
Example program for AD717x and AD411x family of products.
Dependencies: adi_console_menu platform_drivers
ad717x_menu_defines.h
00001 /*! 00002 ***************************************************************************** 00003 @file: ad717x_menu_defines_app.h 00004 00005 @brief: Header for AD717x/AD411x console menu definitions. 00006 00007 @details: 00008 ----------------------------------------------------------------------------- 00009 Copyright (c) 2020 Analog Devices, Inc. 00010 All rights reserved. 00011 00012 This software is proprietary to Analog Devices, Inc. and its licensors. 00013 By using this software you agree to the terms of the associated 00014 Analog Devices Software License Agreement. 00015 *****************************************************************************/ 00016 00017 #ifndef AD717X_MENU_DEFINES_H_ 00018 #define AD717X_MENU_DEFINES_H_ 00019 00020 /******************************************************************************/ 00021 /***************************** Include Files **********************************/ 00022 /******************************************************************************/ 00023 00024 #include "adi_console_menu.h" 00025 00026 /******************************************************************************/ 00027 /********************** Macros and Constants Definitions **********************/ 00028 /******************************************************************************/ 00029 00030 // Prototypes for the console menu functions defined in ad717x_console_app.c file 00031 void display_main_menu_header(void); 00032 int32_t menu_read_id(uint32_t menu_id); 00033 int32_t menu_read_status(uint32_t menu_id); 00034 int32_t menu_sample_channels(uint32_t menu_id); 00035 int32_t menu_chn_enable_disable_display(uint32_t menu_id); 00036 int32_t menu_input_chn_connect_display(uint32_t menu_id); 00037 int32_t menu_config_and_assign_setup(uint32_t menu_id); 00038 int32_t menu_display_setup(uint32_t menu_id); 00039 int32_t menu_read_temperature(uint32_t menu_id); 00040 int32_t menu_calibrate_adc(uint32_t menu_id); 00041 int32_t menu_read_write_device_regs(uint32_t menu_id); 00042 int32_t menu_channels_enable_disable(uint32_t action); 00043 int32_t menu_analog_input_connect(uint32_t user_analog_input); 00044 int32_t menu_input_type_selection(uint32_t input_type_id); 00045 int32_t menu_select_chn_pair(uint32_t user_channel_pair); 00046 int32_t menu_select_input_pair(uint32_t user_input_pair); 00047 int32_t menu_open_wire_detection(uint32_t menu_id); 00048 int32_t menu_rw_ad717x_register(uint32_t rw_id); 00049 int32_t menu_single_conversion(uint32_t channel_id); 00050 int32_t menu_continuous_conversion_tabular(uint32_t channel_id); 00051 int32_t menu_continuous_conversion_stream(uint32_t channel_id); 00052 int32_t menu_filter_select(uint32_t user_input_filter_type); 00053 int32_t menu_postfiler_enable_disable(uint32_t user_action); 00054 int32_t menu_postfiler_select(uint32_t user_input_post_filter_type); 00055 int32_t menu_odr_select(uint32_t user_input_odr_val); 00056 int32_t menu_polarity_select(uint32_t user_input_polarity); 00057 int32_t menu_reference_source_select(uint32_t user_input_reference); 00058 int32_t menu_ref_buffer_enable_disable(uint32_t user_action); 00059 int32_t menu_input_buffer_enable_disable(uint32_t user_action); 00060 00061 00062 // Define the standard ODR values for the AD717x/AD414x devices 00063 // ODRx value, ODRx string name and ODRx bits 00064 00065 #define ODR_250000 250000.00 00066 #define ODR_250000_STR "250000.00" 00067 #define ODR_250000_BITS 0x00 00068 00069 #define ODR_125000 125000.00 00070 #define ODR_125000_STR "125000.00" 00071 #define ODR_125000_BITS 0x01 00072 00073 #define ODR_62500 62500.00 00074 #define ODR_62500_STR "62500.00" 00075 #define ODR_62500_BITS 0x02 00076 00077 #define ODR_50000 50000.00 00078 #define ODR_50000_STR "50000.00" 00079 #define ODR_50000_BITS 0x03 00080 00081 #define ODR_31250 31250.00 00082 #define ODR_31250_STR "31250.00" 00083 #define ODR_31250_BITS 0x04 00084 00085 #define ODR_25000 25000.00 00086 #define ODR_25000_STR "25000.00" 00087 #define ODR_25000_BITS 0x05 00088 00089 #define ODR_15625 15625.00 00090 #define ODR_15625_STR "15625.00" 00091 #define ODR_15625_BITS 0x06 00092 00093 #define ODR_10417 10417.00 00094 #define ODR_10417_STR "10417.00" 00095 #define ODR_10417_BITS 0x07 00096 00097 #define ODR_10000 10000.00 00098 #define ODR_10000_STR "10000.00" 00099 #define ODR_10000_BITS 0x07 00100 00101 #define ODR_5208 5208.00 00102 #define ODR_5208_STR "5208.00" 00103 #define ODR_5208_BITS 0x08 00104 00105 #define ODR_5000 5000.00 00106 #define ODR_5000_STR "5000.00" 00107 #define ODR_5000_BITS 0x08 00108 00109 #define ODR_3906 3906.00 00110 #define ODR_3906_STR "3906.00" 00111 #define ODR_3906_BITS 0x09 00112 00113 #define ODR_2604 2604.00 00114 #define ODR_2604_STR "2604.00" 00115 #define ODR_2604_BITS 0x09 00116 00117 #define ODR_2597 2597.00 00118 #define ODR_2597_STR "2597.00" 00119 #define ODR_2597_BITS 0x09 00120 00121 #define ODR_2500 2500.00 00122 #define ODR_2500_STR "2500.00" 00123 #define ODR_2500_BITS 0x09 00124 00125 #define ODR_1157 1157.00 00126 #define ODR_1157_STR "1157.00" 00127 #define ODR_1157_BITS 0x0A 00128 00129 #define ODR_1008 1008.00 00130 #define ODR_1008_STR "1008.00" 00131 #define ODR_1008_BITS 0x0A 00132 00133 #define ODR_1007 1007.00 00134 #define ODR_1007_STR "1007.00" 00135 #define ODR_1007_BITS 0x0A 00136 00137 #define ODR_1000 1000.00 00138 #define ODR_1000_STR "1000.00" 00139 #define ODR_1000_BITS 0x0A 00140 00141 #define ODR_539 539.00 00142 #define ODR_539_STR "539.00" 00143 #define ODR_539_BITS 0x0B 00144 00145 #define ODR_504 504.00 00146 #define ODR_504_STR "504.00" 00147 #define ODR_504_BITS 0x0B 00148 00149 #define ODR_503_8 503.80 00150 #define ODR_503_8_STR "503.80" 00151 #define ODR_503_8_BITS 0x0B 00152 00153 #define ODR_500 500.00 00154 #define ODR_500_STR "500.00" 00155 #define ODR_500_BITS 0x0B 00156 00157 #define ODR_401 401.00 00158 #define ODR_401_STR "401.00" 00159 #define ODR_401_BITS 0x0C 00160 00161 #define ODR_400_6 400.60 00162 #define ODR_400_6_STR "400.60" 00163 #define ODR_400_6_BITS 0x0C 00164 00165 #define ODR_400 400.00 00166 #define ODR_400_STR "400.00" 00167 #define ODR_400_BITS 0x0C 00168 00169 #define ODR_397_5 397.50 00170 #define ODR_397_5_STR "397.50" 00171 #define ODR_397_5_BITS 0x0C 00172 00173 #define ODR_397 397.00 00174 #define ODR_397_STR "397.00" 00175 #define ODR_397_BITS 0x0C 00176 00177 #define ODR_381 381.00 00178 #define ODR_381_STR "381.00" 00179 #define ODR_381_BITS 0x0C 00180 00181 #define ODR_206 206.00 00182 #define ODR_206_STR "206.00" 00183 #define ODR_206_BITS 0x0D 00184 00185 #define ODR_200_3 200.3 00186 #define ODR_200_3_STR "200.30" 00187 #define ODR_200_3_BITS 0x0D 00188 00189 #define ODR_200 200.00 00190 #define ODR_200_STR "200.00" 00191 #define ODR_200_BITS 0x0D 00192 00193 #define ODR_102 102.00 00194 #define ODR_102_STR "102.00" 00195 #define ODR_102_BITS 0x0E 00196 00197 #define ODR_100_2 100.20 00198 #define ODR_100_2_STR "100.20" 00199 #define ODR_100_2_BITS 0x0E 00200 00201 #define ODR_100 100.00 00202 #define ODR_100_STR "100.00" 00203 #define ODR_100_BITS 0x0E 00204 00205 #define ODR_60 60.00 00206 #define ODR_60_STR "60.00" 00207 #define ODR_60_BITS 0x0F 00208 00209 #define ODR_59_98 59.98 00210 #define ODR_59_98_STR "59.98" 00211 #define ODR_59_98_BITS 0x0F 00212 00213 #define ODR_59_94 59.94 00214 #define ODR_59_94_STR "59.94" 00215 #define ODR_59_94_BITS 0x0F 00216 00217 #define ODR_59_52 59.52 00218 #define ODR_59_52_STR "59.52" 00219 #define ODR_59_52_BITS 0x0F 00220 00221 #define ODR_50 50.00 00222 #define ODR_50_STR "50.00" 00223 #define ODR_50_BITS 0x10 00224 00225 #define ODR_49_96 49.96 00226 #define ODR_49_96_STR "49.96" 00227 #define ODR_49_96_BITS 0x10 00228 00229 #define ODR_49_68 49.68 00230 #define ODR_49_68_STR "49.68" 00231 #define ODR_49_68_BITS 0x10 00232 00233 #define ODR_20_01 20.01 00234 #define ODR_20_01_STR "20.01" 00235 #define ODR_20_01_BITS 0x11 00236 00237 #define ODR_20 20.00 00238 #define ODR_20_STR "20.00" 00239 #define ODR_20_BITS 0x11 00240 00241 #define ODR_16_63 16.63 00242 #define ODR_16_63_STR "16.63" 00243 #define ODR_16_63_BITS 0x12 00244 00245 #define ODR_16_67 16.67 00246 #define ODR_16_67_STR "16.67" 00247 #define ODR_16_67_BITS 0x12 00248 00249 #define ODR_10 10.00 00250 #define ODR_10_STR "10.00" 00251 #define ODR_10_BITS 0x13 00252 00253 #define ODR_5 5.00 00254 #define ODR_5_STR "5.00" 00255 #define ODR_5_BITS 0x14 00256 00257 #define ODR_2_5 2.50 00258 #define ODR_2_5_STR "2.50" 00259 #define ODR_2_5_BITS 0x15 00260 00261 #define ODR_1_25 1.25 00262 #define ODR_1_25_STR "1.25" 00263 #define ODR_1_25_BITS 0x16 00264 00265 #define ODR_RES_STR "RES" 00266 #define ODR_RES_BITS 0x17 00267 00268 00269 // Analog input bits (0:9) for the AD717x/AD414x devices 00270 #define VIN0_INPUT_BITS 0x00 00271 #define VIN1_INPUT_BITS 0x01 00272 #define VIN2_INPUT_BITS 0x02 00273 #define VIN3_INPUT_BITS 0x03 00274 #define VIN4_INPUT_BITS 0x04 00275 #define VIN5_INPUT_BITS 0x05 00276 #define VIN6_INPUT_BITS 0x06 00277 #define VIN7_INPUT_BITS 0x07 00278 #define VIN8_INPUT_BITS 0x08 00279 #define VIN9_INPUT_BITS 0x09 00280 #define VIN10_INPUT_BITS 0x0A 00281 #define VIN11_INPUT_BITS 0x0B 00282 #define VIN12_INPUT_BITS 0x0C 00283 #define VIN13_INPUT_BITS 0x0D 00284 #define VIN14_INPUT_BITS 0x0E 00285 #define VIN15_INPUT_BITS 0x0F 00286 #define VIN16_INPUT_BITS 0x10 00287 #define VINCOM_INPUT_BITS 0x10 00288 #define IN0N_INPUT_BITS 0x08 00289 #define IN1N_INPUT_BITS 0x09 00290 #define IN2N_INPUT_BITS 0x0A 00291 #define IN3N_INPUT_BITS 0x0B 00292 #define IN3P_INPUT_BITS 0x0C 00293 #define IN2P_INPUT_BITS 0x0D 00294 #define IN1P_INPUT_BITS 0x0E 00295 #define IN0P_INPUT_BITS 0x0F 00296 #define TEMP_SENSOR_POS_INP_BITS 0x11 00297 #define TEMP_SENSOR_NEG_INP_BITS 0x12 00298 #define AVDD1_AVSS_P_BITS 0x13 00299 #define AVDD1_AVSS_N_BITS 0x14 00300 #define REFP_INPUT_BITS 0x15 00301 #define REFN_INPUT_BITS 0x16 00302 00303 // Offset to form VIN+ and VIN- pairs 00304 #define VIN_PAIR_OFFSET 5 00305 00306 00307 // Channels define for the AD717x/AD414x devices 00308 #define ADC_CHN(x) (x) 00309 00310 // Offset to form Channel pairs 00311 #define CHN_PAIR_OFFSET 4 00312 #define CHN_PAIR_MASK 0x0F 00313 00314 00315 // Device register read/write identifiers 00316 #define DEVICE_REG_READ_ID (uint32_t)1 00317 #define DEVICE_REG_WRITE_ID (uint32_t)2 00318 00319 // Enable/Disable selection identifiers 00320 #define SELECT_DISBLE (uint32_t)0 00321 #define SELECT_ENABLE (uint32_t)1 00322 00323 // Input Type selection identifiers 00324 #define SINGLE_ENDED_INPUT (uint32_t)0 00325 #define DIFF_ENDED_INPUT (uint32_t)1 00326 00327 // Analog input select identifiers 00328 #define ANALOG_INP_PAIR_SELECT (uint32_t)0 00329 #define POS_ANALOG_INP_SELECT (uint32_t)1 00330 #define NEG_ANALOG_INP_SELECT (uint32_t)2 00331 00332 00333 // Digital filter type (ORDER0) bits 00334 #define SINC5_SINC1_FILTER (uint32_t)0 00335 #define SINC3_FILTER (uint32_t)1 00336 00337 // SINC5+SINC1 post filter type bits 00338 #define POST_FILTER_NA (uint32_t)0 00339 #define POST_FLTR_27_SPS (uint32_t)2 00340 #define POST_FLTR_25_SPS (uint32_t)3 00341 #define POST_FLTR_20_SPS (uint32_t)5 00342 #define POST_FLTR_16_67_SPS (uint32_t)6 00343 00344 // Reference source type bits 00345 #define EXTERNAL (uint32_t)0 00346 #define INTERNAL (uint32_t)2 00347 #define AVDD_AVSS (uint32_t)3 00348 00349 // Polarity selection bits 00350 #define UNIPOLAR (uint32_t)0 00351 #define BIPOLAR (uint32_t)1 00352 00353 /******************************************************************************/ 00354 /********************** Variables and User Defined Data Types *****************/ 00355 /******************************************************************************/ 00356 00357 // Enable/Disable status names 00358 const char *enable_disable_status[] = { 00359 "Disable", 00360 "Enable" 00361 }; 00362 00363 // Polarity names 00364 const char *polarity_status[] = { 00365 "Unipolar", 00366 "Bipolar" 