Ambient Library
Fork of AmbientLib by
Embed:
(wiki syntax)
Show/hide line numbers
Ambient.h
00001 #ifndef AMBIENT_H 00002 #define AMBIENT_H 00003 00004 #include "mbed.h" 00005 #include "TCPSocketConnection.h" 00006 00007 #define AMBIENT_WRITEKEY_SIZE 18 00008 #define AMBIENT_MAX_RETRY 5 00009 #define AMBIENT_DATA_SIZE 24 00010 #define AMBIENT_NUM_PARAMS 11 00011 00012 /** AMBIENT class 00013 * to send data to Ambient service. 00014 * 00015 * Exsample: 00016 * @code 00017 * #include "mbed.h" 00018 * #include "EthernetInterface.h" 00019 * #include "Ambient.h" 00020 * #include "HDC1000.h" 00021 * 00022 * unsigned int channelId = 100; 00023 * const char* writeKey = "ライトキー"; 00024 * AMBIENT ambient; 00025 * 00026 * HDC1000 hdc1000(p9,p10); 00027 * 00028 * int main() { 00029 * printf("start\r\n"); 00030 * 00031 * EthernetInterface eth; 00032 * eth.init(); //Use DHCP 00033 * eth.connect(); 00034 * 00035 * TCPSocketConnection socket; 00036 * ambient.init(channelId, writeKey, &socket); 00037 * 00038 * printf("Ambient send to ch: %d\r\n", channelId); 00039 * 00040 * while (true) { 00041 * float temp, humid; 00042 * char tempbuf[12], humidbuf[12]; 00043 * 00044 * hdc1000.get(); 00045 * temp = hdc1000.temperature(); 00046 * humid = hdc1000.humidity(); 00047 * 00048 * sprintf(tempbuf, "%2.1f", temp); 00049 * ambient.set(1, tempbuf); 00050 * sprintf(humidbuf, "%2.0f", humid); 00051 * ambient.set(2, humidbuf); 00052 * printf("Temp: %s C, Humid: %s %%\r\n", tempbuf, humidbuf); 00053 * 00054 * ambient.send(); 00055 * 00056 * wait(30.0); 00057 * } 00058 * } 00059 * @endcode 00060 */ 00061 class AMBIENT 00062 { 00063 public: 00064 /** Create AMBIENT instance 00065 */ 00066 AMBIENT(void); 00067 00068 /** Initialize the instance 00069 * @param channelId Initialize the Ambient instance with channelId. 00070 * @param writeKey and writeKey 00071 * @param s and pointer to socket 00072 * @returns 00073 * true on success, 00074 * false on error 00075 */ 00076 bool init(unsigned int channelId, const char * writeKey, TCPSocketConnection * s, int dev = 0); 00077 /** Set data on field-th field of payload. 00078 * @param field index of payload (1 to 8) 00079 * @param data data 00080 * @returns 00081 * true on success, 00082 * false on error 00083 */ 00084 bool set(int field, char * data); 00085 /** Clear data on field-th field of payload. 00086 * @param field index of payload (1 to 8) 00087 * @returns 00088 * true on success, 00089 * false on error 00090 */ 00091 bool clear(int field); 00092 00093 /** Send data to Ambient 00094 */ 00095 bool send(void); 00096 00097 private: 00098 00099 TCPSocketConnection * s; 00100 unsigned int channelId; 00101 char writeKey[AMBIENT_WRITEKEY_SIZE]; 00102 int dev; 00103 char host[18]; 00104 int port; 00105 00106 struct { 00107 int set; 00108 char item[AMBIENT_DATA_SIZE]; 00109 } data[AMBIENT_NUM_PARAMS]; 00110 }; 00111 00112 #endif // AMBIENT_H
Generated on Fri Jul 15 2022 18:17:02 by
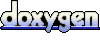