mbed Sensor node for Instrumented Booth over ETH.
Dependencies: EthernetInterface-1 MaxbotixDriver Presence HTU21D_TEMP_HUMID_SENSOR_SAMPLE Resources SHARPIR mbed-rtos mbed-src WDT_K64F nsdl_lib
Fork of Trenton_Switch_LPC1768_ETH by
sensor_ctl.cpp
00001 /** Implements Sensor Control for CES Instrumented Booth */ 00002 00003 #include "mbed.h" 00004 #include "sensor_ctl.h" 00005 #include "node_cfg.h" 00006 00007 //Sensor Drivers 00008 #include "htu21d.h" 00009 #include "MAX9814.h" 00010 #include "sonar.h" 00011 #include "Presence.h" 00012 #include "SHARPIR.h" 00013 #include "DoorTrip.h" 00014 //#include "FXOS8700Q.h" 00015 00016 //Sensor MDS Resources 00017 #include "door_trip.h" 00018 #include "height.h" 00019 #include "presence_resource.h" 00020 #include "sound_level.h" 00021 #include "temperature.h" 00022 #include "accelerometer.h" 00023 00024 00025 //Common Sensors 00026 #if NODE_SENSOR_STATION 00027 htu21d temphumid(PTE25, PTE24); //Temp humid sensor || SDA, SCL 00028 MAX9814 microphone(PTB3); //Analogue in required. 00029 #if NODE_PIR_STATION 00030 presence pir(PTB11, false, PIR_SENSOR_DEBOUNCE_MS); //(InterruptPin), for PIR sensor, 00031 #endif //NODE PIR STATION 00032 #if NODE_KIOSK_STATION 00033 presence kiosk(PTB10, true, KIOSK_SENSOR_DEBOUNCE_MS); //(Interrupt pinrequired, no timeout) 00034 #endif //NODE KIOSK STATION 00035 #if NODE_DOOR_TRIP_STATION 00036 DoorTrip laser(PTC11, true, DOOR_SENSOR_DEBOUNCE_MS ); //(AnalogIn required), for IR door trip 00037 #endif //NODE TRIP STATION 00038 #if NODE_HEIGHT_STATION 00039 Timer sonarTimer; 00040 Timer debounceHeight; 00041 Sonar sonar(PTC10, sonarTimer); //(AnalogIn required, Leave as SW2.) 00042 00043 #define DOOR_HEIGHT_START_MEASURING_THRESHOLD_CM 150 00044 #define DOOR_HEIGHT_STOP_MEASURING_THRESHOLD_CM 140 00045 #define DOOR_HEIGHT_STOP_MEASURING_SAMPLE_COUNT 2 00046 #define DOOR_HEIGHT_SENSOR_MOUNT_HEIGHT_CM 220 00047 #endif //NODE HEIGHT STATION 00048 #if NODE_ACCELEROMETER_STATION 00049 FXOS8700Q_acc acc( PTE25, PTE24, FXOS8700CQ_SLAVE_ADDR1); 00050 #endif //NODE ACCELEROMETER STATION 00051 #endif //NODE_SENSOR_STATION 00052 00053 00054 //Variables provided to rest of applications 00055 float current_temperature_value = 0; 00056 float current_ambient_noise_value = 0; 00057 float current_ambient_noise_sum = 0; 00058 int current_ambient_noise_count = 0; 00059 //Either height XOR kiosk presence XOR PIR station... 00060 float current_height_value = 0; 00061 bool current_presence_value = false; //Either from Kiosk or PIR 00062 //And it might have a door trip.. 00063 bool current_door_trip_value = false; 00064 bool current_accelerometer_value = false; 00065 00066 //Door trip... 00067 float door_trip_starting_volts = 0; 00068 float maxValue=0; 00069 bool set=0; 00070 00071 //Door Height... 00072 float door_height_max_value = 0; 00073 bool door_height_measuring = 0; 00074 int door_height_sample_count = 0; 00075 00076 //Initialisation 00077 void init_sensors() { 00078 // #if NODE_DOOR_TRIP_STATION 00079 // door_trip_starting_volts = sharpir.volt(); 00080 // #endif 00081 #if NODE_PIR_STATION 00082 wait(2); //Wait 2 seconds for sensor calibration period. 