
Example Program for EVAL-AD7606
Dependencies: platform_drivers
app_config.h
00001 /*************************************************************************//** 00002 * @file app_config.h 00003 * @brief Header file for application configurations (platform-agnostic) 00004 ****************************************************************************** 00005 * Copyright (c) 2020-2021 Analog Devices, Inc. 00006 * All rights reserved. 00007 * 00008 * This software is proprietary to Analog Devices, Inc. and its licensors. 00009 * By using this software you agree to the terms of the associated 00010 * Analog Devices Software License Agreement. 00011 *****************************************************************************/ 00012 00013 #ifndef _APP_CONFIG_H_ 00014 #define _APP_CONFIG_H_ 00015 00016 /* List of supported platforms*/ 00017 #define MBED_PLATFORM 1 00018 00019 /* Select the active platform */ 00020 #define ACTIVE_PLATFORM MBED_PLATFORM 00021 00022 /******************************************************************************/ 00023 /***************************** Include Files **********************************/ 00024 /******************************************************************************/ 00025 00026 #include <stdint.h> 00027 00028 /******************************************************************************/ 00029 /********************** Macros and Constants Definition ***********************/ 00030 /******************************************************************************/ 00031 00032 // **** Note for User: ACTIVE_DEVICE selection ****// 00033 /* Define the device type here from the list of below device type defines 00034 * (one at a time. Defining more than one device can result into compile error). 00035 * e.g. #define DEV_AD7606B -> This will make AD7606B as an ACTIVE_DEVICE. 00036 * The ACTIVE_DEVICE is default set to AD7606B if device type is not defined. 00037 * */ 00038 00039 //#define DEV_AD7606B 00040 00041 #if defined(DEV_AD7605_4) 00042 #define ACTIVE_DEVICE ID_AD7605_4 00043 #define ACTIVE_DEVICE_NAME "ad7605-4" 00044 #elif defined(DEV_AD7606_4) 00045 #define ACTIVE_DEVICE ID_AD7606_4 00046 #define ACTIVE_DEVICE_NAME "ad7606-4" 00047 #elif defined(DEV_AD7606_6) 00048 #define ACTIVE_DEVICE ID_AD7606_6 00049 #define ACTIVE_DEVICE_NAME "ad7606-6" 00050 #elif defined(DEV_AD7606_8) 00051 #define ACTIVE_DEVICE ID_AD7606_8 00052 #define ACTIVE_DEVICE_NAME "ad7606-8" 00053 #elif defined(DEV_AD7606B) 00054 #define ACTIVE_DEVICE ID_AD7606B 00055 #define ACTIVE_DEVICE_NAME "ad7606b" 00056 #elif defined(DEV_AD7606C_16) 00057 #define ACTIVE_DEVICE ID_AD7606C_16 00058 #define ACTIVE_DEVICE_NAME "ad7606c-16" 00059 #elif defined(DEV_AD7606C_18) 00060 #define ACTIVE_DEVICE ID_AD7606C_18 00061 #define ACTIVE_DEVICE_NAME "ad7606c-18" 00062 #elif defined(DEV_AD7608) 00063 #define ACTIVE_DEVICE ID_AD7608 00064 #define ACTIVE_DEVICE_NAME "ad7608" 00065 #elif defined(DEV_AD7609) 00066 #define ACTIVE_DEVICE ID_AD7609 00067 #define ACTIVE_DEVICE_NAME "ad7609" 00068 #else 00069 #warning No/Unsupported ADxxxxy symbol defined. AD7606B defined 00070 #define DEV_AD7606B 00071 #define ACTIVE_DEVICE ID_AD7606B 00072 #define ACTIVE_DEVICE_NAME "ad7606b" 00073 #endif 00074 00075 #if defined(DEV_AD7605_4) 00076 #define AD7606X_ADC_CHANNELS 4 00077 #define AD7606X_ADC_RESOLUTION 16 00078 #elif defined(DEV_AD7606_4) 00079 #define AD7606X_ADC_CHANNELS 4 00080 #define AD7606X_ADC_RESOLUTION 16 