
Code used in the table for the Pong Demo
Dependencies: PixelArray WS2812 mbed
main.cpp
00001 #include "mbed.h" 00002 #include "WS2812.h" 00003 #include "PixelArray.h" 00004 00005 #define WS2812_BUF 112 00006 #define NUM_COLORS 6 00007 #define NUM_LEDS_PER_COLOR 1 00008 00009 //-------- Colours ----------- 00010 #define RED 0x2f0000 00011 #define YELLOW 0x2f2f00 00012 #define GREEN 0x002f00 00013 #define LIGHTBLUE 0x002f2f 00014 #define DARKBLUE 0x00002f 00015 #define BLUE 0x0000ff // Player scored a goal 00016 #define PINK 0x2f002f 00017 #define OFF 0x000000 00018 #define WHITE 0xffffaa 00019 #define ARMBLUE 0x128BAB 00020 #define PURPLE 0xff0055 // Player has conceded a goal 00021 00022 // GPIO 00023 AnalogIn robotBreakBeam(A0); 00024 AnalogIn playerBreakBeam(A1); 00025 DigitalOut led_green(LED_GREEN, 1); 00026 00027 // SERIAL 00028 Serial pc(USBTX, USBRX); // tx, rx 00029 00030 // LED STRIPS 00031 // See the program page for information on the timing numbers 00032 // The given numbers are for the K64F 00033 WS2812 robotScoreLED(D9, WS2812_BUF, 0, 5, 5, 0); 00034 WS2812 playerScoreLED(D8,WS2812_BUF, 0, 5, 5, 0); 00035 WS2812 robotGoalLED(D5,WS2812_BUF, 0, 5, 5, 0); 00036 WS2812 playerGoalLED(D3,WS2812_BUF, 0, 5, 5, 0); 00037 00038 // LED Variables 00039 bool seg1A, seg1B, seg1C, seg1D, seg1E, seg1F, seg1G; 00040 int seg1Array[112]; 00041 int mainArray[11][122]; 00042 PixelArray robotScorePx(WS2812_BUF); 00043 PixelArray playerScorePx(WS2812_BUF); 00044 PixelArray robotGoalPx(WS2812_BUF); 00045 PixelArray playerGoalPx(WS2812_BUF); 00046 const int PLAYERGOALLEDS = 55; 00047 const int ROBOTGOALLEDS = 55; 00048 00049 // Score counters 00050 int robotScore; 00051 int playerScore; 00052 int scoreLimit = 3; 00053 bool finishedGame = false; 00054 int endFlashes = 3; 00055 int numFlashes; 00056 00057 // Robot Bream Beam value 00058 double prevRbbValue; // Previous Robot break beam value 00059 double prevPbbValue; // Previous player break beam value 00060 00061 // FUNCTION DECLERATIONS 00062 void Write7Seg(int num); 00063 void SetLEDArray(int x); 00064 void WriteRobotGoal(bool scored); 00065 void WritePlayerGoal(bool scored); 00066 void WriteScores(); 00067 void HandleGoal(bool hasRobotScored); 00068 void WritePxScores(int line_num, bool isRobot); 00069 void WritePxGoal(unsigned int colour,bool isRobot); 00070 void HandleWin(); 00071 void WriteGoalsOff(); 00072 00073 00074 void setup() 00075 { 00076 00077 // Turn on green LED 00078 led_green = 0; 00079 00080 // Set brightness of the 4 LED strips 00081 robotScoreLED.setII(0x19); 00082 robotScoreLED.useII(WS2812::GLOBAL); 00083 playerScoreLED.setII(0x19); 00084 playerScoreLED.useII(WS2812::GLOBAL); 00085 robotGoalLED.setII(0x19); 00086 robotGoalLED.useII(WS2812::GLOBAL); 00087 playerGoalLED.setII(0x80); 00088 playerGoalLED.useII(WS2812::GLOBAL); 00089 00090 // Fills 2D array with data 00091 for(int i=0; i<10; i++) { 00092 Write7Seg(i); 00093 } 