
Lora Personalized device for Everynet
Dependencies: LMiCPersonalizedforEverynet SX1276Lib X_NUCLEO_IKS01A1 cantcoap lwip mbed-rtos mbed
Fork of LoRaWAN-test-10secs by
debug.cpp
00001 /******************************************************************************* 00002 * Copyright (c) 2014-2015 IBM Corporation. 00003 * All rights reserved. This program and the accompanying materials 00004 * are made available under the terms of the Eclipse Public License v1.0 00005 * which accompanies this distribution, and is available at 00006 * http://www.eclipse.org/legal/epl-v10.html 00007 * 00008 * Contributors: 00009 * IBM Zurich Research Lab - initial API, implementation and documentation 00010 * Semtech Apps Team - Adapted for MBED 00011 *******************************************************************************/ 00012 #include <stdio.h> 00013 #include "lmic.h" 00014 #include "debug.h" 00015 00016 #include "mbed.h" 00017 Serial pc(USBTX, USBRX); 00018 00019 void debug_init () { 00020 pc.baud(9600); 00021 // print banner 00022 debug_str("\r\n============== DEBUG STARTED ==============\r\n"); 00023 } 00024 00025 void debug_led (u1_t val) { 00026 debug_val( "LED = ", val ); 00027 } 00028 00029 void debug_char (u1_t c) { 00030 pc.printf("%c", c ); 00031 } 00032 00033 void debug_hex (u1_t b) { 00034 pc.printf("%02X", b ); 00035 } 00036 00037 void debug_buf (const u1_t* buf, u2_t len) { 00038 while( len-- ) { 00039 debug_hex( *buf++ ); 00040 debug_char( ' ' ); 00041 } 00042 debug_char( '\r' ); 00043 debug_char( '\n' ); 00044 } 00045 00046 void debug_uint (u4_t v) { 00047 for( s1_t n = 24; n >= 0; n -= 8 ) { 00048 debug_hex( v >> n ); 00049 } 00050 } 00051 00052 void debug_str (const char* str) { 00053 /*while( *str ) { 00054 debug_char( *str++ ); 00055 }*/ 00056 pc.printf(str); 00057 } 00058 00059 void debug_str (const u1_t* str) { 00060 while( *str ) { 00061 debug_char( *str++ ); 00062 } 00063 } 00064 00065 void debug_val (const char* label, u4_t val) { 00066 debug_str( label ); 00067 debug_uint( val ); 00068 debug_char( '\r' ); 00069 debug_char( '\n' ); 00070 } 00071 00072 void debug_val (const u1_t* label, u4_t val) { 00073 debug_str( label ); 00074 debug_uint( val ); 00075 debug_char( '\r' ); 00076 debug_char( '\n' ); 00077 } 00078 00079 void debug_event (int ev) { 00080 static const char* evnames[] = { 00081 [EV_SCAN_TIMEOUT] = "SCAN_TIMEOUT", 00082 [EV_BEACON_FOUND] = "BEACON_FOUND", 00083 [EV_BEACON_MISSED] = "BEACON_MISSED", 00084 [EV_BEACON_TRACKED] = "BEACON_TRACKED", 00085 [EV_JOINING] = "JOINING", 00086 [EV_JOINED] = "JOINED", 00087 [EV_RFU1] = "RFU1", 00088 [EV_JOIN_FAILED] = "JOIN_FAILED", 00089 [EV_REJOIN_FAILED] = "REJOIN_FAILED", 00090 [EV_TXCOMPLETE] = "TXCOMPLETE", 00091 [EV_LOST_TSYNC] = "LOST_TSYNC", 00092 [EV_RESET] = "RESET", 00093 [EV_RXCOMPLETE] = "RXCOMPLETE", 00094 [EV_LINK_DEAD] = "LINK_DEAD", 00095 [EV_LINK_ALIVE] = "LINK_ALIVE", 00096 }; 00097 debug_str(evnames[ev]); 00098 debug_char('\r'); 00099 debug_char('\n'); 00100 }
Generated on Wed Jul 13 2022 06:25:04 by
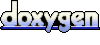