00083 #endif //PIR STATION 00084 #if NODE_ACCELEROMETER_STATION 00085 acc.enable(); 00086 #endif 00087 #if NODE_HEIGHT_STATION 00088 sonarTimer.start(); 00089 #endif 00090 } 00091 00092 #if NODE_SENSOR_STATION 00093 00094 //timer handler functions 00095 void handle_temperature_report_timer() { 00096 current_temperature_value = temphumid.getTemp(); 00097 printf("Temperature Sample: %2.2f\r\n", current_temperature_value); 00098 temperature_report(); 00099 } 00100 00101 void handle_microphone_sample_timer(){ 00102 float sample = microphone.sound_level(); 00103 //printf("Sound Sample: %2.2f\r\n", sample); 00104 //Keep running sum and sample count to compute average... 00105 current_ambient_noise_sum += sample; 00106 current_ambient_noise_count++; 00107 current_ambient_noise_value = current_ambient_noise_sum / current_ambient_noise_count; 00108 // if (sample > current_ambient_noise_value){ 00109 // current_ambient_noise_value = sample; 00110 // } 00111 } 00112 00113 void handle_microphone_report_timer(){ 00114 //Report. 00115 sound_level_report(); 00116 //Reset noise... 00117 current_ambient_noise_value = 0; 00118 current_ambient_noise_sum = 0; 00119 current_ambient_noise_count = 0; 00120 } 00121 00122 #if NODE_PIR_STATION 00123 void handle_motion_report_timer(){ 00124 bool new_pir = pir.isPresent(); 00125 //printf("PIR Sample: %d\r\n", new_pir); 00126 //printf("Old PIR Sample: %d\r\n", current_presence_value); 00127 if(new_pir != current_presence_value) { 00128 //printf("Reporting PIR...\r\n"); 00129 current_presence_value = new_pir; 00130 presence_report(); 00131 } 00132 } 00133 #endif //NODE_PIR_STATION 00134 00135 #if NODE_KIOSK_STATION 00136 void handle_kiosk_report_timer(){ 00137 bool new_kiosk = kiosk.isPresent(); 00138 if(new_kiosk != current_presence_value) { 00139 current_presence_value = new_kiosk; 00140 presence_report(); 00141 } 00142 } 00143 #endif //NODE_KIOSK_STATION 00144 00145 #if NODE_DOOR_TRIP_STATION 00146 void handle_door_trip_report_timer(){ 00147 bool new_laser = laser.isPresent(); 00148 00149 if(new_laser != current_door_trip_value) { 00150 current_door_trip_value = new_laser; 00151 door_trip_report(); 00152 } 00153 } 00154 #endif //NODE_DOOR_TRIP_STATION 00155 00156 #if NODE_HEIGHT_STATION 00157 void handle_door_height_sample_timer(){ 00158 int height_sample = DOOR_HEIGHT_SENSOR_MOUNT_HEIGHT_CM-sonar.read(); 00159 if(height_sample > DOOR_HEIGHT_START_MEASURING_THRESHOLD_CM) { 00160 door_height_sample_count=0; 00161 if (height_sample > door_height_max_value) { 00162 door_height_max_value = height_sample; 00163 } 00164 door_height_measuring=true; 00165 } 00166 00167 if((height_sample < DOOR_HEIGHT_STOP_MEASURING_THRESHOLD_CM && door_height_measuring )){ 00168 door_height_sample_count++; 00169 if (door_height_sample_count > DOOR_HEIGHT_STOP_MEASURING_SAMPLE_COUNT){ 00170 current_height_value=door_height_max_value; 00171 door_height_max_value=0,door_height_measuring=false; 00172 door_height_sample_count = 0; 00173 height_report(); 00174 } 00175 } 00176 } 00177 #endif //NODE HEIGHT STATION 00178 00179 #if NODE_ACCELEROMETER_STATION 00180 void handle_accelerometer_report_timer(){ 00181 bool new_accelerometer = acc.get_values(); 00182 if(new_accelerometer != current_accelerometer_value) { 00183 00184 current_accelerometer_value = new_accelerometer; 00185 accelerometer_report(); 00186 } 00187 00188 } 00189 #endif //NODE_ACCELEROMETER_STATION 00190 00191 #endif //NODE_SENSOR_STATION
Generated on Wed Jul 13 2022 06:03:53 by
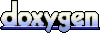