00094 00095 // Set scores to 0 00096 robotScore = 0; 00097 playerScore = 0; 00098 numFlashes = 0; 00099 00100 // Set LEDS to start values 00101 WriteScores(); 00102 wait(0.01); 00103 WritePlayerGoal(true); 00104 wait(0.01); 00105 WriteRobotGoal(true); 00106 00107 wait(5); 00108 00109 WriteGoalsOff(); 00110 // Turn off green LED 00111 led_green = 1; 00112 } 00113 00114 // Set segment variables 00115 void Write7Seg(int num) 00116 { 00117 switch (num) { 00118 case 0 : 00119 // Off 00120 seg1A = 1; 00121 seg1B = 1; 00122 seg1C = 1; 00123 seg1D = 1; 00124 seg1E = 1; 00125 seg1F = 1; 00126 seg1G = 0; 00127 00128 SetLEDArray(0); 00129 00130 break; 00131 00132 case 1 : 00133 // 1 00134 seg1A = 0; 00135 seg1B = 1; 00136 seg1C = 1; 00137 seg1D = 0; 00138 seg1E = 0; 00139 seg1F = 0; 00140 seg1G = 0; 00141 00142 SetLEDArray(1); 00143 break; 00144 00145 case 2 : 00146 // 2 00147 seg1A = 1; 00148 seg1B = 1; 00149 seg1C = 0; 00150 seg1D = 1; 00151 seg1E = 1; 00152 seg1F = 0; 00153 seg1G = 1; 00154 00155 SetLEDArray(2); 00156 00157 break; 00158 00159 case 3 : 00160 // 3 00161 seg1A = 1; 00162 seg1B = 1; 00163 seg1C = 1; 00164 seg1D = 1; 00165 seg1E = 0; 00166 seg1F = 0; 00167 seg1G = 1; 00168 00169 SetLEDArray(3); 00170 break; 00171 00172 case 4: 00173 // 4 00174 seg1A = 0; 00175 seg1B = 1; 00176 seg1C = 1; 00177 seg1D = 0; 00178 seg1E = 0; 00179 seg1F = 1; 00180 seg1G = 1; 00181 00182 SetLEDArray(4); 00183 00184 break; 00185 00186 case 5: 00187 // 5 00188 seg1A = 1; 00189 seg1B = 0; 00190 seg1C = 1; 00191 seg1D = 1; 00192 seg1E = 0; 00193 seg1F = 1; 00194 seg1G = 1; 00195 00196 SetLEDArray(5); 00197 00198 break; 00199 00200 case 6: 00201 // 6 00202 seg1A = 1; 00203 seg1B = 0; 00204 seg1C = 1; 00205 seg1D = 1; 00206 seg1E = 1; 00207 seg1F = 1; 00208 seg1G = 1; 00209 00210 SetLEDArray(6); 00211 00212 break; 00213 00214 case 7: 00215 // 7 00216 seg1A = 1; 00217 seg1B = 1; 00218 seg1C = 1; 00219 seg1D = 0; 00220 seg1E = 0; 00221 seg1F = 0; 00222 seg1G = 0; 00223 00224 SetLEDArray(7); 00225 00226 break; 00227 00228 case 8: 00229 // 8 00230 seg1A = 1; 00231 seg1B = 1; 00232 seg1C = 1; 00233 seg1D = 1; 00234 seg1E = 1; 00235 seg1F = 1; 00236 seg1G = 1; 00237 00238 SetLEDArray(8); 00239 break; 00240 00241 case 9: 00242 // 9 00243 seg1A = 1; 00244 seg1B = 1; 00245 seg1C = 1; 00246 seg1D = 0; 00247 seg1E = 0; 00248 seg1F = 1; 00249 seg1G = 1; 00250 00251 SetLEDArray(9); 00252 break; 00253 00254 case 10: 00255 // OFF 00256 seg1A = 0; 00257 seg1B = 0; 00258 seg1C = 0; 00259 seg1D = 0; 00260 seg1E = 0; 00261 seg1F = 0; 00262 seg1G = 0; 00263 00264 SetLEDArray(10); 00265 break; 00266 00267 default : 00268 00269 break; 00270 00271 } 00272 } 00273 00274 // Write segment config to main array 00275 void SetLEDArray(int x) 00276 { 00277 for (int i = 0; i < WS2812_BUF; i++) { 00278 if (i < 16) { 00279 mainArray[x][i] = seg1A; 00280 } 00281 00282 if ( i >= 16 && i < 32) { 00283 mainArray[x][i] = seg1B; 00284 } 00285 00286 