
Example program for EVAL-AD4130
Dependencies: tempsensors sdp_k1_sdram
ad4130_calibration.py
00001 import serial 00002 from time import sleep 00003 from adi import ad4130 00004 from ad4130_xattr import * 00005 00006 # Delays in second 00007 short_time = 0.2 00008 00009 # Calibration type identifiers 00010 internal_calibration = '1' 00011 system_calibration = '2' 00012 00013 # Analog input mapping as configured in the firmware 00014 ain_mapping = { 00015 "User Default" : [ 'AIN0-AVSS', 'AIN1-AVSS', 'AIN2-AVSS', 'AIN3-AVSS', 00016 'AIN4-AVSS', 'AIN5-AVSS', 'AIN6-AVSS', 'AIN7-AVSS', 00017 'AIN8-AVSS', 'AIN9-AVSS', 'AIN10-AVSS', 'AIN11-AVSS', 00018 'AIN12-AVSS', 'AIN13-AVSS', 'AIN14-AVSS', 'AIN15-AVSS' 00019 ], 00020 "Thermistor" : [ 'AIN4-AIN5' ], 00021 "Thermocouple" : [ 'AIN2-AIN3', 'AIN4-AIN5' ], 00022 "2-Wire RTD" : [ 'AIN2-AIN3' ], 00023 "3-Wire RTD" : [ 'AIN2-AIN3' ], 00024 "4-Wire RTD" : [ 'AIN2-AIN3' ], 00025 "Loadcell" : [ 'AIN5-AIN6' ], 00026 "ECG" : [ 'AIN11-AIN14' ], 00027 "Noise Test" : [ 'AIN0-AIN1' ], 00028 } 00029 00030 # IIO Channel name and respective channel index mapping 00031 chn_mappping = { 00032 "voltage0" : 0, "voltage1" : 1, "voltage2" : 2, "voltage3" : 3, "voltage4" : 4, 00033 "voltage5" : 5, "voltage6" : 6, "voltage7" : 7, "voltage8" : 8, "voltage9" : 9, 00034 "voltage10" : 10, "voltage11" : 11, "voltage12" : 12, "voltage13" : 13, 00035 "voltage14" : 14, "voltage15" : 15, 00036 00037 "current0" : 0, "current1" : 1, "current2" : 2, "current3" : 3, "current4" : 4, 00038 "current5" : 5, "current6" : 6, "current7" : 7, "current8" : 8, "current9" : 9, 00039 "current10" : 10, "current11" : 11, "current12" : 12, "current13" : 13, 00040 "current14" : 14, "current15" : 15, 00041 00042 "temp0" : 0, "temp1" : 1, "temp2" : 2, "temp3" : 3, "temp4" : 4, 00043 "temp5" : 5, "temp6" : 6, "temp7" : 7, "temp8" : 8, "temp9" : 9, 00044 "temp10" : 10, "temp11" : 11, "temp12" : 12, "temp13" : 13, 00045 "temp14" : 14, "temp15" : 15, 00046 } 00047 00048 def init_calibration(): 00049 global device 00050 global demo_config 00051 00052 ######## User configuration ########## 00053 # Configure the backend for PC to IIOD interface 00054 uri = "serial:COM12,230400" # For UART, baud rate must be same as set in the FW. COM port is physical Or VCOM. 00055 device_name = "ad4130-8" # Name of the device must be same as set in the FW. 00056 ###################################### 00057 00058 # Create an IIO device context 00059 device = ad4130_xattr(uri, device_name) 00060 device._ctx.set_timeout(100000) 00061 00062 # Get current user device config from the firmware 00063 demo_config = device.demo_config 00064 print("\r\nDemo Config: {}\r\n".format(demo_config)) 00065 00066 def get_calibration_status(calibration_type, chn, chn_index): 00067 global gain_before_calib, gain_after_calib 00068 global offset_before_calib, offset_after_calib 00069 global calibration_status 00070 00071 if (calibration_type == system_calibration): 00072 calib_status = chn.system_calibration 00073 else: 00074 calib_status = chn.internal_calibration 00075 00076 gain_before_calib = calib_status[0:8] 00077 gain_after_calib = calib_status[8:16] 00078 offset_before_calib = calib_status[16:24] 00079 offset_after_calib = calib_status[24:32] 00080 calibration_status = calib_status[32:] 00081 00082 def perform_calibration(): 00083 00084 if (demo_config == "Power Test"): 00085 # Power test uses internal ADC channels, on which calibration can't be performed 00086 print("Invalid demo mode config. Calibration can't be performed on internal ADC channels!!") 