
Example program for AD717x and AD411x family of products.
Dependencies: adi_console_menu platform_drivers
ad717x_support.h
00001 /*! 00002 ***************************************************************************** 00003 @file: ad717x_support.h 00004 00005 @brief: Header for AD717x/AD411x No-OS driver supports 00006 00007 @details: 00008 ----------------------------------------------------------------------------- 00009 Copyright (c) 2020 Analog Devices, Inc. 00010 All rights reserved. 00011 00012 This software is proprietary to Analog Devices, Inc. and its licensors. 00013 By using this software you agree to the terms of the associated 00014 Analog Devices Software License Agreement. 00015 *****************************************************************************/ 00016 00017 #ifndef AD717X_SUPPORT_H_ 00018 #define AD717X_SUPPORT_H_ 00019 00020 /******************************************************************************/ 00021 /***************************** Include Files **********************************/ 00022 /******************************************************************************/ 00023 00024 /******************************************************************************/ 00025 /********************** Macros and Constants Definitions **********************/ 00026 /******************************************************************************/ 00027 00028 /* 00029 * Create a contiguous bitmask starting at bit position @l and ending at 00030 * position @h. 00031 */ 00032 #ifndef GENMASK 00033 #define GENMASK(h, l) (((~0UL) - (1UL << (l)) + 1) & (~0UL >> (31 - (h)))) 00034 #endif 00035 #define BIT(x) (1UL << (x)) 00036 00037 00038 /* ADC Mode Register bits */ 00039 #define AD717X_ADCMODE_REG_MODE_MSK GENMASK(6,4) 00040 #define AD717X_ADCMODE_REG_MODE_RD(x) (((x) >> 4) & 0x7) 00041 00042 00043 /* Channel Map Register bits */ 00044 #define AD717X_CHMAP_REG_CH_EN_RD(x) (((x) >> 15) & 0x1) 00045 00046 #define AD717X_CHMAP_REG_SETUP_SEL_MSK GENMASK(14,12) 00047 #define AD717X_CHMAP_REG_SETUP_SEL_RD(x) (((x) >> 12) & 0x7) 00048 00049 #define AD717X_CHMAP_REG_AINPOS_MSK GENMASK(9,5) 00050 #define AD717X_CHMAP_REG_AINPOS_RD(x) (((x) >> 5) & 0x1F) 00051 00052 #define AD717X_CHMAP_REG_AINNEG_MSK GENMASK(4,0) 00053 #define AD717X_CHMAP_REG_AINNEG_RD(x) (((x) >> 0) & 0x1F) 00054 00055 /* Channel Map Register additional bits for AD4111, AD4112, AD4114, AD4115 */ 00056 #define AD4111_CHMAP_REG_INPUT_MSK GENMASK(9,0) 00057 #define AD4111_CHMAP_REG_INPUT_RD(x) (((x) >> 0) & 0x3FF) 00058 00059 00060 /* Setup Configuration Register bits */ 00061 #define AD717X_SETUP_CONF_REG_BI_UNIPOLAR_RD(x) (((x) >> 12) & 0x1) 00062 00063 #define AD717X_SETUP_CONF_REG_REF_SEL_MSK GENMASK(5,4) 00064 #define AD717X_SETUP_CONF_REG_REF_SEL_RD(x) (((x) >> 4) & 0x3) 00065 00066 /* Setup Configuration Register additional bits for AD7173-8 */ 00067 #define AD717X_SETUP_CONF_REG_REF_BUF_MSK GENMASK(11,10) 00068 #define AD717X_SETUP_CONF_REG_REF_BUF_RD(x) (((x)>> 10) & 0x3) 00069 00070 #define AD717X_SETUP_CONF_REG_AIN_BUF_MSK GENMASK(9,8) 00071 #define AD717X_SETUP_CONF_REG_AIN_BUF_RD(x) (((x) >> 8) & 0x3) 00072 00073 /* Setup Configuration Register additional bits for AD7172-2, AD7172-4, AD7175-2 */ 00074 #define AD717X_SETUP_CONF_REG_REFBUF_P_RD(x) (((x) >> 11) & 0x1) 00075 #define AD717X_SETUP_CONF_REG_REFBUF_N_RD(x) (((x) >> 10) & 0x1) 00076 00077 #define AD717X_SETUP_CONF_REG_AINBUF_P_RD(x) (((x) >> 9) & 0x1) 00078 #define AD717X_SETUP_CONF_REG_AINBUF_N_RD(x) (((x) >> 8) & 0x1) 00079 00080 /* Setup Configuration Register additional bits for AD4111, AD4112, AD4114, AD4115 */ 00081 #define AD4111_SETUP_CONF_REG_REFPOS_BUF_RD(x) (((x) >> 11) & 0x1) 00082 #define AD4111_SETUP_CONF_REG_REFNEG_BUF_RD(x) (((x) >> 10) & 0x1) 00083 00084 #define AD4111_SETUP_CONF_REG_AIN_BUF_MSK GENMASK(9,8) 00085 #define AD4111_SETUP_CONF_REG_AIN_BUF_RD(x) (((x) >> 8) & 0x3) 00086 00087 00088 /* Filter Configuration Register bits */ 00089 #define AD717X_FILT_CONF_REG_ENHFILTEN_RD(x) (((x) >> 11) & 0x1) 00090 00091 #define AD717X_FILT_CONF_REG_ENHFILT_MSK GENMASK(10,8) 00092 #define AD717X_FILT_CONF_REG_ENHFILT_RD(x) (((x) >> 8) & 0x7) 00093 00094 #define AD717X_FILT_CONF_REG_ORDER_MSK GENMASK(6,5) 00095 #define AD717X_FILT_CONF_REG_ORDER_RD(x) (((x) >> 5) & 0x3) 00096 00097 #define AD717X_FILT_CONF_REG_ODR_MSK GENMASK(4,0) 00098 #define AD717X_FILT_CONF_REG_ODR_RD(x) (((x) >> 0) & 0x1F) 00099 00100 00101 // ADC operating mode types 00102 #define CONTINUOUS_CONVERSION 0 00103 #define SINGLE_CONVERISION 1 00104 #define STANDBY_MODE 2 00105 #define POWER_DOWN_MODE 3 00106 #define INTERNAL_OFFSET_CAL_MODE 4 00107 #define INTERNAL_FULL_SCALE_CAL_MODE 5 00108 00109 /******************************************************************************/ 00110 /********************** Variables and User Defined Data Types *****************/ 00111 /******************************************************************************/ 00112 00113 /******************************************************************************/ 00114 /************************ Public Declarations *********************************/ 00115 /******************************************************************************/ 00116 00117 #endif /* AD717X_SUPPORT_H_ */
Generated on Wed Jul 13 2022 14:09:09 by
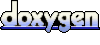