
updated 7seg controls for new 7 seg boards
Dependencies: PixelArray WS2812 mbed
Fork of frdm_pong_table_controller by
main.h
00001 #ifndef MAIN_H 00002 #define MAIN_H 00003 00004 #include "mbed.h" 00005 #include "WS2812.h" 00006 #include "PixelArray.h" 00007 #include "PinDetect.h" 00008 00009 #define WS2812_BUF 122 00010 #define NUM_COLORS 6 00011 #define NUM_LEDS_PER_COLOR 1 00012 00013 //-------- Colours ----------- 00014 #define RED 0x2f0000 00015 #define YELLOW 0x2f2f00 00016 #define GREEN 0x002f00 00017 #define LIGHTBLUE 0x002f2f 00018 #define DARKBLUE 0x00002f 00019 #define BLUE 0x0000ff // Player scored a goal 00020 #define PINK 0x2f002f 00021 #define OFF 0x000000 00022 #define WHITE 0xffffaa 00023 #define ARMBLUE 0x128BAB 00024 #define PURPLE 0xff0055 // Player has conceded a goal 00025 00026 // Goal Sensors 00027 AnalogIn robotBreakBeam(A0); 00028 AnalogIn playerBreakBeam(A1); 00029 00030 // K64F On-board LED 00031 DigitalOut led_green(LED_GREEN, 1); 00032 00033 //K64F On-board Switches 00034 DigitalIn PB1(PTC6); 00035 DigitalIn PB2(PTA4); 00036 PinDetect idleButton(PTC11); 00037 00038 // SERIAL 00039 RawSerial pc(USBTX, USBRX); // tx, rx 00040 00041 // LED STRIPS 00042 // See the program page for information on the timing numbers 00043 // The given numbers are for the K64F 00044 WS2812 robotScoreLED(D3, WS2812_BUF, 0, 5, 5, 0); 00045 WS2812 playerScoreLED(D5,WS2812_BUF, 0, 5, 5, 0); 00046 PixelArray robotScorePx(WS2812_BUF); 00047 PixelArray playerScorePx(WS2812_BUF); 00048 00049 00050 // LED Variables 00051 bool seg1A, seg1B, seg1C, seg1D, seg1E, seg1F, seg1G; 00052 int mainArray[11][122]; 00053 int rand_colors[] = {0x00FF00, 0x7FFF00, 0xFFFF00, 0xFF7F00, 0xFF0000, 0xFE00FF, 0x7F00FF, 0x0000FF, 0x007FFF, 0x00FFFE, 0x00FF7F}; 00054 00055 // Score counters 00056 int robotScore; 00057 int playerScore; 00058 int scoreLimit = 3; 00059 bool finishedGame = false; 00060 int endFlashes = 3; 00061 int numFlashes; 00062 00063 // Flags 00064 volatile int idle_flag = 0; 00065 volatile int idle_button_pressed = 0; 00066 volatile int previous_state = 0; 00067 00068 // Robot Bream Beam value 00069 double prevRbbValue; // Previous Robot break beam value 00070 double prevPbbValue; // Previous player break beam value 00071 00072 // FUNCTION DECLERATIONS 00073 void Setup(); 00074 void SetNumberPatterns(); // sets segment patterns for numbers 00075 void SetLEDArray(int x); // sets segment patterns in mainArray 00076 void WriteScores(); // writes scores to the LEDs 00077 void WritePxAnimation(int line_num,bool isRobot,bool colour); // writes mainArray to either LED buffer in blue or random colours 00078 00079 // Protocols for handling goals and whether the player or robot has won 00080 void HandleGoal(bool hasRobotScored); 00081 void HandleWin(); 00082 00083 // Animation for scoring a goal and winning the game 00084 void GoalAnimation(bool hasRobotScored); 00085 void WinAnimation(bool isRobotWinner); 00086 00087 // Decorative Animations 00088 void CircleAnimation(bool robot, bool robotColour,bool player, bool playerColour, int numberOfRepitions); 00089 void FigureOf8Animation(bool robot, bool robotColour,bool player, bool playerColour, int numberOfRepitions); 00090 void DrainAnimation(bool robot, bool robotColour, bool player, bool playerColour); 00091 00092 // Animations for writing and deleting displayed numbers 00093 void ZeroInAnimation(bool robot, bool robotColour,bool player, bool playerColour); 00094 void ZeroOutAnimation(bool robot, bool robotColour,bool player, bool playerColour); 00095 void OneInAnimation(bool robot, bool robotColour,bool player, bool playerColour); 00096 void OneOutAnimation(bool robot, bool robotColour,bool player, bool playerColour); 00097 void TwoInAnimation(bool robot, bool robotColour,bool player, bool playerColour); 00098 void TwoOutAnimation(bool robot, bool robotColour,bool player, bool playerColour); 00099 void ThreeInAnimation(bool robot, bool robotColour,bool player, bool playerColour); 00100 void ThreeOutAnimation(bool robot, bool robotColour,bool player, bool playerColour); 00101 00102 // Protocol and transition functions for Idle and Play states 00103 void IdleStateProtocol(); 00104 void PlayStateProtocol(); 00105 void PlayToIdleTransition(); 00106 void IdleToPlayTransition(); 00107 00108 // ISRs 00109 void idleButtonISR(); 00110 00111 00112 #endif
Generated on Thu Jul 14 2022 07:25:28 by
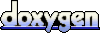