
Atlas data logger
Dependencies: MPU9150 SDFileSystem mbed
Atlas.cpp
00001 #include "mbed.h" 00002 #include "Atlas.h" 00003 #include "SDFileSystem.h" 00004 00005 DigitalOut grn(LED_GRN); 00006 DigitalOut ylw(LED_YLW); 00007 Serial pc(USBTX, USBRX); 00008 Serial gps(GPS_TX, GPS_RX); 00009 SDFileSystem sd(SPI_MOSI, SPI_MISO, SPI_SCK, SPI_CS, "sd"); 00010 00011 void init() { 00012 pc.baud(115200); 00013 gps.baud(115200); 00014 grn = LED_ON; 00015 ylw = LED_ON; 00016 00017 printf("Attempting to open SD card...\n"); 00018 mkdir("/sd/mydir", 0777); 00019 00020 printf("Attempting to open file...\n"); 00021 FILE *fp = fopen("/sd/mydir/sdtest.txt", "w"); 00022 if(fp == NULL) { 00023 error("Could not open file for write\n"); 00024 ylw = LED_OFF; 00025 } 00026 fprintf(fp, "Hello fun SD Card World!"); 00027 00028 printf("Closing file...\n"); 00029 fclose(fp); 00030 00031 pc.printf("Atlas Ready!\n"); 00032 } 00033 00034 int main() { 00035 init(); 00036 while(true) { 00037 if(gps.readable()) { 00038 pc.putc(gps.getc()); 00039 } 00040 } 00041 }
Generated on Thu Jul 14 2022 05:51:15 by
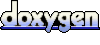