00367 }; 00368 00369 // Filter names 00370 const char *filter_name[] = { 00371 "Sinc5+1", 00372 "Sinc3", 00373 }; 00374 00375 // Post filter names 00376 const char *postfilter_name[] = { 00377 "NA", 00378 "NA", 00379 "27_SPS", 00380 "25_SPS", 00381 "NA", 00382 "20_SPS", 00383 "16_SPS", 00384 }; 00385 00386 // Reference source names 00387 const char *reference_name[] = { 00388 "External", 00389 "External", 00390 "Internal", 00391 "AVDD-AVSS" 00392 }; 00393 00394 00395 // Analog Input pin map 00396 const char *input_pin_map[] = { 00397 #if defined(DEV_AD4111) || defined(DEV_AD4112) 00398 "VIN0", "VIN1", "VIN2", "VIN3", "VIN4", "VIN5", 00399 "VIN6", "VIN7", "IN0-", "IN1-", "IN2-", "IN3-", 00400 "IN3+", "IN2+", "IN1+", "IN0+", "VINCOM", 00401 "TEMP+", "TEMP-", "RES", "RES", "REF+", "REF-" 00402 #elif defined(DEV_AD4114) || defined(DEV_AD4115) 00403 "VIN0", "VIN1", "VIN2", "VIN3", "VIN4", "VIN5", 00404 "VIN6", "VIN7", "VIN8", "VIN9", "VIN10", "VIN11", 00405 "VIN12", "VIN13", "VIN14", "VIN15", "VINCOM", 00406 "TEMP+", "TEMP-", "RES", "RES", "REF+", "REF-" 00407 #elif defined(DEV_AD7173_8) || defined(DEV_AD7175_8) 00408 "AIN0", "AIN1", "AIN2", "AIN3", "AIN4", "AIN5", 00409 "AIN6", "AIN7", "AIN8", "AIN9", "AIN10", "AIN11", 00410 "AIN12", "AIN13", "AIN14", "AIN15", "AIN16", 00411 "TEMP+", "TEMP-", 00412 #if defined(DEV_AD7173_8) 00413 "RES", "RES", 00414 #else 00415 "((AVDD1 ? AVSS)/5)+", "((AVDD1 ? AVSS)/5)-", 00416 #endif 00417 "REF+", "REF-" 00418 #elif defined(DEV_AD7172_2) || defined(DEV_AD7177_2) || defined(DEV_AD7175_2) 00419 "AIN0", "AIN1", "AIN2", "AIN3", "AIN4", 00420 "RES", "RES", "RES", "RES", "RES", "RES", 00421 "RES", "RES", "RES", "RES", "RES", "RES", 00422 "TEMP+", "TEMP-", "((AVDD1 ? AVSS)/5)+", "((AVDD1 ? AVSS)/5)-", 00423 "REF+", "REF-" 00424 #elif defined(DEV_AD7172_4) 00425 "AIN0", "AIN1", "AIN2", "AIN3", "AIN4", 00426 "AIN5", "AIN6", "AIN7", "AIN8", 00427 "RES", "RES", "RES", "RES", "RES", "RES", 00428 "TEMP+", "TEMP-", "((AVDD1 ? AVSS)/5)+", "((AVDD1 ? AVSS)/5)-", 00429 "REF+", "REF-" 00430 #elif defined(DEV_AD7176_2) 00431 "AIN0", "AIN1", "AIN2", "AIN3", "AIN4", 00432 "RES", "RES", "RES", "RES", "RES", "RES", 00433 "RES", "RES", "RES", "RES", "RES", "RES", 00434 "RES", "RES", "RES", "RES", "REF+", "REF-" 00435 #endif 00436 }; 00437 00438 00439 // SIN5+SINC1 Filter ODR map 00440 const float sinc5_sinc1_odr_map[] = { 00441 #if defined(DEV_AD4115) 00442 ODR_125000, ODR_125000, ODR_62500, ODR_62500, 00443 ODR_31250, ODR_25000, ODR_15625, ODR_10417, ODR_5000, 00444 ODR_2500, ODR_1000, ODR_500, ODR_397_5, ODR_200, ODR_100, 00445 ODR_59_98, ODR_49_96, ODR_20, ODR_16_67, 00446 ODR_10, ODR_5, ODR_2_5, ODR_2_5 00447 #elif defined(DEV_AD4111) || defined(DEV_AD4112) || defined(DEV_AD4114) || \ 00448 defined(DEV_AD7172_2) || defined(DEV_AD7172_4) || defined(DEV_AD7173_8) 00449 ODR_31250, ODR_31250, ODR_31250, ODR_31250, ODR_31250, ODR_31250, 00450 ODR_15625, ODR_10417, ODR_5208, ODR_2597, ODR_1007, ODR_503_8, 00451 ODR_381, ODR_200_3, ODR_100_2, ODR_59_52, ODR_49_68, ODR_20_01, 00452 ODR_16_63, ODR_10, ODR_5, ODR_2_5, ODR_1_25, 00453 #elif defined(DEV_AD7176_2) || defined(DEV_AD7175_2) || defined(DEV_AD7175_8) ||\ 00454 defined(DEV_AD7177_2) 00455 #if (DEV_AD7176_2) || defined(DEV_AD7175_2) || defined(DEV_AD7175_8) 00456 ODR_250000, ODR_125000, ODR_62500, ODR_50000, ODR_31250, ODR_25000, ODR_15625, 00457 #elif defined(DEV_AD7177_2) 00458 ODR_0, ODR_0, ODR_0, ODR_0, ODR_0, ODR_0, ODR_0, 00459 #endif 00460 ODR_10000, ODR_5000, ODR_2500, ODR_1000, ODR_500, ODR_397_5, ODR_200, 00461 ODR_100, ODR_59_94, ODR_49_96, ODR_20, ODR_16_67, ODR_10, ODR_5, 00462 ODR_0, ODR_0, 00463 #endif 00464 }; 00465 00466 const float sinc3_odr_map[] = { 00467 #if defined(DEV_AD4115) 00468 ODR_125000, ODR_125000, ODR_62500, ODR_62500, 00469 ODR_31250, ODR_25000, ODR_15625, ODR_10417, ODR_5000, 00470 ODR_3906, ODR_1157, ODR_539, ODR_401, ODR_206, 00471 ODR_102, ODR_59_98, ODR_50, ODR_20, ODR_16_67, 00472 ODR_10, ODR_5, ODR_2_5, ODR_2_5 00473 #elif defined(DEV_AD4111) || defined(DEV_AD4112) || defined(DEV_AD4114) || \ 00474 defined(DEV_AD7172_2) || defined(DEV_AD7172_4) || defined(DEV_AD7173_8) 00475 ODR_31250, ODR_31250, ODR_31250, 00476 ODR_31250, ODR_31250, ODR_31250, 00477 ODR_15625, ODR_10417, ODR_5208, 00478 #if defined(DEV_AD4111) || defined(DEV_AD4112) || defined(DEV_AD4114) 00479 ODR_3906, ODR_1157, ODR_539, 00480 ODR_401, ODR_206, ODR_102, 00481 #else 00482 ODR_2604, ODR_1008, ODR_504, 00483 ODR_400_6, ODR_200_3, ODR_100_2, 00484 #endif 00485 ODR_59_98, ODR_50, ODR_20_01, 00486 ODR_16_67, ODR_10, ODR_5, 00487 ODR_2_5, ODR_1_25, 00488 #elif defined(DEV_AD7176_2) || defined(DEV_AD7175_2) || defined(DEV_AD7175_8) ||\ 00489 defined(DEV_AD7177_2) 00490 #if (DEV_AD7176_2) || defined(DEV_AD7175_2) || defined(DEV_AD7175_8) 00491 ODR_250000, ODR_125000, ODR_62500, 00492 ODR_50000, ODR_31250, ODR_25000, 00493 ODR_15625, 00494 #elif defined(DEV_AD7177_2) 00495 ODR_0, ODR_0, ODR_0, ODR_0, 00496 ODR_0, ODR_0, ODR_0, 00497 #endif 00498 ODR_10000, ODR_5000, ODR_2500, ODR_1000, ODR_500, ODR_400, ODR_200, 00499 ODR_100, ODR_60, ODR_50, ODR_20, ODR_16_67, ODR_10, ODR_5, 00500 ODR_0, ODR_0, 00501 #endif 00502 }; 00503 00504 00505 /* 00506 * Definition of the channel enable/disable menu items and menu itself 00507 */ 00508 console_menu_item chn_enable_disable_items[] = { 00509 { "Enable Channels", 'E', menu_channels_enable_disable, SELECT_ENABLE }, 00510 { "Disable Channels", 'D', menu_channels_enable_disable, SELECT_DISBLE }, 00511 }; 00512 00513 console_menu chn_enable_disable_menu = { 00514 .title = "Channel Enable/Disable Menu", 00515 .items = chn_enable_disable_items, 00516 .itemCount = ARRAY_SIZE(chn_enable_disable_items), 00517 .headerItem = NULL, 00518 .footerItem = NULL, 00519 .enableEscapeKey = true 00520 }; 00521 00522 00523 /* 00524 * Definition of the analog input connection menu items and menu itself 00525 */ 00526 console_menu_item analog_input_connect_items[] = { 00527 #if defined(DEV_AD4111) || defined(DEV_AD4112) || defined(DEV_AD4114) || defined(DEV_AD4115) 