
Example program for EVAL-AD4130
Dependencies: tempsensors sdp_k1_sdram
ad4130_xattr.py
00001 # Copyright (C) 2022 Analog Devices, Inc. 00002 # 00003 # All rights reserved. 00004 # 00005 # Redistribution and use in source and binary forms, with or without modification, 00006 # are permitted provided that the following conditions are met: 00007 # - Redistributions of source code must retain the above copyright 00008 # notice, this list of conditions and the following disclaimer. 00009 # - Redistributions in binary form must reproduce the above copyright 00010 # notice, this list of conditions and the following disclaimer in 00011 # the documentation and/or other materials provided with the 00012 # distribution. 00013 # - Neither the name of Analog Devices, Inc. nor the names of its 00014 # contributors may be used to endorse or promote products derived 00015 # from this software without specific prior written permission. 00016 # - The use of this software may or may not infringe the patent rights 00017 # of one or more patent holders. This license does not release you 00018 # from the requirement that you obtain separate licenses from these 00019 # patent holders to use this software. 00020 # - Use of the software either in source or binary form, must be run 00021 # on or directly connected to an Analog Devices Inc. component. 00022 # 00023 # THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, 00024 # INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, MERCHANTABILITY AND FITNESS FOR A 00025 # PARTICULAR PURPOSE ARE DISCLAIMED. 00026 # 00027 # IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00028 # EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, INTELLECTUAL PROPERTY 00029 # RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR 00030 # BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, 00031 # STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF 00032 # THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 00034 from adi import ad4130 00035 from adi.attribute import attribute 00036 from decimal import Decimal 00037 00038 # Create a child class of ad4130 parent class for defining extended iio attributes (the ones which 00039 # are not part of original linux iio drivers and created for non-linux iio applications) 00040 class ad4130_xattr(ad4130): 00041 00042 # List of extended iio channels 00043 xchannel = [] 00044 00045 def __init__(self, uri, device_name): 00046 super().__init__(uri, device_name) 00047 00048 # Create an instances of the extended channel class 00049 for ch in self._ctrl.channels: 00050 name = ch._id 00051 self._rx_channel_names.append(name) 00052 self.xchannel.append(self._xchannel(self._ctrl, name)) 00053 00054 # Add the _xchannel class methods as _channel methods, so that they can be accessed with 00055 # attribute name as 'channel' instead 'xchannel' 00056 # e.g. channel attribute 'system_calibration' can be accessed as either "obj.channel[chn_num].system_calibration" Or 00057 # "obj.xchannel[chn_num].system_calibration", where obj refers to object of 'ad4130_xattr' class 00058 self._channel.system_calibration = self._xchannel.system_calibration 00059 self._channel.internal_calibration = self._xchannel.internal_calibration 00060 self._channel.loadcell_offset_calibration = self._xchannel.loadcell_offset_calibration 00061 self._channel.loadcell_gain_calibration = self._xchannel.loadcell_gain_calibration 00062 00063 #------------------------------------------------ 00064 # Device extended attributes 00065 #------------------------------------------------ 00066 00067 @property 00068 def demo_config(self): 00069 """AD4130 demo mode config""" 00070 return self._get_iio_dev_attr_str("demo_config") 00071 00072 @property 00073 def sample_rate(self): 00074 """AD4130 device sample_rate""" 00075 return int(self._get_iio_dev_attr_str("sampling_frequency")) 00076 00077 #------------------------------------------------ 00078 # Channel extended attributes 00079 #------------------------------------------------ 00080 00081 class _xchannel(attribute): 00082 00083 def __init__(self, ctrl, channel_name): 00084 self.name = channel_name 00085 self._ctrl = ctrl 00086 00087 @property 00088 def system_calibration(self): 00089 """AD4130 channel system calibration""" 00090 return self._get_iio_attr_str(self.name, "system_calibration", False) 00091 00092 @system_calibration.setter 00093 def system_calibration(self, value): 00094 self._set_iio_attr(self.name, "system_calibration", False, value) 00095 00096 @property 00097 def internal_calibration(self): 00098 """AD4130 channel internal calibration""" 00099 return self._get_iio_attr_str(self.name, "internal_calibration", False) 00100 00101 @internal_calibration.setter 00102 def internal_calibration(self, value): 00103 self._set_iio_attr(self.name, "internal_calibration", False, value) 00104 00105 @property 00106 def loadcell_offset_calibration(self): 00107 """AD4130 loadcell offset calibration""" 00108 return self._get_iio_attr_str(self.name, "loadcell_offset_calibration", False) 00109 00110 @loadcell_offset_calibration.setter 00111 def loadcell_offset_calibration(self, value): 00112 self._set_iio_attr(self.name, "loadcell_offset_calibration", False, value) 00113 00114 @property 00115 def loadcell_gain_calibration(self): 00116 """AD4130 loadcell gain calibration""" 00117 return self._get_iio_attr_str(self.name, "loadcell_gain_calibration", False) 00118 00119 @loadcell_gain_calibration.setter 00120 def loadcell_gain_calibration(self, value): 00121 self._set_iio_attr(self.name, "loadcell_gain_calibration", False, value) 00122
Generated on Wed Jul 20 2022 12:42:25 by
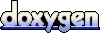