
Example Program for EVAL-AD7606
Dependencies: platform_drivers
ad7606_support.c
00001 /*************************************************************************//** 00002 * @file ad7606_support.c 00003 * @brief AD7606 device No-OS driver supports 00004 ****************************************************************************** 00005 * Copyright (c) 2020 Analog Devices, Inc. 00006 * 00007 * All rights reserved. 00008 * 00009 * This software is proprietary to Analog Devices, Inc. and its licensors. 00010 * By using this software you agree to the terms of the associated 00011 * Analog Devices Software License Agreement. 00012 *****************************************************************************/ 00013 00014 /******************************************************************************/ 00015 /***************************** Include Files **********************************/ 00016 /******************************************************************************/ 00017 00018 #include <stdint.h> 00019 00020 #include "app_config.h" 00021 #include "ad7606_support.h" 00022 #include "ad7606_data_capture.h" 00023 00024 /******************************************************************************/ 00025 /********************** Macros and Constants Definition ***********************/ 00026 /******************************************************************************/ 00027 00028 /******************************************************************************/ 00029 /********************** Variables and User Defined Data Types *****************/ 00030 /******************************************************************************/ 00031 00032 /******************************************************************************/ 00033 /************************ Functions Definitions *******************************/ 00034 /******************************************************************************/ 00035 00036 /*! 00037 * @brief Function to convert adc raw data into equivalent voltage 00038 * @param adc_raw[in] - ADC raw data 00039 * @param scale[in] - ADC raw to voltage conversion scale 00040 * @return equivalent voltage 00041 */ 00042 float convert_adc_raw_to_voltage(int32_t adc_raw, float scale) 00043 { 00044 float voltage; 00045 00046 /* Convert adc data into equivalent voltage. 00047 * scale = (chn_range / MAX_ADC_CNT * 1000) as defined in iio_ad7606.c 00048 * */ 00049 voltage = (adc_raw * (scale / 1000)); 00050 00051 return voltage; 00052 } 00053 00054 00055 /*! 00056 * @brief Function to get the polarity of analog input 00057 * @param chn_range_bits[in] - Bits from the channel range register 00058 * @return UNIPOLAR or BIPOLAR 00059 */ 00060 polarity_e ad7606_get_input_polarity(uint8_t chn_range_bits) 00061 { 00062 polarity_e polarity; 00063 00064 if (chn_range_bits >= AD7606C_UNIPOLAR_RANGE_MIN 00065 && chn_range_bits <= AD7606C_UNIPOLAR_RANGE_MAX) { 00066 polarity = UNIPOLAR; 00067 } else { 00068 polarity = BIPOLAR; 00069 } 00070 00071 return polarity; 00072 } 00073 00074 00075 /*! 00076 * @brief Read the num_of_bytes from previous conversion 00077 * @param dev[in]- Device instance 00078 * @param num_of_bytes[in] - Number of bytes to read from previous conversion 00079 * @return SUCCESS in case of success, FAILURE otherwise 00080 */ 00081 int32_t ad7606_read_conversion_data(struct ad7606_dev *dev, 00082 uint8_t num_of_bytes) 00083 { 00084 memset(dev->data, 0, sizeof(dev->data)); 00085 return spi_write_and_read(dev->spi_desc, dev->data, num_of_bytes); 00086 }
Generated on Wed Jul 13 2022 11:55:04 by
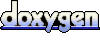