00528 // Input pin name/pair Key Menu Function AINP/AINM Bits 00529 // 00530 { "VIN0, VIN1", 'A', menu_analog_input_connect, ((VIN0_INPUT_BITS << VIN_PAIR_OFFSET) | VIN1_INPUT_BITS) }, 00531 { "VIN0, VINCOM", 'B', menu_analog_input_connect, ((VIN0_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00532 { "VIN1, VIN0", 'C', menu_analog_input_connect, ((VIN1_INPUT_BITS << VIN_PAIR_OFFSET) | VIN0_INPUT_BITS) }, 00533 { "VIN1, VINCOM", 'D', menu_analog_input_connect, ((VIN1_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00534 { "VIN2, VIN3", 'E', menu_analog_input_connect, ((VIN2_INPUT_BITS << VIN_PAIR_OFFSET) | VIN3_INPUT_BITS) }, 00535 { "VIN2, VINCOM", 'F', menu_analog_input_connect, ((VIN2_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00536 { "VIN3, VIN2", 'G', menu_analog_input_connect, ((VIN3_INPUT_BITS << VIN_PAIR_OFFSET) | VIN2_INPUT_BITS) }, 00537 { "VIN3, VINCOM", 'H', menu_analog_input_connect, ((VIN3_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00538 { "VIN4, VIN5", 'I', menu_analog_input_connect, ((VIN4_INPUT_BITS << VIN_PAIR_OFFSET) | VIN5_INPUT_BITS) }, 00539 { "VIN4, VINCOM", 'J', menu_analog_input_connect, ((VIN4_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00540 { "VIN5, VIN4", 'K', menu_analog_input_connect, ((VIN5_INPUT_BITS << VIN_PAIR_OFFSET) | VIN4_INPUT_BITS) }, 00541 { "VIN5, VINCOM", 'L', menu_analog_input_connect, ((VIN5_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00542 { "VIN6, VIN7", 'M', menu_analog_input_connect, ((VIN6_INPUT_BITS << VIN_PAIR_OFFSET) | VIN7_INPUT_BITS) }, 00543 { "VIN6, VINCOM", 'N', menu_analog_input_connect, ((VIN6_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00544 { "VIN7, VIN6", 'O', menu_analog_input_connect, ((VIN7_INPUT_BITS << VIN_PAIR_OFFSET) | VIN6_INPUT_BITS) }, 00545 { "VIN7, VINCOM", 'P', menu_analog_input_connect, ((VIN7_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00546 #if defined(DEV_AD4111) || defined(DEV_AD4112) 00547 { "IN3+, IN3-", 'Q', menu_analog_input_connect, ((IN3P_INPUT_BITS << VIN_PAIR_OFFSET) | IN3N_INPUT_BITS) }, 00548 { "IN2+, IN2-", 'R', menu_analog_input_connect, ((IN2P_INPUT_BITS << VIN_PAIR_OFFSET) | IN2N_INPUT_BITS) }, 00549 { "IN1+, IN1-", 'S', menu_analog_input_connect, ((IN1P_INPUT_BITS << VIN_PAIR_OFFSET) | IN1N_INPUT_BITS) }, 00550 { "IN0+, IN0-", 'T', menu_analog_input_connect, ((IN0P_INPUT_BITS << VIN_PAIR_OFFSET) | IN0N_INPUT_BITS) }, 00551 #else // mapping for AD4114/15 00552 { "VIN8, VIN9", 'Q', menu_analog_input_connect, ((VIN8_INPUT_BITS << VIN_PAIR_OFFSET) | VIN9_INPUT_BITS) }, 00553 { "VIN8, VINCOM", 'R', menu_analog_input_connect, ((VIN8_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00554 { "VIN9, VIN8", 'S', menu_analog_input_connect, ((VIN9_INPUT_BITS << VIN_PAIR_OFFSET) | VIN8_INPUT_BITS) }, 00555 { "VIN9, VINCOM", 'T', menu_analog_input_connect, ((VIN9_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00556 { "VIN10, VIN11", 'U', menu_analog_input_connect, ((VIN10_INPUT_BITS << VIN_PAIR_OFFSET) | VIN11_INPUT_BITS) }, 00557 { "VIN10, VINCOM", 'V', menu_analog_input_connect, ((VIN10_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00558 { "VIN11, VIN10", 'W', menu_analog_input_connect, ((VIN11_INPUT_BITS << VIN_PAIR_OFFSET) | VIN10_INPUT_BITS) }, 00559 { "VIN11, VINCOM", 'X', menu_analog_input_connect, ((VIN11_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00560 { "VIN12, VIN13", 'Y', menu_analog_input_connect, ((VIN12_INPUT_BITS << VIN_PAIR_OFFSET) | VIN13_INPUT_BITS) }, 00561 { "VIN12, VINCOM", 'Z', menu_analog_input_connect, ((VIN12_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00562 { "VIN13, VIN12", '1', menu_analog_input_connect, ((VIN13_INPUT_BITS << VIN_PAIR_OFFSET) | VIN12_INPUT_BITS) }, 00563 { "VIN13, VINCOM", '2', menu_analog_input_connect, ((VIN13_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00564 { "VIN14, VIN15", '3', menu_analog_input_connect, ((VIN14_INPUT_BITS << VIN_PAIR_OFFSET) | VIN15_INPUT_BITS) }, 00565 { "VIN14, VINCOM", '4', menu_analog_input_connect, ((VIN14_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00566 { "VIN15, VIN14", '5', menu_analog_input_connect, ((VIN15_INPUT_BITS << VIN_PAIR_OFFSET) | VIN14_INPUT_BITS) }, 00567 { "VIN15, VINCOM", '6', menu_analog_input_connect, ((VIN15_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00568 #endif 00569 { "Temperature Sensor", '7', menu_analog_input_connect, ((TEMP_SENSOR_POS_INP_BITS << VIN_PAIR_OFFSET) | TEMP_SENSOR_NEG_INP_BITS) }, 00570 { "Reference", '8', menu_analog_input_connect, ((REFP_INPUT_BITS << VIN_PAIR_OFFSET) | REFN_INPUT_BITS) }, 00571 #else 00572 { "AIN0", 'A', menu_analog_input_connect, VIN0_INPUT_BITS }, 00573 { "AIN1", 'B', menu_analog_input_connect, VIN1_INPUT_BITS }, 00574 { "AIN2", 'C', menu_analog_input_connect, VIN2_INPUT_BITS }, 00575 { "AIN3", 'D', menu_analog_input_connect, VIN3_INPUT_BITS }, 00576 { "AIN4", 'E', menu_analog_input_connect, VIN4_INPUT_BITS }, 00577 #if defined(DEV_AD7172_2) || defined(DEV_AD7177_2) || defined(DEV_AD7175_2) 00578 { "Temperature Sensor+", 'F', menu_analog_input_connect, TEMP_SENSOR_POS_INP_BITS }, 00579 { "Temperature Sensor-", 'G', menu_analog_input_connect, TEMP_SENSOR_NEG_INP_BITS }, 00580 { "((AVDD1 - AVSS)/5)+ ", 'H', menu_analog_input_connect, AVDD1_AVSS_P_BITS }, 00581 { "((AVDD1 - AVSS)/5)-", 'I', menu_analog_input_connect, AVDD1_AVSS_N_BITS }, 00582 { "REF+", 'J', menu_analog_input_connect, REFP_INPUT_BITS }, 00583 { "REF-", 'K', menu_analog_input_connect, REFN_INPUT_BITS }, 00584 #elif defined(DEV_AD7172_4) 00585 { "AIN5", 'F', menu_analog_input_connect, VIN5_INPUT_BITS }, 00586 { "AIN6", 'G', menu_analog_input_connect, VIN6_INPUT_BITS }, 00587 { "AIN7", 'H', menu_analog_input_connect, VIN7_INPUT_BITS }, 00588 { "AIN8", 'I', menu_analog_input_connect, VIN8_INPUT_BITS }, 00589 { "((AVDD1 - AVSS)/5)+ ", 'J', menu_analog_input_connect, AVDD1_AVSS_P_BITS }, 00590 { "((AVDD1 - AVSS)/5)-", 'K', menu_analog_input_connect, AVDD1_AVSS_N_BITS }, 00591 { "REF+", 'L', menu_analog_input_connect, REFP_INPUT_BITS }, 00592 { "REF-", 'M', menu_analog_input_connect, REFN_INPUT_BITS }, 00593 #elif defined(DEV_AD7176_2) 00594 { "REF+", 'F', menu_analog_input_connect, REFP_INPUT_BITS }, 00595 { "REF-", 'G', menu_analog_input_connect, REFN_INPUT_BITS }, 00596 #elif defined(DEV_AD7173_8) || defined(DEV_AD7175_8) 00597 { "AIN5", 'F', menu_analog_input_connect, VIN5_INPUT_BITS }, 00598 { "AIN6", 'G', menu_analog_input_connect, VIN6_INPUT_BITS }, 00599 { "AIN7", 'H', menu_analog_input_connect, VIN7_INPUT_BITS }, 00600 { "AIN8", 'I', menu_analog_input_connect, VIN8_INPUT_BITS }, 00601 { "AIN9", 'J', menu_analog_input_connect, VIN9_INPUT_BITS }, 00602 { "AIN10", 'K', menu_analog_input_connect, VIN10_INPUT_BITS }, 00603 { "AIN11", 'L', menu_analog_input_connect, VIN11_INPUT_BITS }, 00604 { "AIN12", 'M', menu_analog_input_connect, VIN12_INPUT_BITS }, 00605 { "AIN13", 'N', menu_analog_input_connect, VIN13_INPUT_BITS }, 00606 { "AIN14", 'O', menu_analog_input_connect, VIN14_INPUT_BITS }, 00607 { "AIN15", 'P', menu_analog_input_connect, VIN15_INPUT_BITS }, 00608 { "AIN16", 'Q', menu_analog_input_connect, VIN16_INPUT_BITS }, 00609 { "Temperature Sensor+", 'R', menu_analog_input_connect, TEMP_SENSOR_POS_INP_BITS }, 00610 { "Temperature Sensor-", 'S', menu_analog_input_connect, TEMP_SENSOR_NEG_INP_BITS }, 00611 #if (DEV_AD7175_8) 00612 { "((AVDD1 - AVSS)/5)+ ", 'T', menu_analog_input_connect, AVDD1_AVSS_P_BITS }, 00613 { "((AVDD1 - AVSS)/5)-", 'U', menu_analog_input_connect, AVDD1_AVSS_N_BITS }, 00614 #endif 00615 { "REF+", 'V', menu_analog_input_connect, REFP_INPUT_BITS }, 00616 { "REF-", 'W', menu_analog_input_connect, REFN_INPUT_BITS }, 00617 #endif 00618 #endif 00619 }; 00620 00621 console_menu analog_input_connect_menu = { 00622 .title = "Select Analog Input", 00623 .items = analog_input_connect_items, 00624 .itemCount = ARRAY_SIZE(analog_input_connect_items), 00625 .headerItem = NULL, 00626 .footerItem = NULL, 00627 .enableEscapeKey = true 00628 }; 00629 00630 00631 /* 00632 * Definition of the open wire detect inputs type menu items and menu itself 00633 */ 00634 console_menu_item open_wire_detect_input_type_items[] = { 00635 { "Single Ended Input", 'S', menu_input_type_selection, SINGLE_ENDED_INPUT }, 00636 { "Differential Ended Input", 'D', menu_input_type_selection, DIFF_ENDED_INPUT }, 00637 }; 00638 00639 console_menu open_wire_detect_input_type_menu = { 00640 .title = "Select Analog Input Type", 00641 .items = open_wire_detect_input_type_items, 00642 .itemCount = ARRAY_SIZE(open_wire_detect_input_type_items), 00643 .headerItem = NULL, 00644 .footerItem = NULL, 00645 .enableEscapeKey = true 00646 }; 00647 00648 00649 /* 00650 * Definition of the open wire detect single ended input channel pair menu items and menu itself 00651 */ 00652 console_menu_item open_wire_detect_se_channel_items[] = { 00653 { "CHN0, CHN15", 'A', menu_select_chn_pair, ((ADC_CHN(0) << CHN_PAIR_OFFSET) | ADC_CHN(15)) }, 00654 { "CHN1, CHN2", 'B', menu_select_chn_pair, ((ADC_CHN(1) << CHN_PAIR_OFFSET) | ADC_CHN(2)) }, 00655 { "CHN3, CHN4", 'C', menu_select_chn_pair, ((ADC_CHN(3) << CHN_PAIR_OFFSET) | ADC_CHN(4)) }, 00656 { "CHN5, CHN6", 'D', menu_select_chn_pair, ((ADC_CHN(5) << CHN_PAIR_OFFSET) | ADC_CHN(6)) }, 00657 { "CHN7, CHN8", 'E', menu_select_chn_pair, ((ADC_CHN(7) << CHN_PAIR_OFFSET) | ADC_CHN(8)) }, 00658 { "CHN9, CHN10", 'F', menu_select_chn_pair, ((ADC_CHN(9) << CHN_PAIR_OFFSET) | ADC_CHN(10)) }, 00659 { "CHN11, CHN12", 'G', menu_select_chn_pair, ((ADC_CHN(11) << CHN_PAIR_OFFSET) | ADC_CHN(12)) }, 00660 { "CHN13, CHN14", 'H', menu_select_chn_pair, ((ADC_CHN(13) << CHN_PAIR_OFFSET) | ADC_CHN(14)) }, 00661 }; 00662 00663 console_menu open_wire_detect_se_channel_menu = { 00664 .title = "Select Channel Pair", 00665 .items = open_wire_detect_se_channel_items, 00666 .itemCount = ARRAY_SIZE(open_wire_detect_se_channel_items), 00667 .headerItem = NULL, 00668 .footerItem = NULL, 00669 .enableEscapeKey = true 00670 }; 00671 00672 00673 /* 00674 * Definition of the open wire detect differential ended input channel pair menu items and menu itself 00675 */ 00676 console_menu_item open_wire_detect_de_channel_items[] = { 00677 { "CHN1, CHN2", 'A', menu_select_chn_pair, ((ADC_CHN(1) << CHN_PAIR_OFFSET) | ADC_CHN(2)) }, 00678 { "CHN5, CHN6", 'B', menu_select_chn_pair, ((ADC_CHN(5) << CHN_PAIR_OFFSET) | ADC_CHN(6)) }, 00679 { "CHN9, CHN10", 'C', menu_select_chn_pair, ((ADC_CHN(9) << CHN_PAIR_OFFSET) | ADC_CHN(10)) }, 00680 { "CHN13, CHN14", 'D', menu_select_chn_pair, ((ADC_CHN(13) << CHN_PAIR_OFFSET) | ADC_CHN(14)) }, 00681 }; 00682 00683 console_menu open_wire_detect_de_channel_menu = { 00684 .title = "Select Channel Pair", 00685 .items = open_wire_detect_de_channel_items, 00686 .itemCount = ARRAY_SIZE(open_wire_detect_de_channel_items), 00687 .headerItem = NULL, 00688 .footerItem = NULL, 00689 .enableEscapeKey = true 00690 }; 00691 00692 00693 /* 00694 * Definition of the open wire detect single ended analog inputs menu items and menu itself 00695 */ 00696 console_menu_item open_wire_detect_se_analog_input_items[] = { 00697 { "VIN0, VINCOM", 'A', menu_select_input_pair, ((VIN0_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00698 { "VIN1, VINCOM", 'B', menu_select_input_pair, ((VIN1_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00699 { "VIN2, VINCOM", 'C', menu_select_input_pair, ((VIN2_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00700 { "VIN3, VINCOM", 'D', menu_select_input_pair, ((VIN3_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00701 { "VIN4, VINCOM", 'E', menu_select_input_pair, ((VIN4_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00702 { "VIN5, VINCOM", 'F', menu_select_input_pair, ((VIN5_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00703 { "VIN6, VINCOM", 'G', menu_select_input_pair, ((VIN6_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00704 { "VIN7, VINCOM", 'H', menu_select_input_pair, ((VIN7_INPUT_BITS << VIN_PAIR_OFFSET) | VINCOM_INPUT_BITS) }, 00705 }; 00706 00707 console_menu open_wire_detect_se_analog_input_menu = { 