
Example Program for EVAL-AD7606
Dependencies: platform_drivers
ad7606_support.h
00001 /***************************************************************************//** 00002 * @file ad7606_support.h 00003 * @brief Header for AD7606 No-OS driver supports 00004 ******************************************************************************** 00005 * Copyright (c) 2020 Analog Devices, Inc. 00006 * 00007 * All rights reserved. 00008 * 00009 * This software is proprietary to Analog Devices, Inc. and its licensors. 00010 * By using this software you agree to the terms of the associated 00011 * Analog Devices Software License Agreement. 00012 *******************************************************************************/ 00013 00014 #ifndef AD7606_SUPPORT_H_ 00015 #define AD7606_SUPPORT_H_ 00016 00017 /******************************************************************************/ 00018 /***************************** Include Files **********************************/ 00019 /******************************************************************************/ 00020 00021 #include "ad7606.h" 00022 00023 /******************************************************************************/ 00024 /********************** Macros and Constants Definition ***********************/ 00025 /******************************************************************************/ 00026 00027 /* Offset b/w two channel selections in CHx_RANGE register */ 00028 #define CHANNEL_RANGE_MSK_OFFSET 4 00029 00030 /* AD7606_REG_OVERSAMPLING */ 00031 #define AD7606_OVERSAMPLING_MSK GENMASK(3,0) 00032 00033 /* Default channel range for AD7606 devices */ 00034 #define DEFAULT_CHN_RANGE (10.0) 00035 00036 /* Diagnostic channels Mux configurations */ 00037 #define AD7606_DIAGN_MUX_CH_MSK(ch) (GENMASK(2, 0) << (3 * ((ch) % 2))) 00038 #define AD7606_DIAGN_MUX_CH_VAL(ch, val) (val << (3 * ((ch) % 2))) 00039 00040 #define AD7606_OPEN_DETECT_ENABLE_MSK(ch) (GENMASK(7,0) & (~(1 << ch))) 00041 00042 /* Diagnostic channels Mux select bits */ 00043 #define ANALOG_INPUT_MUX 0X00 00044 #define TEMPERATURE_MUX 0x01 00045 #define VREF_MUX 0X02 00046 #define ALDO_MUX 0X03 00047 #define DLDO_MUX 0X04 00048 #define VDRIVE_MUX 0x05 00049 00050 /* Diagnostic Mux multiplers */ 00051 #define VREF_MUX_MULTIPLIER 4.0 00052 00053 /* Unipolar inputs range bits for AD7606C */ 00054 #define AD7606C_UNIPOLAR_RANGE_MIN 5 00055 #define AD7606C_UNIPOLAR_RANGE_MAX 7 00056 00057 /* Number of AD7606 registers */ 00058 #define NUM_OF_REGISTERS 0x2F 00059 00060 /******************************************************************************/ 00061 /********************** Variables and User Defined Data Types *****************/ 00062 /******************************************************************************/ 00063 00064 /* Analog input polarity */ 00065 typedef enum { 00066 UNIPOLAR, 00067 BIPOLAR 00068 } polarity_e; 00069 00070 /******************************************************************************/ 00071 /************************ Public Declarations *********************************/ 00072 /******************************************************************************/ 00073 00074 float convert_adc_raw_to_voltage(int32_t adc_raw, float scale); 00075 int32_t ad7606_read_conversion_data(struct ad7606_dev *dev, uint8_t bytes); 00076 polarity_e ad7606_get_input_polarity(uint8_t chn_range_bits); 00077 00078 #endif /* AD7606_SUPPORT_H_ */
Generated on Wed Jul 13 2022 11:55:04 by
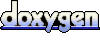