if (i >= 32 && i < 48) { 00287 mainArray[x][i] = seg1C; 00288 } 00289 00290 if (i >= 48 && i < 64) { 00291 mainArray[x][i]= seg1D; 00292 } 00293 00294 if ( i >= 64 && i < 80) { 00295 mainArray[x][i] = seg1E; 00296 } 00297 00298 if (i >= 80 && i < 96) { 00299 mainArray[x][i] = seg1F; 00300 } 00301 00302 if ( i >= 96 && i < 112) { 00303 mainArray[x][i] = seg1G; 00304 } 00305 }// FOR LOOP 00306 } 00307 00308 // Write to player 1 LED (ROBOT) 00309 void WriteRobotGoal(bool scored) 00310 { 00311 if(scored == true) { 00312 WritePxGoal(BLUE,true); 00313 } else { 00314 WritePxGoal(PURPLE,true); 00315 } 00316 robotGoalLED.write(robotGoalPx.getBuf()); 00317 } 00318 00319 // Write to player 1 LED (ROBOT) 00320 void WritePlayerGoal(bool scored) 00321 { 00322 if(scored == true) { 00323 WritePxGoal(BLUE,false); 00324 } else { 00325 WritePxGoal(PURPLE,false); 00326 } 00327 playerGoalLED.write(playerGoalPx.getBuf()); 00328 } 00329 00330 // Write OFF to both goals 00331 void WriteGoalsOff() 00332 { 00333 WritePxGoal(OFF,true); 00334 robotGoalLED.write(robotGoalPx.getBuf()); 00335 WritePxGoal(OFF,false); 00336 playerGoalLED.write(playerGoalPx.getBuf()); 00337 } 00338 00339 // Update both Score LEDs with the current score 00340 void WriteScores() 00341 { 00342 WritePxScores(playerScore,false); 00343 playerScoreLED.write(playerScorePx.getBuf()); 00344 wait(0.01); 00345 WritePxScores(robotScore,true); 00346 robotScoreLED.write(robotScorePx.getBuf()); 00347 } 00348 00349 // Write pixel array 00350 void WritePxScores(int line_num,bool isRobot) 00351 { 00352 if(isRobot == true) { 00353 00354 for (int i = 0; i < WS2812_BUF; i++) { 00355 if (mainArray[line_num][i] == 0) { 00356 robotScorePx.Set(i,OFF); 00357 } 00358 00359 if (mainArray[line_num][i] == 1) { 00360 robotScorePx.Set(i,WHITE); 00361 } 00362 } 00363 } else { 00364 for (int i = 0; i < WS2812_BUF; i++) { 00365 if (mainArray[line_num][i] == 0) { 00366 playerScorePx.Set(i,OFF); 00367 } 00368 00369 if (mainArray[line_num][i] == 1) { 00370 playerScorePx.Set(i,WHITE); 00371 } 00372 } 00373 } 00374 00375 } 00376 00377 // Write Goal pixel array, using input colour for the specified goal 00378 // INPUTS: colour, isRobot 00379 // 00380 // colour - This is the colour that you wish to set the array to 00381 // isRobot - This specifies which goal to set the colours of, based on if it is the Robot side goal or not 00382 // 00383 void WritePxGoal(unsigned int colour,bool isRobot) 00384 { 00385 if(isRobot == true) { 00386 for (int i = 0; i < WS2812_BUF; i++) { 00387 robotGoalPx.Set(i,colour); 00388 } 00389 } else { 00390 for (int i = 0; i < WS2812_BUF; i++) { 00391 playerGoalPx.Set(i,colour); 00392 } 00393 } 00394 } 00395 00396 // Write the goal LEDs and score LEDs when a goal is recorded. Also check to see if the goal being scored 00397 // is the final point of the game and the game is over. 00398 // INPUTS: hasRobotScored 00399 // 00400 // hasRobotScored - true if