
Example program for EVAL-AD4130
Dependencies: tempsensors sdp_k1_sdram
ad4130_support.h
00001 /***************************************************************************//** 00002 * @file ad4130_support.h 00003 * @brief Header for AD4130 No-OS driver supports 00004 ******************************************************************************** 00005 * Copyright (c) 2020-2022 Analog Devices, Inc. 00006 * All rights reserved. 00007 * 00008 * This software is proprietary to Analog Devices, Inc. and its licensors. 00009 * By using this software you agree to the terms of the associated 00010 * Analog Devices Software License Agreement. 00011 *******************************************************************************/ 00012 00013 #ifndef AD4130_SUPPORT_H_ 00014 #define AD4130_SUPPORT_H_ 00015 00016 /******************************************************************************/ 00017 /***************************** Include Files **********************************/ 00018 /******************************************************************************/ 00019 00020 #include <stdint.h> 00021 #include "ad413x.h" 00022 00023 /******************************************************************************/ 00024 /********************** Macros and Constants Definition ***********************/ 00025 /******************************************************************************/ 00026 00027 #define AD413X_ADDR(x) ((x) & 0xFF) 00028 00029 #define AD4130_INT_SRC_SEL_MSK NO_OS_GENMASK(9, 8) 00030 #define AD4130_FILTER_FS_MSK NO_OS_GENMASK(10, 0) 00031 #define AD4130_FIFO_MODE_MSK NO_OS_GENMASK(17, 16) 00032 #define AD413X_WATERMARK_MSK NO_OS_GENMASK(7, 0) 00033 00034 #define AD413X_COMM_REG_RD NO_OS_BIT(6) 00035 00036 /******************************************************************************/ 00037 /********************** Variables and User Defined Data Types *****************/ 00038 /******************************************************************************/ 00039 00040 /* FIFO modes */ 00041 typedef enum { 00042 FIFO_DISABLED, 00043 FIFO_OLDEST_SAVE_MODE, 00044 FIFO_STREAM_MODE 00045 } fifo_mode_e; 00046 00047 /* ADC conversion interrupt source */ 00048 typedef enum { 00049 INT_PIN, 00050 CLK_PIN, 00051 GPIO1_PIN 00052 } adc_conv_int_source_e; 00053 00054 /******************************************************************************/ 00055 /************************ Public Declarations *********************************/ 00056 /******************************************************************************/ 00057 00058 float ad4130_get_reference_voltage(struct ad413x_dev *dev, uint8_t chn); 00059 int32_t perform_sign_conversion(struct ad413x_dev *dev, uint32_t adc_raw_data, 00060 uint8_t chn); 00061 float convert_adc_sample_into_voltage(void *dev, uint32_t adc_raw, 00062 uint8_t chn); 00063 float convert_adc_raw_into_rtd_resistance(void *dev, uint32_t adc_raw, 00064 float rtd_ref, uint8_t chn); 00065 int32_t ad4130_read_fifo(struct ad413x_dev *dev, uint32_t *data, 00066 uint32_t adc_samples); 00067 int32_t ad413x_mon_conv_and_read_data(struct ad413x_dev *dev, 00068 uint32_t *raw_data); 00069 int32_t ad413x_set_int_source(struct ad413x_dev *dev, 00070 adc_conv_int_source_e conv_int_source); 00071 int32_t ad413x_set_filter_fs(struct ad413x_dev *dev, uint32_t fs, 00072 uint8_t preset); 00073 00074 #endif /* AD4130_SUPPORT_H_ */
Generated on Wed Jul 20 2022 12:42:25 by
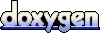