
Example program for EVAL-AD4130
Dependencies: tempsensors sdp_k1_sdram
ad4130_sensor_measurement.py
00001 import serial 00002 from time import sleep 00003 from pynput import keyboard 00004 from adi import ad4130 00005 from ad4130_xattr import * 00006 00007 # Delays in seconds 00008 short_time = 0.1 00009 long_time = 1 00010 loadcell_settling_time = 2 00011 00012 # Global variables 00013 weight_input = 0 00014 loadcell_offset = [ 0 ] 00015 loadcell_gain = [ 0 ] 00016 00017 # Power test channels defined in the firmware 00018 POWER_TEST_V_AVDD_CHN = 0 00019 POWER_TEST_V_IOVDD_CHN = 1 00020 POWER_TEST_I_AVDD_CHN = 2 00021 POWER_TEST_I_IOVDD_CHN = 3 00022 POWER_TEST_V_AVSS_DGND_CHN = 4 00023 POWER_TEST_V_REF_CHN = 5 00024 00025 # IIO Channel name and respective channel index mapping 00026 chn_mappping = { 00027 "voltage0" : 0, "voltage1" : 1, "voltage2" : 2, "voltage3" : 3, "voltage4" : 4, 00028 "voltage5" : 5, "voltage6" : 6, "voltage7" : 7, "voltage8" : 8, "voltage9" : 9, 00029 "voltage10" : 10, "voltage11" : 11, "voltage12" : 12, "voltage13" : 13, 00030 "voltage14" : 14, "voltage15" : 15, 00031 00032 "current0" : 0, "current1" : 1, "current2" : 2, "current3" : 3, "current4" : 4, 00033 "current5" : 5, "current6" : 6, "current7" : 7, "current8" : 8, "current9" : 9, 00034 "current10" : 10, "current11" : 11, "current12" : 12, "current13" : 13, 00035 "current14" : 14, "current15" : 15, 00036 00037 "temp0" : 0, "temp1" : 1, "temp2" : 2, "temp3" : 3, "temp4" : 4, 00038 "temp5" : 5, "temp6" : 6, "temp7" : 7, "temp8" : 8, "temp9" : 9, 00039 "temp10" : 10, "temp11" : 11, "temp12" : 12, "temp13" : 13, 00040 "temp14" : 14, "temp15" : 15, 00041 } 00042 00043 def key_press_event(key): 00044 global key_pressed 00045 key_pressed = True 00046 00047 def init_sensor_measurement(): 00048 global device 00049 global demo_config 00050 global listener 00051 00052 ######## User configuration ########## 00053 # Configure the backend for PC to IIOD interface 00054 uri = "serial:COM12,230400" # For UART, baud rate must be same as set in the FW. COM port is physical Or VCOM. 00055 device_name = "ad4130-8" # Name of the device must be same as set in the FW. 00056 ###################################### 00057 00058 # Create an IIO device context 00059 device = ad4130_xattr(uri, device_name) 00060 device._ctx.set_timeout(100000) 00061 00062 # Get current user device config from the firmware 00063 demo_config = device.demo_config 00064 print("\r\nDemo Config: {}\r\n".format(demo_config)) 00065 00066 listener = keyboard.Listener(on_press=key_press_event) 00067 listener.start() 00068 00069 def perform_loadcell_calibration(chn): 00070 global loadcell_offset 00071 global loadcell_gain 00072 global weight_input 00073 global device 00074 00075 chn_indx = chn_mappping[chn.name] 00076 00077 print("\r\nCalibrating Loadcell for channel {}".format(chn_indx)) 00078 input("Please ensure no weight is applied on Loadcell and press enter to continue calibration ") 00079 print("Waiting to settle-down the Loadcell..") 00080 sleep(loadcell_settling_time) 00081 print("Performing Loadcell offset calibration..") 