
Interrupt to switch ON/OFF LED depending on Button Status
Dependencies: mbed
LED-Button-Interrupt.cpp
00001 #include "mbed.h" 00002 00003 // inizializza oggetti 00004 InterruptIn myButton(USER_BUTTON); 00005 DigitalOut myLed(LED1); 00006 00007 // dichiara variabile. Inizialmente delay lunghissimo 00008 double dDelay = 2000; // 2 sec 00009 00010 00011 /*************************************************************/ 00012 /* Routine di gestione Interrupt associata al button pressed */ 00013 /*************************************************************/ 00014 void pressedIRQ() 00015 { 00016 // assegna valore alla variabile dDelay che sarà poi usata nel Main per generare un ritardo 00017 dDelay = 100; // 100 ms 00018 } 00019 00020 /**************************************************************/ 00021 /* Routine di gestione Interrupt associata al button released */ 00022 /**************************************************************/ 00023 void releasedIRQ() 00024 { 00025 // assegna valore alla variabile dDelay che sarà poi usata nel Main per generare un ritardo 00026 dDelay = 500; // 500 ms 00027 } 00028 00029 00030 /********/ 00031 /* MAIN */ 00032 /********/ 00033 int main() 00034 { 00035 00036 // Associa routine di Interrup all'evento Button premuto 00037 myButton.fall(&pressedIRQ); // Ogni volta che premo Button viene richiamata la routine pressedIRQ 00038 // Associa routine di Interrup all'evento Button rilasciato 00039 myButton.rise(&releasedIRQ); 00040 00041 // POLLING: accende/spegne il LED con un ritardo diverso a seconda che sia premuto/rilasciato il pulsante 00042 while (true) 00043 { 00044 // accende/spegne il LED 00045 myLed = !myLed; 00046 00047 // Il delay dipende dalla posizione Pressed/Released del pulsante 00048 wait_ms(dDelay); 00049 00050 // posso aumentare la precisione del ritardo impostandolo in usec 00051 //wait_us(dDelay); 00052 } 00053 }
Generated on Mon Aug 15 2022 11:58:23 by
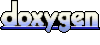