00081 #elif defined(DEV_AD7606_6) 00082 #define AD7606X_ADC_CHANNELS 6 00083 #define AD7606X_ADC_RESOLUTION 16 00084 #elif defined(DEV_AD7606_8) 00085 #define AD7606X_ADC_CHANNELS 8 00086 #define AD7606X_ADC_RESOLUTION 16 00087 #elif defined(DEV_AD7606B) 00088 #define AD7606X_ADC_CHANNELS 8 00089 #define AD7606X_ADC_RESOLUTION 16 00090 #elif defined(DEV_AD7606C_16) 00091 #define AD7606X_ADC_CHANNELS 8 00092 #define AD7606X_ADC_RESOLUTION 16 00093 #elif defined(DEV_AD7606C_18) 00094 #define AD7606X_ADC_CHANNELS 8 00095 #define AD7606X_ADC_RESOLUTION 18 00096 #elif defined(DEV_AD7608) 00097 #define AD7606X_ADC_CHANNELS 8 00098 #define AD7606X_ADC_RESOLUTION 18 00099 #elif defined(DEV_AD7609) 00100 #define AD7606X_ADC_CHANNELS 8 00101 #define AD7606X_ADC_RESOLUTION 18 00102 #else 00103 /* Default config for AD7606B */ 00104 #define AD7606X_ADC_CHANNELS 8 00105 #define AD7606X_ADC_RESOLUTION 16 00106 #endif 00107 00108 /* Macros for stringification */ 00109 #define XSTR(s) STR(s) 00110 #define STR(s) #s 00111 00112 /****** Macros used to form a VCOM serial number ******/ 00113 #if !defined(DEVICE_NAME) 00114 #define DEVICE_NAME "DEV_AD760B" 00115 #endif 00116 00117 #if !defined(PLATFORM_NAME) 00118 #define PLATFORM_NAME "SDP_K1" 00119 #endif 00120 /******/ 00121 00122 #if (ACTIVE_PLATFORM == MBED_PLATFORM) 00123 #include "app_config_mbed.h" 00124 00125 /* Used to form a VCOM serial number */ 00126 #define FIRMWARE_NAME "ad7606_mbed_iio_application" 00127 00128 /* Redefine the init params structure mapping w.r.t. platform */ 00129 #define ext_int_extra_init_params mbed_ext_int_extra_init_params 00130 #define uart_extra_init_params mbed_uart_extra_init_params 00131 #define spi_extra_init_params mbed_spi_extra_init_params 00132 #else 00133 #error "No/Invalid active platform selected" 00134 #endif 00135 00136 /* ADC max count (full scale value) for unipolar inputs */ 00137 #define ADC_MAX_COUNT_UNIPOLAR (uint32_t)((1 << AD7606X_ADC_RESOLUTION) - 1) 00138 00139 /* ADC max count (full scale value) for bipolar inputs */ 00140 #define ADC_MAX_COUNT_BIPOLAR (uint32_t)(1 << (AD7606X_ADC_RESOLUTION-1)) 00141 00142 /* Enable the VirtualCOM port connection/interface. By default serial comminunication 00143 * is physical UART */ 00144 //#define USE_VIRTUAL_COM_PORT 00145 00146 #if defined(USE_VIRTUAL_COM_PORT) 00147 /* Below USB configurations (VID and PID) are owned and assigned by ADI. 00148 * If intended to distribute software further, use the VID and PID owned by your 00149 * organization */ 00150 #define VIRTUAL_COM_PORT_VID 0x0456 00151 #define VIRTUAL_COM_PORT_PID 0xb66c 00152 /* Serial number string is formed as: application name + device (target) name + platform (host) name */ 00153 #define VIRTUAL_COM_SERIAL_NUM (FIRMWARE_NAME "_" DEVICE_NAME "_" PLATFORM_NAME) 00154 #endif 00155 00156 /* Baud rate for IIO application UART interface */ 00157 #define IIO_UART_BAUD_RATE (230400) 00158 00159 /******************************************************************************/ 00160 /********************** Public/Extern Declarations ****************************/ 00161 /******************************************************************************/ 00162 00163 extern struct uart_init_param uart_init_params; 00164 extern struct gpio_desc *led_gpio_desc; 00165 00166 int32_t init_system(void); 00167 00168 #endif /* _APP_CONFIG_H_ */
Generated on Wed Jul 13 2022 11:55:04 by
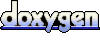