
Example Program for EVAL-AD7606
Dependencies: platform_drivers
app_config.c
00001 /***************************************************************************//** 00002 * @file app_config.c 00003 * @brief Application configurations module (platform-agnostic) 00004 * @details This module performs the system configurations 00005 ******************************************************************************** 00006 * Copyright (c) 2020-2021 Analog Devices, Inc. 00007 * All rights reserved. 00008 * 00009 * This software is proprietary to Analog Devices, Inc. and its licensors. 00010 * By using this software you agree to the terms of the associated 00011 * Analog Devices Software License Agreement. 00012 *******************************************************************************/ 00013 00014 /******************************************************************************/ 00015 /***************************** Include Files **********************************/ 00016 /******************************************************************************/ 00017 00018 #include <stdbool.h> 00019 00020 #include "app_config.h" 00021 #include "adc_data_capture.h" 00022 #include "error.h" 00023 #include "uart.h" 00024 #include "gpio.h" 00025 #include "irq.h" 00026 #include "pwm.h" 00027 00028 /******************************************************************************/ 00029 /************************ Macros/Constants ************************************/ 00030 /******************************************************************************/ 00031 00032 /******************************************************************************/ 00033 /******************** Variables and User Defined Data Types *******************/ 00034 /******************************************************************************/ 00035 00036 /* UART init parameters */ 00037 struct uart_init_param uart_init_params = { 00038 .device_id = NULL, 00039 .baud_rate = IIO_UART_BAUD_RATE, 00040 .extra = &uart_extra_init_params 00041 }; 00042 00043 /* LED GPO init parameters */ 00044 static struct gpio_init_param led_gpio_init_params = { 00045 .number = LED_GPO, 00046 .extra = NULL 00047 }; 00048 00049 /* External interrupt init parameters */ 00050 static struct irq_init_param ext_int_init_params = { 00051 .irq_ctrl_id = EXTERNAL_INT_ID1, 00052 .extra = &ext_int_extra_init_params 00053 }; 00054 00055 /* External interrupt callback descriptor */ 00056 static struct callback_desc ext_int_callback_desc = { 00057 data_capture_callback, 00058 NULL, 00059 NULL 00060 }; 00061 00062 /* PWM init parameters */ 00063 static struct pwm_init_param pwm_init_params = { 00064 .id = PWM_TRIGGER, // GPIO used for PWM 00065 .period_ns = CONV_TRIGGER_PERIOD_NSEC, // PWM period in nsec 00066 .duty_cycle_ns = CONV_TRIGGER_DUTY_CYCLE_NSEC // PWM duty cycle in nsec 00067 }; 00068 00069 /* LED GPO descriptor */ 00070 gpio_desc *led_gpio_desc; 00071 00072 /* External interrupt descriptor */ 00073 struct irq_ctrl_desc *ext_int_desc; 00074 00075 /* PWM descriptor */ 00076 struct pwm_desc *pwm_desc; 00077 00078 /******************************************************************************/ 00079 /************************** Functions Declarations ****************************/ 00080 /******************************************************************************/ 00081 00082 /******************************************************************************/ 00083 /************************** Functions Definitions *****************************/ 00084 /******************************************************************************/ 00085 00086 /** 00087 * @brief Initialize the GPIOs 00088 * @return SUCCESS in case of success, FAILURE otherwise 00089 * @details This function initialize the GPIOs used by application 00090 */ 00091 static int32_t init_gpio(void) 00092 { 00093 do { 00094 /* Initialize the LED GPO */ 00095 if (gpio_get_optional(&led_gpio_desc, &led_gpio_init_params) != SUCCESS) { 00096 break; 00097 } 00098 00099 if (gpio_direction_output(led_gpio_desc, GPIO_HIGH) != SUCCESS) { 00100 break; 00101 } 00102 00103 return SUCCESS; 00104 } while (0); 00105 00106 return FAILURE; 00107 } 00108 00109 00110 /** 00111 * @brief Initialize the IRQ contoller 00112 * @return SUCCESS in case of success, FAILURE otherwise 00113 * @details This function initialize the interrupts for system peripherals 00114 */ 00115 static int32_t init_interrupt(void) 00116 { 00117 do { 00118 /* Init interrupt controller for external interrupt */ 00119 if (irq_ctrl_init(&ext_int_desc, &ext_int_init_params) != SUCCESS) { 00120 break; 00121 } 00122 00123 /* Register a callback function for external interrupt */ 00124 if (irq_register_callback(ext_int_desc, 00125 EXTERNAL_INT_ID1, 00126 &ext_int_callback_desc) != SUCCESS) { 00127 break; 00128 } 00129 00130 return SUCCESS; 00131 } while (0); 00132 00133 return FAILURE; 00134 } 00135 00136 00137 /** 00138 * @brief Initialize the PWM contoller 00139 * @return SUCCESS in case of success, FAILURE otherwise 00140 */ 00141 static int32_t init_pwm(void) 00142 { 00143 do { 00144 /* Initialize the PWM interface to generate PWM signal 00145 * on conversion trigger event pin */ 00146 if (pwm_init(&pwm_desc, &pwm_init_params) != SUCCESS) { 00147 break; 00148 } 00149 00150 if (pwm_enable(pwm_desc) != SUCCESS) { 00151 break; 00152 } 00153 00154 return SUCCESS; 00155 } while (0); 00156 00157 return FAILURE; 00158 } 00159 00160 00161 /** 00162 * @brief Initialize the system peripherals 00163 * @return SUCCESS in case of success, FAILURE otherwise 00164 */ 00165 int32_t init_system(void) 00166 { 00167 if (init_gpio() != SUCCESS) { 00168 return FAILURE; 00169 } 00170 00171 if (init_interrupt() != SUCCESS) { 00172 return FAILURE; 00173 } 00174 00175 if (init_pwm() != SUCCESS) { 00176 return FAILURE; 00177 } 00178 00179 return SUCCESS; 00180 }
Generated on Wed Jul 13 2022 11:55:04 by
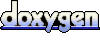