
Example program for AD717x and AD411x family of products.
Dependencies: adi_console_menu platform_drivers
ad717x_console_app.h
00001 /*! 00002 ***************************************************************************** 00003 @file: ad717x_console_app.h 00004 00005 @brief: Header for AD717x/AD411x console application interface. 00006 00007 @details: 00008 ----------------------------------------------------------------------------- 00009 Copyright (c) 2020 Analog Devices, Inc. 00010 All rights reserved. 00011 00012 This software is proprietary to Analog Devices, Inc. and its licensors. 00013 By using this software you agree to the terms of the associated 00014 Analog Devices Software License Agreement. 00015 *****************************************************************************/ 00016 00017 #ifndef AD717X_CONSOLE_APP_H_ 00018 #define AD717X_CONSOLE_APP_H_ 00019 00020 /******************************************************************************/ 00021 /***************************** Include Files **********************************/ 00022 /******************************************************************************/ 00023 00024 #include "adi_console_menu.h" 00025 #include "app_config.h" 00026 00027 /******************************************************************************/ 00028 /********************** Macros and Constants Definitions **********************/ 00029 /******************************************************************************/ 00030 00031 #define ADC_REF_VOLTAGE 2.5 // in volts 00032 00033 #if defined(DEV_AD7177_2) 00034 #define ADC_RESOLUTION 32 // in bits 00035 #else 00036 #define ADC_RESOLUTION 24 // in bits 00037 #endif 00038 00039 00040 // Define the number of channels for selected device 00041 #if defined(DEV_AD4111) || defined(DEV_AD4112) || \ 00042 defined(DEV_AD4114) || defined(DEV_AD4115) || \ 00043 defined(DEV_AD7173_8) || defined(DEV_AD7175_8) 00044 #define NUMBER_OF_CHANNELS 16U 00045 #elif defined(DEV_AD7172_4) 00046 #define NUMBER_OF_CHANNELS 8U 00047 #else 00048 #define NUMBER_OF_CHANNELS 4U 00049 #endif 00050 00051 00052 // Define the number of setups for selected device 00053 #if defined(DEV_AD4111) || defined(DEV_AD4112) || \ 00054 defined(DEV_AD4114) || defined(DEV_AD4115) || \ 00055 defined(DEV_AD7173_8) || defined(DEV_AD7172_4) || \ 00056 defined(DEV_AD7175_8) 00057 #define NUMBER_OF_SETUPS 8U 00058 #else 00059 #define NUMBER_OF_SETUPS 4U 00060 #endif 00061 00062 /******************************************************************************/ 00063 /********************** Variables and User Defined Data Types *****************/ 00064 /******************************************************************************/ 00065 00066 extern console_menu ad717x_main_menu; 00067 00068 /* AD717x Setup Configuration Structure */ 00069 typedef struct { 00070 uint32_t setup; // Selected setup 00071 uint32_t filter; // Filter type 00072 uint32_t postfilter; // Post filter type for SINC5+1 Filter 00073 uint32_t post_filter_enabled; // Post filter enable status 00074 uint32_t odr_bits; // Output data rate register bits 00075 uint32_t polarity; // Bipolar or Unipolar analog input 00076 uint32_t reference; // Reference source for ADC 00077 uint32_t input_buffers; // Buffers on analog inputs 00078 uint32_t reference_buffers; // Buffers on reference source 00079 uint32_t pos_analog_input; // Positive analog input 00080 uint32_t neg_analog_input; // Negative analog input 00081 uint32_t channel_enabled; // Channel Enable/Disable flag 00082 uint32_t setup_assigned; // Setup assigned to a channel 00083 } ad717x_setup_config; 00084 00085 /******************************************************************************/ 00086 /************************ Public Declarations *********************************/ 00087 /******************************************************************************/ 00088 00089 int32_t ad717x_app_initialize(void); 00090 00091 #endif /* AD717X_CONSOLE_APP_H_ */
Generated on Wed Jul 13 2022 14:09:09 by
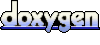