
Use this "Hello-World" program to stream accelerometer, magnetometer and gyroscope sensor data from FRDM-STBC-AGM01 to the cloud using AT&T M2X Data Services.
Dependencies: EthernetInterface FXAS21002 FXOS8700 M2XStreamClient jsonlite mbed-rtos mbed
main.cpp
00001 /* 00002 * Copyright (c) 2015 - 2016, Freescale Semiconductor, Inc. 00003 * Copyright 2016-2017 NXP 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * o Redistributions of source code must retain the above copyright notice, this list 00009 * of conditions and the following disclaimer. 00010 * 00011 * o Redistributions in binary form must reproduce the above copyright notice, this 00012 * list of conditions and the following disclaimer in the documentation and/or 00013 * other materials provided with the distribution. 00014 * 00015 * o Neither the name of the copyright holder nor the names of its 00016 * contributors may be used to endorse or promote products derived from this 00017 * software without specific prior written permission. 00018 * 00019 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00020 * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00021 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00022 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR 00023 * ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00024 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00025 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON 00026 * ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00027 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00028 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00029 */ 00030 #include "FXAS21002.h" 00031 #include "FXOS8700.h" 00032 #include "mbed.h" 00033 #include "M2XStreamClient.h" 00034 #include "EthernetInterface.h" 00035 00036 // Initialize Serial port 00037 Serial pc(USBTX, USBRX); 00038 00039 // Initialize pins for I2C communication for sensors. Set jumpers J6,J7 in FRDM-STBC-AGM01 board accordingly. 00040 FXOS8700 accel(D14,D15); 00041 FXOS8700 mag(D14,D15); 00042 FXAS21002 gyro(D14,D15); 00043 00044 // Set Sensor Stream details 00045 char deviceId[] = "8b34bc421abf15b7ec6471fa19513a98"; // Device you want to push to 00046 char streamAcc[] = "acc_rms"; // Stream you want to push to 00047 char streamMag[] = "mag_rms"; // Stream you want to push to 00048 char streamGyr[] = "gyr_rms"; // Stream you want to push to 00049 char m2xKey[] = "737018ea33de7760ab346c85ae2d9d27"; // Your M2X API Key or Master API Key 00050 00051 int main() 00052 { 00053 // Intialize Ethernet connection 00054 EthernetInterface eth; 00055 eth.init(); 00056 eth.connect(); 00057 printf("Success. Connected!. Device IP Address is %s\r\n", eth.getIPAddress()); 00058 00059 // Initialize the M2X client 00060 Client client; 00061 M2XStreamClient m2xClient(&client, m2xKey); 00062 int ret; 00063 00064 00065 // Configure Accelerometer FXOS8700, Magnetometer FXOS8700 & Gyroscope FXAS21002 00066 accel.accel_config(); 00067 mag.mag_config(); 00068 gyro.gyro_config(); 00069 00070 float accel_data[3]; float accel_rms=0.0; 00071 float mag_data[3]; float mag_rms=0.0; 00072 float gyro_data[3]; float gyro_rms=0.0; 00073 00074 printf("Begin Data Acquisition from FXOS8700 and FXAS21002....\r\n\r\n"); 00075 wait(0.5); 00076 00077 while(1) 00078 { 00079 accel.acquire_accel_data_g(accel_data); 00080 accel_rms = sqrt(((accel_data[0]*accel_data[0])+(accel_data[1]*accel_data[1])+(accel_data[2]*accel_data[2]))/3); 00081 printf("%4.2f,\t%4.2f,\t%4.2f,\t",accel_data[0],accel_data[1],accel_data[2]); 00082 wait(0.01); 00083 00084 mag.acquire_mag_data_uT(mag_data); 00085 printf("%4.2f,\t%4.2f,\t%4.2f,\t",mag_data[0],mag_data[1],mag_data[2]); 00086 mag_rms = sqrt(((mag_data[0]*mag_data[0])+(mag_data[1]*mag_data[1])+(mag_data[2]*mag_data[2]))/3); 00087 wait(0.01); 00088 00089 gyro.acquire_gyro_data_dps(gyro_data); 00090 printf("%4.2f,\t%4.2f,\t%4.2f\r\n",gyro_data[0],gyro_data[1],gyro_data[2]); 00091 gyro_rms = sqrt(((gyro_data[0]*gyro_data[0])+(gyro_data[1]*gyro_data[1])+(gyro_data[2]*gyro_data[2]))/3); 00092 wait(0.01); 00093 00094 ret = m2xClient.updateStreamValue(deviceId, streamAcc, accel_rms); 00095 printf("send() returned %d\r\n", ret); 00096 ret = m2xClient.updateStreamValue(deviceId, streamMag, mag_rms); 00097 printf("send() returned %d\r\n", ret); 00098 ret = m2xClient.updateStreamValue(deviceId, streamGyr, gyro_rms); 00099 printf("send() returned %d\r\n", ret); 00100 wait(1); 00101 } 00102 00103 }
Generated on Thu Jul 14 2022 01:01:06 by
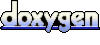