the goal being recorded is from the playerside goal, meaning the robot has scored a point 00401 // 00402 void HandleGoal(bool hasRobotScored) 00403 { 00404 if(hasRobotScored == true) { 00405 robotScore++; 00406 WriteRobotGoal(true); 00407 wait(0.01); 00408 WritePlayerGoal(false); 00409 wait(0.01); 00410 WriteScores(); 00411 } else { 00412 playerScore++; 00413 WriteRobotGoal(false); 00414 wait(0.01); 00415 WritePlayerGoal(true); 00416 wait(0.01); 00417 WriteScores(); 00418 } 00419 00420 wait(5); 00421 00422 // If either player reaches the score limit, then call HandleWin(). If not, then turn goal LEDs off 00423 if(robotScore == scoreLimit || playerScore == scoreLimit) { 00424 HandleWin(); 00425 } else { 00426 WriteGoalsOff(); 00427 } 00428 } 00429 00430 // Handle behaviour when either the player or robot has won a game. 00431 void HandleWin() 00432 { 00433 // Initalise variable as true 00434 bool isRobotWinner = true; 00435 00436 // Decide if the robot is the winner. If not, then change isRobotWinner to false 00437 if(playerScore == scoreLimit) { 00438 isRobotWinner = false; 00439 pc.printf("Player won!"); 00440 00441 // Animation for goal LEDs. Turns off the robot Goal LEDs, working from in the middle point outwards. 00442 for(int i = 0; i < (ROBOTGOALLEDS / 2); i++) { 00443 robotGoalPx.Set((ROBOTGOALLEDS /2) - i, OFF); 00444 robotGoalPx.Set((ROBOTGOALLEDS /2) + i, OFF); 00445 robotGoalLED.write(robotGoalPx.getBuf()); 00446 wait(0.15); 00447 } 00448 00449 WriteGoalsOff(); 00450 00451 } else { 00452 pc.printf("Robot won!"); 00453 00454 // Animation for goal LEDs. Turns off the player Goal LEDs, working from in the middle point outwards. 00455 for(int i = 0; i < (PLAYERGOALLEDS / 2); i++) { 00456 playerGoalPx.Set((PLAYERGOALLEDS /2) - i, OFF); 00457 playerGoalPx.Set((PLAYERGOALLEDS /2) + i, OFF); 00458 playerGoalLED.write(playerGoalPx.getBuf()); 00459 wait(0.25); 00460 } 00461 00462 WriteGoalsOff(); 00463 } 00464 00465 // Reset scores then write to LEDs 00466 robotScore = 0; 00467 playerScore = 0; 00468 WriteScores(); 00469 } 00470 00471 int main() 00472 { 00473 setup(); 00474 00475 while(1) { 00476 double rbbValue = robotBreakBeam; // Read Robot Break beam 00477 double pbbValue = playerBreakBeam; // Read Player Break beam 00478 00479 // IF PLAYER HAS SCORED A GOAL 00480 if ((prevRbbValue - rbbValue)>0.03) { 00481 pc.printf("Player has scored a goal \n\r"); 00482 HandleGoal(false); 00483 } 00484 00485 // IF ROBOT HAS SCORED A GOAL 00486 if ((prevPbbValue - pbbValue) > 0.03) { 00487 pc.printf("Robot has scored a goal \n\r"); 00488 HandleGoal(true); 00489 } 00490 00491 prevRbbValue = rbbValue; 00492 prevPbbValue = pbbValue; 00493 00494 pc.printf("PlayerGoal: %f, RobotGoal: %f \r\n",pbbValue,rbbValue); 00495 pc.printf("Player: %i v %i : Robot \r\n",playerScore,robotScore); 00496 wait(0.02); 00497 00498 } 00499 }
Generated on Mon Jul 25 2022 03:50:42 by
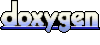