBIAS5 (1 << 5) 00331 #define AD7124_8_IO_CTRL2_REG_GPIO_VBIAS4 (1 << 4) 00332 #define AD7124_8_IO_CTRL2_REG_GPIO_VBIAS3 (1 << 3) 00333 #define AD7124_8_IO_CTRL2_REG_GPIO_VBIAS2 (1 << 2) 00334 #define AD7124_8_IO_CTRL2_REG_GPIO_VBIAS1 (1 << 1) 00335 #define AD7124_8_IO_CTRL2_REG_GPIO_VBIAS0 (1 << 0) 00336 00337 /* ID Register bits */ 00338 #define AD7124_ID_REG_DEVICE_ID(x) (((x) & 0xF) << 4) 00339 #define AD7124_ID_REG_SILICON_REV(x) (((x) & 0xF) << 0) 00340 00341 /* Error Register bits */ 00342 #define AD7124_ERR_REG_LDO_CAP_ERR (1 << 19) 00343 #define AD7124_ERR_REG_ADC_CAL_ERR (1 << 18) 00344 #define AD7124_ERR_REG_ADC_CONV_ERR (1 << 17) 00345 #define AD7124_ERR_REG_ADC_SAT_ERR (1 << 16) 00346 #define AD7124_ERR_REG_AINP_OV_ERR (1 << 15) 00347 #define AD7124_ERR_REG_AINP_UV_ERR (1 << 14) 00348 #define AD7124_ERR_REG_AINM_OV_ERR (1 << 13) 00349 #define AD7124_ERR_REG_AINM_UV_ERR (1 << 12) 00350 #define AD7124_ERR_REG_REF_DET_ERR (1 << 11) 00351 #define AD7124_ERR_REG_DLDO_PSM_ERR (1 << 9) 00352 #define AD7124_ERR_REG_ALDO_PSM_ERR (1 << 7) 00353 #define AD7124_ERR_REG_SPI_IGNORE_ERR (1 << 6) 00354 #define AD7124_ERR_REG_SPI_SLCK_CNT_ERR (1 << 5) 00355 #define AD7124_ERR_REG_SPI_READ_ERR (1 << 4) 00356 #define AD7124_ERR_REG_SPI_WRITE_ERR (1 << 3) 00357 #define AD7124_ERR_REG_SPI_CRC_ERR (1 << 2) 00358 #define AD7124_ERR_REG_MM_CRC_ERR (1 << 1) 00359 00360 /* Error_En Register bits */ 00361 #define AD7124_ERREN_REG_MCLK_CNT_EN (1 << 22) 00362 #define AD7124_ERREN_REG_LDO_CAP_CHK_TEST_EN (1 << 21) 00363 #define AD7124_ERREN_REG_LDO_CAP_CHK(x) (((x) & 0x3) << 19) 00364 #define AD7124_ERREN_REG_ADC_CAL_ERR_EN (1 << 18) 00365 #define AD7124_ERREN_REG_ADC_CONV_ERR_EN (1 << 17) 00366 #define AD7124_ERREN_REG_ADC_SAT_ERR_EN (1 << 16) 00367 #define AD7124_ERREN_REG_AINP_OV_ERR_EN (1 << 15) 00368 #define AD7124_ERREN_REG_AINP_UV_ERR_EN (1 << 14) 00369 #define AD7124_ERREN_REG_AINM_OV_ERR_EN (1 << 13) 00370 #define AD7124_ERREN_REG_AINM_UV_ERR_EN (1 << 12) 00371 #define AD7124_ERREN_REG_REF_DET_ERR_EN (1 << 11) 00372 #define AD7124_ERREN_REG_DLDO_PSM_TRIP_TEST_EN (1 << 10) 00373 #define AD7124_ERREN_REG_DLDO_PSM_ERR_ERR (1 << 9) 00374 #define AD7124_ERREN_REG_ALDO_PSM_TRIP_TEST_EN (1 << 8) 00375 #define AD7124_ERREN_REG_ALDO_PSM_ERR_EN (1 << 7) 00376 #define AD7124_ERREN_REG_SPI_IGNORE_ERR_EN (1 << 6) 00377 #define AD7124_ERREN_REG_SPI_SCLK_CNT_ERR_EN (1 << 5) 00378 #define AD7124_ERREN_REG_SPI_READ_ERR_EN (1 << 4) 00379 #define AD7124_ERREN_REG_SPI_WRITE_ERR_EN (1 << 3) 00380 #define AD7124_ERREN_REG_SPI_CRC_ERR_EN (1 << 2) 00381 #define AD7124_ERREN_REG_MM_CRC_ERR_EN (1 << 1) 00382 00383 /* Channel Registers 0-15 bits */ 00384 #define AD7124_CH_MAP_REG_CH_ENABLE (1 << 15) 00385 #define AD7124_CH_MAP_REG_SETUP(x) (((x) & 0x7) << 12) 00386 #define AD7124_CH_MAP_REG_AINP(x) (((x) & 0x1F) << 5) 00387 #define AD7124_CH_MAP_REG_AINM(x) (((x) & 0x1F) << 0) 00388 00389 /* Configuration Registers 0-7 bits */ 00390 #define AD7124_CFG_REG_BIPOLAR (1 << 11) 00391 #define AD7124_CFG_REG_BURNOUT(x) (((x) & 0x3) << 9) 00392 #define AD7124_CFG_REG_REF_BUFP (1 << 8) 00393 #define AD7124_CFG_REG_REF_BUFM (1 << 7) 00394 #define AD7124_CFG_REG_AIN_BUFP (1 << 6) 00395 #define AD7124_CFG_REG_AINN_BUFM (1 << 5) 00396 #define AD7124_CFG_REG_REF_SEL(x) ((x) & 0x3) << 3 00397 #define AD7124_CFG_REG_PGA(x) (((x) & 0x7) << 0) 00398 00399 /* Filter Register 0-7 bits */ 00400 #define AD7124_FILT_REG_FILTER(x) ((uint32_t)((x) & 