00087 return 00088 00089 # Select calibration type 00090 calibration_type = input("\r\nSelect Calibration Type:\r\n\ 00091 {}. Internal Calibration\r\n\ 00092 {}. System Calibration\r\n".format(internal_calibration, system_calibration)) 00093 if (calibration_type > system_calibration): 00094 print("Invalid Input!!") 00095 return 00096 00097 # Perform calibration for all channels 00098 for chn in device.channel: 00099 chn_index = chn_mappping[chn.name] 00100 print("-------------------------------------------") 00101 print("Calibrating channel {} ".format(chn_index)) 00102 00103 if (calibration_type == system_calibration): 00104 # Perform zero-scale (offset) system calibration 00105 val = input("Apply zero-scale voltage between {} and press enter".format(ain_mapping[demo_config][chn_index])) 00106 chn.system_calibration = "start_calibration" 00107 sleep(short_time) 00108 get_calibration_status(calibration_type, chn, chn_index) 00109 print("Offset (before calibration): 0x{}".format(offset_before_calib)) 00110 print("Offset (after calibration): 0x{}".format(offset_after_calib)) 00111 if (calibration_status == "calibration_done"): 00112 print("System offset calibration successfull..\r\n") 00113 else: 00114 print("System offset calibration failed!!\r\n") 00115 00116 # Perform full-scale (gain) system calibration 00117 val = input("Apply full-scale voltage between {} and press enter".format(ain_mapping[demo_config][chn_index])) 00118 chn.system_calibration = "start_calibration" 00119 sleep(short_time) 00120 get_calibration_status(calibration_type, chn, chn_index) 00121 print("Gain (before calibration): 0x{}".format(gain_before_calib)) 00122 print("Gain (after calibration): 0x{}".format(gain_after_calib)) 00123 if (calibration_status == "calibration_done"): 00124 print("System gain calibration successfull..\r\n") 00125 else: 00126 print("System gain calibration failed!!\r\n") 00127 else: 00128 # Perform full-scale (gain) internal calibration 00129 chn.internal_calibration = "start_calibration" 00130 sleep(short_time) 00131 get_calibration_status(calibration_type, chn, chn_index) 00132 print("Gain (before calibration): 0x{}".format(gain_before_calib)) 00133 print("Gain (after calibration): 0x{}".format(gain_after_calib)) 00134 if (calibration_status == "calibration_done"): 00135 print("Internal gain calibration successfull..\r\n") 00136 elif (calibration_status == "calibration_skipped"): 00137 print("Internal gain calibration skipped due to PGA=1\r\n") 00138 else: 00139 print("Internal gain calibration failed..\r\n") 00140 00141 # Perform zero-scale (offset) internal calibration 00142 chn.internal_calibration = "start_calibration" 00143 sleep(short_time) 00144 get_calibration_status(calibration_type, chn, chn_index) 00145 print("Offset (before calibration): 0x{}".format(offset_before_calib)) 00146 print("Offset (after calibration): 0x{}".format(offset_after_calib)) 00147 if (calibration_status == "calibration_done"): 00148 print("Internal offset calibration successfull..\r\n") 00149 else: 00150 print("Internal offset calibration failed!!\r\n") 00151 00152 def exit(): 00153 global device 00154 00155 # Delete the objects 00156 del device 00157 00158 def main(): 00159 init_calibration() 00160 perform_calibration() 00161 exit() 00162 00163 if __name__ == "__main__": 00164 main()
Generated on Wed Jul 20 2022 12:42:25 by
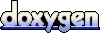