00708 .title = "Select Analog Inputs", 00709 .items = open_wire_detect_se_analog_input_items, 00710 .itemCount = ARRAY_SIZE(open_wire_detect_se_analog_input_items), 00711 .headerItem = NULL, 00712 .footerItem = NULL, 00713 .enableEscapeKey = true 00714 }; 00715 00716 00717 /* 00718 * Definition of the open wire detect differential ended analog inputs menu items and menu itself 00719 */ 00720 console_menu_item open_wire_detect_de_analog_input_items[] = { 00721 { "VIN0, VIN1", 'A', menu_select_input_pair, ((VIN0_INPUT_BITS << VIN_PAIR_OFFSET) | VIN1_INPUT_BITS) }, 00722 { "VIN2, VIN3", 'B', menu_select_input_pair, ((VIN2_INPUT_BITS << VIN_PAIR_OFFSET) | VIN3_INPUT_BITS) }, 00723 { "VIN4, VIN5", 'C', menu_select_input_pair, ((VIN4_INPUT_BITS << VIN_PAIR_OFFSET) | VIN5_INPUT_BITS) }, 00724 { "VIN6, VIN7", 'D', menu_select_input_pair, ((VIN6_INPUT_BITS << VIN_PAIR_OFFSET) | VIN7_INPUT_BITS) }, 00725 }; 00726 00727 console_menu open_wire_detect_de_analog_input_menu = { 00728 .title = "Select Analog Inputs", 00729 .items = open_wire_detect_de_analog_input_items, 00730 .itemCount = ARRAY_SIZE(open_wire_detect_de_analog_input_items), 00731 .headerItem = NULL, 00732 .footerItem = NULL, 00733 .enableEscapeKey = true 00734 }; 00735 00736 00737 /* 00738 * Definition of the adc register read/write menu items and menu itself 00739 */ 00740 console_menu_item reg_read_write_items[] = { 00741 { "Read Device Register", 'R', menu_rw_ad717x_register, DEVICE_REG_READ_ID }, 00742 { "Write Device Register", 'W', menu_rw_ad717x_register, DEVICE_REG_WRITE_ID }, 00743 }; 00744 00745 console_menu reg_read_write_menu = { 00746 .title = "Register Read/Write Menu", 00747 .items = reg_read_write_items, 00748 .itemCount = ARRAY_SIZE(reg_read_write_items), 00749 .headerItem = NULL, 00750 .footerItem = NULL, 00751 .enableEscapeKey = true 00752 }; 00753 00754 00755 /* 00756 * Definition of the sampling menu items and menu itself 00757 */ 00758 console_menu_item acquisition_menu_items[] = { 00759 { "Single Conversion Mode", 'S', menu_single_conversion }, 00760 { "Continuous Conversion Mode - Table View", 'T', menu_continuous_conversion_tabular }, 00761 { "Continuous Conversion Mode - Stream Data", 'C', menu_continuous_conversion_stream }, 00762 }; 00763 00764 console_menu acquisition_menu = { 00765 .title = "Data Acquisition Menu", 00766 .items = acquisition_menu_items, 00767 .itemCount = ARRAY_SIZE(acquisition_menu_items), 00768 .headerItem = NULL, 00769 .footerItem = NULL, 00770 .enableEscapeKey = true 00771 }; 00772 00773 00774 /* 00775 * Definition of the filter select menu items and menu itself 00776 */ 00777 console_menu_item filter_select_items[] = { 00778 { "Sinc5+1", 'A', menu_filter_select, SINC5_SINC1_FILTER }, 00779 { "Sinc3", 'B', menu_filter_select, SINC3_FILTER }, 00780 }; 00781 00782 console_menu filter_select_menu = { 00783 .title = "Filter Selection Menu", 00784 .items = filter_select_items, 00785 .itemCount = ARRAY_SIZE(filter_select_items), 00786 .headerItem = NULL, 00787 .footerItem = NULL, 00788 .enableEscapeKey = true 00789 }; 00790 00791 00792 /* 00793 * Definition of the postfilter enable/disable menu items and menu itself 00794 */ 00795 console_menu_item postfilter_enable_disable_items[] = { 00796 { "Enable", 'E', menu_postfiler_enable_disable, SELECT_ENABLE }, 00797 { "Disable", 'D', menu_postfiler_enable_disable, SELECT_DISBLE }, 00798 }; 00799 00800 console_menu postfilter_enable_disable_menu = { 00801 .title = "Post-filter Enable/Disable Menu", 00802 .items = postfilter_enable_disable_items, 00803 .itemCount = ARRAY_SIZE(postfilter_enable_disable_items), 00804 .headerItem = NULL, 00805 .footerItem = NULL, 00806 .enableEscapeKey = true 00807 }; 00808 00809 00810 /* 00811 * Definition of the post-filter select menu items and menu itself 00812 */ 00813 console_menu_item postfilter_select_items[] = { 00814 { "27 SPS, 47 dB reject, 36.7 ms settling ",'A', menu_postfiler_select, POST_FLTR_27_SPS }, 00815 { "25 SPS, 62 dB reject, 40 ms settling", 'B', menu_postfiler_select, POST_FLTR_25_SPS }, 00816 { "20 SPS, 86 dB reject, 50 ms settling", 'C', menu_postfiler_select, POST_FLTR_20_SPS }, 00817 { "16.67 SPS, 92 dB reject, 60 ms settling",'D', menu_postfiler_select, POST_FLTR_16_67_SPS }, 00818 }; 00819 00820 console_menu postfilter_select_menu = { 00821 .title = "Post-filter Selection Menu", 00822 .items = postfilter_select_items, 00823 .itemCount = ARRAY_SIZE(postfilter_select_items), 00824 .headerItem = NULL, 00825 .footerItem = NULL, 00826 .enableEscapeKey = true 00827 }; 00828 00829 00830 /* 00831 * Definition of the SINC5+SINC1 ODR select menu items and menu itself 00832 */ 00833 console_menu_item sinc5_1_data_rate_select_items[] = { 00834 #if defined(DEV_AD4115) 00835 { ODR_125000_STR, 'A', menu_odr_select, ODR_125000_BITS }, 00836 { ODR_62500_STR, 'B', menu_odr_select, ODR_62500_BITS }, 00837 { ODR_31250_STR, 'C', menu_odr_select, ODR_31250_BITS }, 00838 { ODR_25000_STR, 'D', menu_odr_select, ODR_25000_BITS }, 00839 { ODR_15625_STR, 'E', menu_odr_select, ODR_15625_BITS }, 00840 { ODR_10417_STR, 'F', menu_odr_select, ODR_10417_BITS }, 00841 { ODR_5000_STR, 'G', menu_odr_select, ODR_5000_BITS }, 00842 { ODR_2500_STR, 'H', menu_odr_select, ODR_2500_BITS }, 00843 { ODR_1000_STR, 'I', menu_odr_select, ODR_1000_BITS }, 00844 { ODR_500_STR, 'J', menu_odr_select, ODR_500_BITS }, 00845 { ODR_397_5_STR, 'K', menu_odr_select, ODR_397_5_BITS }, 00846 { ODR_200_STR, 'L', menu_odr_select, ODR_200_BITS }, 00847 { ODR_100_STR, 'M', menu_odr_select, ODR_100_BITS }, 00848 { ODR_59_98_STR, 'N', menu_odr_select, ODR_59_98_BITS }, 00849 { ODR_49_96_STR, 'O', menu_odr_select, ODR_49_96_BITS }, 00850 { ODR_20_STR, 'P', menu_odr_select, ODR_20_BITS }, 00851 { ODR_16_67_STR, 'Q', menu_odr_select, ODR_16_67_BITS }, 00852 { ODR_10_STR, 'R', menu_odr_select, ODR_10_BITS }, 00853 { ODR_5_STR, 'S', menu_odr_select, ODR_5_BITS }, 00854 { ODR_2_5_STR, 'T', menu_odr_select, ODR_2_5_BITS }, 00855 #elif defined(DEV_AD4111) || defined(DEV_AD4112) || defined(DEV_AD4114) || \ 00856 