0x7) << 21) 00401 #define AD7124_FILT_REG_REJ60 ((uint32_t)1 << 20) 00402 #define AD7124_FILT_REG_POST_FILTER(x) ((uint32_t)((x) & 0x7) << 17) 00403 #define AD7124_FILT_REG_SINGLE_CYCLE ((uint32_t)1 << 16) 00404 #define AD7124_FILT_REG_FS(x) ((uint32_t)((x) & 0x7FF) << 0) 00405 00406 public: 00407 00408 /** SPI configuration & constructor */ 00409 AD7124( PinName CS = SPI_CS, PinName MOSI = SPI_MOSI, PinName MISO = SPI_MISO, PinName SCK = SPI_SCK); 00410 void frequency(int hz); 00411 00412 /** Low level SPI bus comm methods */ 00413 void reset(void); 00414 00415 void write_reg(uint8_t regAddress, uint8_t regValue); 00416 uint16_t write_spi(uint16_t data); 00417 uint16_t read_reg (uint8_t regAddress); 00418 bool get_miso(); 00419 00420 00421 int32_t Reset(); 00422 /* Reads and returns the value of a device register. */ 00423 uint32_t ReadDeviceRegister(enum ad7124_registers reg); 00424 00425 /* Writes the specified value to a device register. */ 00426 int32_t WriteDeviceRegister(enum ad7124_registers reg, uint32_t value); 00427 00428 /*! Reads the value of the specified register. */ 00429 int32_t ReadRegister(ad7124_st_reg* pReg); 00430 00431 /*! Writes the value of the specified register. */ 00432 int32_t WriteRegister(ad7124_st_reg reg); 00433 00434 /*! Reads the value of the specified register without a device state check. */ 00435 int32_t NoCheckReadRegister(ad7124_st_reg* pReg); 00436 00437 /*! Writes the value of the specified register without a device state check. */ 00438 int32_t NoCheckWriteRegister(ad7124_st_reg reg); 00439 00440 /*! Waits until the device can accept read and write user actions. */ 00441 int32_t WaitForSpiReady (uint32_t timeout); 00442 00443 /*! Waits until a new conversion result is available. */ 00444 int32_t WaitForConvReady (uint32_t timeout); 00445 00446 /*! Reads the conversion result from the device. */ 00447 int32_t ReadData (int32_t* pData); 00448 00449 /*! Computes the CRC checksum for a data buffer. */ 00450 uint8_t ComputeCRC8 (uint8_t* pBuf, uint8_t bufSize); 00451 00452 /*! Updates the device SPI interface settings. */ 00453 void UpdateDevSpiSettings (); 00454 00455 /*! Initializes the AD7124. */ 00456 int32_t Setup (); 00457 00458 uint8_t SPI_Read(uint8_t *data, uint8_t bytes_number); 00459 uint8_t SPI_Write(uint8_t *data, uint8_t bytes_number); 00460 00461 DigitalIn miso;///< DigitalIn must be initialized before SPI to prevent pin MUX overwrite 00462 SPI ad7124; ///< SPI instance of the AD7790 00463 DigitalOut cs; ///< DigitalOut instance for the chipselect of the AD7790 00464 00465 private: 00466 00467 00468 ad7124_st_reg *regs; // reg map 38 bytes ? 00469 uint8_t useCRC; // boolean ? 00470 int check_ready; // ? 00471 int spi_rdy_poll_cnt; // timer ? 00472 00473 const static uint8_t _SPI_MODE = 0x03; 00474 const static uint8_t _RESET = 0xFF; 00475 const static uint8_t _DUMMY_BYTE = 0xFF; 00476 const static uint16_t _READ_FLAG = 0x4000; 00477 const static uint8_t _DELAY_TIMING = 0x02; 00478 00479 #define AD7124_CRC8_POLYNOMIAL_REPRESENTATION 0x07 /* x8 + x2 + x + 1 */ 00480 #define AD7124_DISABLE_CRC 0 00481 #define AD7124_USE_CRC 1 00482 #define AD7124_READ_DATA 2 00483 00484 #define INVALID_VAL -1 /* Invalid argument */ 00485 #define COMM_ERR -2 /* Communication error on receive */ 00486 #define TIMEOUT -3 /* A timeout has occured */ 00487 00488 }; 00489 #endif 00490 00491
Generated on Tue Jul 12 2022 17:59:52 by
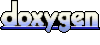