mbed Sensor node for Instrumented Booth over ETH.
Dependencies: EthernetInterface-1 MaxbotixDriver Presence HTU21D_TEMP_HUMID_SENSOR_SAMPLE Resources SHARPIR mbed-rtos mbed-src WDT_K64F nsdl_lib
Fork of Trenton_Switch_LPC1768_ETH by
nsdl_run.cpp
00001 #include "mbed.h" 00002 #include "nsdl_support.h" 00003 #include "nsdl_dbg.h" 00004 00005 #include "node_cfg.h" 00006 00007 #include "door_trip.h" 00008 #include "height.h" 00009 #include "presence_resource.h" 00010 #include "temperature.h" 00011 #include "sound_level.h" 00012 00013 00014 // **************************************************************************** 00015 // Configuration section 00016 00017 // NSP configuration 00018 /* Change this IP address to that of your NanoService Platform installation */ 00019 uint8_t NSP_address_bytes[] = NSP_IP_ADDRESS_BYTES; 00020 00021 uint8_t endpoint_name[30] = NODE_NAME; 00022 uint8_t ep_type[] = ENDPOINT_TYPE; 00023 uint8_t lifetime_ptr[] = LIFE_TIME; 00024 00025 // **************************************************************************** 00026 // Resource creation 00027 00028 static int create_resources() 00029 { 00030 sn_nsdl_resource_info_s *resource_ptr = NULL; 00031 sn_nsdl_ep_parameters_s *endpoint_ptr = NULL; 00032 00033 NSDL_DEBUG("Creating resources"); 00034 00035 /* Create resources */ 00036 resource_ptr = (sn_nsdl_resource_info_s*)nsdl_alloc(sizeof(sn_nsdl_resource_info_s)); 00037 if(!resource_ptr) 00038 return 0; 00039 memset(resource_ptr, 0, sizeof(sn_nsdl_resource_info_s)); 00040 00041 resource_ptr->resource_parameters_ptr = (sn_nsdl_resource_parameters_s*)nsdl_alloc(sizeof(sn_nsdl_resource_parameters_s)); 00042 if(!resource_ptr->resource_parameters_ptr) 00043 { 00044 nsdl_free(resource_ptr); 00045 return 0; 00046 } 00047 memset(resource_ptr->resource_parameters_ptr, 0, sizeof(sn_nsdl_resource_parameters_s)); 00048 00049 // Static resources 00050 nsdl_create_static_resource(resource_ptr, sizeof("dev/mfg")-1, (uint8_t*) "dev/mfg", 0, 0, (uint8_t*) "ARM mbed", sizeof("ARM mbed")-1); 00051 nsdl_create_static_resource(resource_ptr, sizeof("dev/mdl")-1, (uint8_t*) "dev/mdl", 0, 0, (uint8_t*) "Ethernet node", sizeof("Ethernet node")-1); 00052 00053 // Dynamic resources 00054 #if NODE_SENSOR_STATION 00055 create_temperature_resource(resource_ptr); 00056 create_sound_level_resource(resource_ptr); 00057 #if NODE_KIOSK_STATION 00058 create_presence_resource(resource_ptr); 00059 #elif NODE_HEIGHT_STATION 00060 create_height_resource(resource_ptr); 00061 #endif 00062 #if NODE_DOOR_TRIP_STATION 00063 create_door_trip_resource(resource_ptr); 00064 #endif 00065 #if NODE_PIR_STATION 00066 create_presence_resource(resource_ptr); 00067 #endif 00068 #endif 00069 00070 /* Register with NSP */ 00071 // endpoint_ptr = nsdl_init_register_endpoint(endpoint_ptr, (uint8_t*)endpoint_name, ep_type, lifetime_ptr); 00072 // if(sn_nsdl_register_endpoint(endpoint_ptr) != 0) 00073 // printf("NSP registering failed\r\n"); 00074 // else 00075 // printf("NSP registering OK\r\n"); 00076 // nsdl_clean_register_endpoint(&endpoint_ptr); 00077 00078 nsdl_free(resource_ptr->resource_parameters_ptr); 00079 nsdl_free(resource_ptr); 00080 return 1; 00081 } 00082 00083 // **************************************************************************** 00084 // Program entry point 00085 // this modified to startup as an option in the Wi-Go demo 00086 00087 void nsdl_run() 00088 { 00089 00090 NSDL_DEBUG("ARM NSP Init\r\n"); 00091 00092 // Initialize NSDL stack 00093 nsdl_init(); 00094 00095 // Create NSDL resources 00096 create_resources(); 00097 00098 }
Generated on Wed Jul 13 2022 06:03:53 by
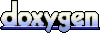