defined(DEV_AD7172_2) || defined(DEV_AD7172_4) || defined(DEV_AD7173_8) 00857 { ODR_31250_STR, 'A', menu_odr_select, ODR_31250_BITS }, 00858 { ODR_15625_STR, 'B', menu_odr_select, ODR_15625_BITS }, 00859 { ODR_10417_STR, 'C', menu_odr_select, ODR_10417_BITS }, 00860 { ODR_5208_STR, 'D', menu_odr_select, ODR_5208_BITS }, 00861 { ODR_2597_STR, 'E', menu_odr_select, ODR_2597_BITS }, 00862 { ODR_1007_STR, 'F', menu_odr_select, ODR_1007_BITS }, 00863 { ODR_503_8_STR, 'G', menu_odr_select, ODR_503_8_BITS }, 00864 { ODR_381_STR, 'H', menu_odr_select, ODR_381_BITS }, 00865 { ODR_200_3_STR, 'I', menu_odr_select, ODR_200_3_BITS }, 00866 { ODR_100_2_STR, 'J', menu_odr_select, ODR_100_2_BITS }, 00867 { ODR_59_52_STR, 'K', menu_odr_select, ODR_59_52_BITS }, 00868 { ODR_49_68_STR, 'L', menu_odr_select, ODR_49_68_BITS }, 00869 { ODR_20_01_STR, 'M', menu_odr_select, ODR_20_01_BITS }, 00870 { ODR_16_63_STR, 'N', menu_odr_select, ODR_16_63_BITS }, 00871 { ODR_10_STR, 'O', menu_odr_select, ODR_10_BITS }, 00872 { ODR_5_STR, 'P', menu_odr_select, ODR_5_BITS }, 00873 { ODR_2_5_STR, 'Q', menu_odr_select, ODR_2_5_BITS }, 00874 { ODR_1_25_STR, 'R', menu_odr_select, ODR_1_25_BITS }, 00875 #elif defined(DEV_AD7176_2) || defined(DEV_AD7175_2) || \ 00876 defined(DEV_AD7175_8) || defined(DEV_AD7177_2) 00877 #if (DEV_AD7176_2) || defined(DEV_AD7175_2) || defined(DEV_AD7175_8) 00878 { ODR_250000_STR, 'A', menu_odr_select, ODR_250000_BITS }, 00879 { ODR_125000_STR, 'B', menu_odr_select, ODR_125000_BITS }, 00880 { ODR_62500_STR, 'C', menu_odr_select, ODR_62500_BITS }, 00881 { ODR_50000_STR, 'D', menu_odr_select, ODR_50000_BITS }, 00882 { ODR_31250_STR, 'E', menu_odr_select, ODR_31250_BITS }, 00883 { ODR_25000_STR, 'F', menu_odr_select, ODR_25000_BITS }, 00884 { ODR_15625_STR, 'G', menu_odr_select, ODR_15625_BITS }, 00885 #endif 00886 { ODR_10000_STR, 'H', menu_odr_select, ODR_10000_BITS }, 00887 { ODR_5000_STR, 'I', menu_odr_select, ODR_5000_BITS }, 00888 { ODR_2500_STR, 'J', menu_odr_select, ODR_2500_BITS }, 00889 { ODR_1000_STR, 'K', menu_odr_select, ODR_1000_BITS }, 00890 { ODR_500_STR, 'L', menu_odr_select, ODR_500_BITS }, 00891 { ODR_397_5_STR, 'M', menu_odr_select, ODR_397_5_BITS }, 00892 { ODR_200_STR, 'N', menu_odr_select, ODR_200_BITS }, 00893 { ODR_100_STR, 'O', menu_odr_select, ODR_100_BITS }, 00894 { ODR_59_94_STR, 'P', menu_odr_select, ODR_59_94_BITS }, 00895 { ODR_49_96_STR, 'Q', menu_odr_select, ODR_49_96_BITS }, 00896 { ODR_20_STR, 'R', menu_odr_select, ODR_20_BITS }, 00897 { ODR_16_67_STR, 'S', menu_odr_select, ODR_16_67_BITS }, 00898 { ODR_10_STR, 'T', menu_odr_select, ODR_10_BITS }, 00899 { ODR_5_STR, 'U', menu_odr_select, ODR_5_BITS }, 00900 #endif 00901 }; 00902 00903 console_menu sinc5_1_data_rate_select_menu = { 00904 .title = "ODR Selection Menu", 00905 .items = sinc5_1_data_rate_select_items, 00906 .itemCount = ARRAY_SIZE(sinc5_1_data_rate_select_items), 00907 .headerItem = NULL, 00908 .footerItem = NULL, 00909 .enableEscapeKey = true 00910 }; 00911 00912 00913 /* 00914 * Definition of the SINC3 ODR select menu items and menu itself 00915 */ 00916 console_menu_item sinc3_data_rate_select_items[] = { 00917 #if defined(DEV_AD4115) 00918 { ODR_125000_STR, 'A', menu_odr_select, ODR_125000_BITS }, 00919 { ODR_62500_STR, 'B', menu_odr_select, ODR_62500_BITS }, 00920 { ODR_31250_STR, 'C', menu_odr_select, ODR_31250_BITS }, 00921 { ODR_25000_STR, 'D', menu_odr_select, ODR_25000_BITS }, 00922 { ODR_15625_STR, 'E', menu_odr_select, ODR_15625_BITS }, 00923 { ODR_10417_STR, 'F', menu_odr_select, ODR_10417_BITS }, 00924 { ODR_5000_STR, 'G', menu_odr_select, ODR_5000_BITS }, 00925 { ODR_3906_STR, 'H', menu_odr_select, ODR_3906_BITS }, 00926 { ODR_1157_STR, 'I', menu_odr_select, ODR_1157_BITS }, 00927 { ODR_539_STR, 'J', menu_odr_select, ODR_539_BITS }, 00928 { ODR_401_STR, 'K', menu_odr_select, ODR_401_BITS }, 00929 { ODR_206_STR, 'L', menu_odr_select, ODR_206_BITS }, 00930 { ODR_102_STR, 'M', menu_odr_select, ODR_102_BITS }, 00931 { ODR_59_98_STR, 'N', menu_odr_select, ODR_59_98_BITS }, 00932 { ODR_50_STR, 'O', menu_odr_select, ODR_50_BITS }, 00933 { ODR_20_STR, 'P', menu_odr_select, ODR_20_BITS }, 00934 { ODR_16_67_STR, 'Q', menu_odr_select, ODR_16_67_BITS }, 00935 { ODR_10_STR, 'R', menu_odr_select, ODR_10_BITS }, 00936 { ODR_5_STR, 'S', menu_odr_select, ODR_5_BITS }, 00937 { ODR_2_5_STR, 'T', menu_odr_select, ODR_2_5_BITS }, 00938 #elif defined(DEV_AD4111) || defined(DEV_AD4112) || defined(DEV_AD4114) || \ 00939 defined(DEV_AD7172_2) || defined(DEV_AD7172_4) || defined(DEV_AD7173_8) 00940 { ODR_31250_STR, 'A', menu_odr_select, ODR_31250_BITS }, 00941 { ODR_15625_STR, 'B', menu_odr_select, ODR_15625_BITS }, 00942 { ODR_10417_STR, 'C', menu_odr_select, ODR_10417_BITS }, 00943 { ODR_5208_STR, 'D', menu_odr_select, ODR_5208_BITS }, 00944 #if defined(DEV_AD4111) || defined(DEV_AD4112) || defined(DEV_AD4114) 00945 { ODR_3906_STR, 'E', menu_odr_select, ODR_3906_BITS }, 00946 { ODR_1157_STR, 'F', menu_odr_select, ODR_1157_BITS }, 00947 { ODR_539_STR, 'G', menu_odr_select, ODR_539_BITS }, 00948 { ODR_401_STR, 'H', menu_odr_select, ODR_401_BITS }, 00949 { ODR_206_STR, 'H', menu_odr_select, ODR_206_BITS }, 00950 { ODR_102_STR, 'I', menu_odr_select, ODR_102_BITS }, 00951 #else 00952 { ODR_2604_STR, 'E', menu_odr_select, ODR_2604_BITS }, 00953 { ODR_1008_STR, 'F', menu_odr_select, ODR_1008_BITS }, 00954 { ODR_504_STR, 'G', menu_odr_select, ODR_504_BITS }, 00955 { ODR_400_6_STR, 'H', menu_odr_select, ODR_400_6_BITS }, 00956 { ODR_200_3_STR, 'H', menu_odr_select, ODR_200_3_BITS }, 00957 { ODR_100_2_STR, 'I', menu_odr_select, ODR_100_2_BITS }, 00958 #endif 00959 { ODR_59_98_STR, 'J', menu_odr_select, ODR_59_98_BITS }, 00960 { ODR_50_STR, 'K', menu_odr_select, ODR_50_BITS }, 00961 { ODR_20_01_STR, 'L', menu_odr_select, ODR_20_01_BITS }, 00962 { ODR_16_67_STR, 'M', menu_odr_select, ODR_16_67_BITS }, 00963 { ODR_10_STR, 'N', menu_odr_select, ODR_10_BITS }, 00964 { ODR_5_STR, 'O', menu_odr_select, ODR_5_BITS }, 00965 { ODR_2_5_STR, 'P', menu_odr_select, ODR_2_5_BITS }, 00966 { ODR_1_25_STR, 'Q', menu_odr_select, ODR_1_25_BITS }, 00967 #elif defined(DEV_AD7176_2) || defined(DEV_AD7175_2) || \ 00968 defined(DEV_AD7175_8) || defined(DEV_AD7177_2) 00969 #if (DEV_AD7176_2) || defined(DEV_AD7175_2) || defined(DEV_AD7175_8) 00970 { ODR_250000_STR, 'A', menu_odr_select, ODR_250000_BITS }, 00971 { ODR_125000_STR, 'B', menu_odr_select, ODR_125000_BITS }, 00972 { ODR_62500_STR, 'C', menu_odr_select, ODR_62500_BITS }, 00973 { ODR_50000_STR, 'D', menu_odr_select, ODR_50000_BITS }, 00974 { ODR_31250_STR, 'E', menu_odr_select, ODR_31250_BITS }, 00975 { ODR_25000_STR, 'F', menu_odr_select, ODR_25000_BITS }, 00976 { ODR_15625_STR, 'G', menu_odr_select, ODR_15625_BITS }, 00977 #endif 00978 { ODR_10000_STR, 'H', menu_odr_select, ODR_10000_BITS }, 00979 { ODR_5000_STR, 'I', menu_odr_select, ODR_5000_BITS }, 00980 { ODR_2500_STR, 'J', menu_odr_select, ODR_2500_BITS }, 00981 { ODR_1000_STR, 'K', menu_odr_select, ODR_1000_BITS }, 00982 { ODR_500_STR, 'L', menu_odr_select, ODR_500_BITS }, 00983 { ODR_400_STR , 'M', menu_odr_select, ODR_400_BITS }, 00984 { ODR_200_STR, 'N', menu_odr_select, ODR_200_BITS }, 00985 { ODR_100_STR, 'O', menu_odr_select, ODR_100_BITS }, 00986 { ODR_60_STR, 'P', menu_odr_select, ODR_60_BITS }, 00987 { ODR_50_STR, 'Q', menu_odr_select, ODR_50_BITS }, 00988 { ODR_20_STR, 'R', menu_odr_select, ODR_20_BITS }, 00989 { ODR_16_67_STR, 'S', menu_odr_select, ODR_16_67_BITS }, 00990 { ODR_10_STR, 'T', menu_odr_select, ODR_10_BITS }, 00991 { ODR_5_STR, 'U', menu_odr_select, ODR_5_BITS }, 00992 #endif 00993 }; 00994 00995 console_menu sinc3_data_rate_select_menu = { 00996 .title = "ODR Selection Menu", 00997 .items = sinc3_data_rate_select_items, 00998 .itemCount = ARRAY_SIZE(sinc3_data_rate_select_items), 00999 .headerItem = NULL, 01000 .footerItem = NULL, 01001 .enableEscapeKey = true 01002 }; 01003 01004 01005 /* 01006 * Definition of the polarity select menu items and menu itself 01007 */ 01008 console_menu_item polarity_select_items[] = { 01009 { "Unipolar", 'U', menu_polarity_select, UNIPOLAR }, 01010 { "Bipolar", 'B', menu_polarity_select, BIPOLAR }, 01011 }; 01012 01013 console_menu polarity_select_menu = { 01014 .title = "Polarity Selection Menu", 01015 .items = polarity_select_items, 01016 .itemCount = ARRAY_SIZE(polarity_select_items), 01017 .headerItem = NULL, 01018 .footerItem = NULL, 01019 .enableEscapeKey = true 01020 }; 01021 01022 01023 /* 01024 * Definition of the refernce source selection menu items and menu itself 01025 */ 01026 console_menu_item reference_select_items[] = { 01027 { "External", 'A', menu_reference_source_select, EXTERNAL }, 01028 { "Internal", 'B', menu_reference_source_select, INTERNAL }, 01029 { "AVDD-AVSS", 'C', menu_reference_source_select, AVDD_AVSS }, 01030 }; 01031 01032 console_menu reference_select_menu = { 01033 .title = "Reference Selection Menu", 01034 .items = reference_select_items, 01035 .itemCount = ARRAY_SIZE(reference_select_items), 01036 .headerItem = NULL, 01037 .footerItem = NULL, 01038 .enableEscapeKey = true 01039 }; 01040 01041 01042 /* 01043 * Definition of the reference buffer enable/disable menu items and menu itself 01044 */ 01045 console_menu_item ref_buffer_enable_disable_items[] = { 01046 { "Enable", 'E', menu_ref_buffer_enable_disable, SELECT_ENABLE }, 01047 { "Disable", 'D', menu_ref_buffer_enable_disable, SELECT_DISBLE }, 01048 }; 01049 01050 console_menu ref_buffer_enable_disable_menu = { 01051 .title = "Reference Buffer Enable/Disable Menu", 01052 .items = ref_buffer_enable_disable_items, 01053 .itemCount = ARRAY_SIZE(ref_buffer_enable_disable_items), 01054 .headerItem = NULL, 01055 .footerItem = NULL, 01056 .enableEscapeKey = true 01057 }; 01058 01059 01060 /* 01061 * Definition of the reference buffer enable/disable menu items and menu itself 01062 */ 01063 console_menu_item input_buffer_enable_disable_items[] = { 01064 { "Enable", 'E', menu_input_buffer_enable_disable, SELECT_ENABLE }, 01065 { "Disable", 'D', menu_input_buffer_enable_disable, SELECT_DISBLE }, 01066 }; 01067 01068 console_menu input_buffer_enable_disable_menu = { 01069 .title = "Input Buffer Enable/Disable Menu", 01070 .items = input_buffer_enable_disable_items, 01071 .itemCount = ARRAY_SIZE(input_buffer_enable_disable_items), 01072 .headerItem = NULL, 01073 .footerItem = NULL, 01074 .enableEscapeKey = true 01075 }; 01076 01077 01078 /* 01079 * Definition of the Main Menu Items and menu itself 01080 */ 01081 console_menu_item main_menu_items[] = { 01082 { "Read Device ID", 'A', menu_read_id }, 01083 { "" }, 01084 { "Read Status Register", 'B', menu_read_status }, 01085 { "" }, 01086 { "Sample Channels", 'C', menu_sample_channels }, 01087 { "" }, 01088 { "Enable/Disable Channels", 'D', menu_chn_enable_disable_display }, 01089 { "" }, 01090 { "Connect Analog Inputs to Channel", 'E', menu_input_chn_connect_display }, 01091 { "" }, 01092 { "Configure and Assign Setup", 'F', menu_config_and_assign_setup }, 01093 { "" }, 01094 { "Display setup", 'G', menu_display_setup }, 01095 { "" }, 01096 { "Read Temperature", 'H', menu_read_temperature }, 01097 { "" }, 01098 { "Calibrate ADC (Internal)", 'I', menu_calibrate_adc }, 01099 { "" }, 01100 #if defined(DEV_AD4111) 01101 { "Open Wire Detection", 'J', menu_open_wire_detection }, 01102 { "" }, 01103 #endif 01104 { "Read/Write Device Registers", 'K', menu_read_write_device_regs }, 01105 }; 01106 01107 console_menu ad717x_main_menu = { 01108 .title = "Main Menu", 01109 .items = main_menu_items, 01110 .itemCount = ARRAY_SIZE(main_menu_items), 01111 .headerItem = display_main_menu_header, 01112 .footerItem = NULL, 01113 .enableEscapeKey = false 01114 }; 01115 01116 /******************************************************************************/ 01117 /************************ Public Declarations *********************************/ 01118 /******************************************************************************/ 01119 01120 #endif /* AD717X_MENU_DEFINES_H_ */
Generated on Wed Jul 13 2022 14:09:09 by
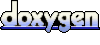