00082 chn.loadcell_offset_calibration = 'start_calibration' 00083 print("Loadcell offset calibration complete") 00084 loadcell_offset[chn_indx] = chn.loadcell_offset_calibration 00085 print("Loadcell offset: {}".format(loadcell_offset[chn_indx])) 00086 00087 weight_input_done = False 00088 while weight_input_done is False: 00089 try: 00090 weight_input = int(input("\r\nApply the weight on loadcell and enter here (in grams): ")) 00091 if (weight_input <= 0): 00092 print("Please ensure weight is > 0!!") 00093 else: 00094 weight_input_done = True 00095 except: 00096 print("Invalid input") 00097 00098 print("Waiting to settle-down the Loadcell..") 00099 sleep(loadcell_settling_time) 00100 print("Performing load cell gain calibration..") 00101 chn.loadcell_gain_calibration = 'start_calibration' 00102 print("Load cell gain calibration complete") 00103 loadcell_gain[chn_indx] = chn.loadcell_gain_calibration 00104 print("Loadcell gain: {}".format(loadcell_gain[chn_indx])) 00105 00106 def perform_sensor_measurement(): 00107 global device 00108 global key_pressed 00109 global loadcell_offset 00110 global loadcell_gain 00111 global weight_input 00112 00113 # Loadcell must be calibrated before performing measurement 00114 if (demo_config == 'Loadcell'): 00115 for chn in device.channel: 00116 perform_loadcell_calibration(chn) 00117 00118 weight = 0 00119 print("\r\n*** Press any key to stop the measurement ***\r\n") 00120 sleep(long_time) 00121 00122 # Print the header 00123 header = "" 00124 for chn in device.channel: 00125 header = header + chn.name + ' ' 00126 print(header) 00127 00128 key_pressed = False 00129 while not key_pressed: 00130 result_str = "" 00131 for chn in device.channel: 00132 sleep(short_time) 00133 if (demo_config == 'Loadcell'): 00134 adc_raw = chn.raw 00135 try: 00136 chn_indx = chn_mappping[chn.name] 00137 weight = ((adc_raw - loadcell_offset[chn_indx]) * weight_input) / (loadcell_gain[chn_indx] - loadcell_offset[chn_indx]) 00138 result_str = result_str + str(round(weight,4)) + ' gram ' 00139 except: 00140 print("\r\nInvalid measurement result. Please check device settings!!") 00141 break 00142 elif (demo_config == 'ECG' or demo_config == 'Noise Test' or demo_config == 'Power Test' or demo_config == 'User Default'): 00143 adc_raw = chn.raw 00144 scale = chn.scale 00145 offset = chn.offset 00146 if (demo_config == 'Power Test'): 00147 chn_indx = chn_mappping[chn.name] 00148 if (chn_indx == POWER_TEST_I_AVDD_CHN or chn_indx == POWER_TEST_I_IOVDD_CHN): 00149 current = ((adc_raw + offset) * scale) 00150 result_str = result_str + str(round(current,4)) + 'mA ' 00151 else: 00152 voltage = ((adc_raw + offset) * scale) / 1000 00153 result_str = result_str + str(round(voltage,4)) + 'V ' 00154 else: 00155 voltage = ((adc_raw + offset) * scale) / 1000 00156 result_str = result_str + str(round(voltage,4)) + 'V ' 00157 else: 00158 # Temperature sensors demo configs (RTD, Thermocouple and Thermistor) 00159 adc_raw = chn.raw 00160 scale = chn.scale 00161 temperature = (adc_raw * scale) / 1000 00162 result_str = result_str + str(round(temperature,4)) + 'C ' 00163 00164 print(result_str) 00165 00166 def exit(): 00167 global listener 00168 global device 00169 00170 # Delete the objects 00171 del listener 00172 del device 00173 00174 def main(): 00175 init_sensor_measurement() 00176 perform_sensor_measurement() 00177 exit() 00178 00179 if __name__ == "__main__": 00180 main()
Generated on Wed Jul 20 2022 12:42:25 by
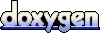