
Test serial console demonstrating various API functions of WiConnect library.
Embed:
(wiki syntax)
Show/hide line numbers
TcpEchoTest.cpp
00001 /** 00002 * ACKme WiConnect Host Library is licensed under the BSD licence: 00003 * 00004 * Copyright (c)2014 ACKme Networks. 00005 * All rights reserved. 00006 * 00007 * Redistribution and use in source and binary forms, with or without modification, 00008 * are permitted provided that the following conditions are met: 00009 * 00010 * 1. Redistributions of source code must retain the above copyright notice, 00011 * this list of conditions and the following disclaimer. 00012 * 2. Redistributions in binary form must reproduce the above copyright notice, 00013 * this list of conditions and the following disclaimer in the documentation 00014 * and/or other materials provided with the distribution. 00015 * 3. The name of the author may not be used to endorse or promote products 00016 * derived from this software without specific prior written permission. 00017 * 00018 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS AND ANY EXPRESS OR IMPLIED 00019 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00020 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00021 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00022 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00023 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00024 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00025 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00026 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00027 * OF SUCH DAMAGE. 00028 */ 00029 00030 #include "tests/Tests.h" 00031 #include "Wiconnect.h" 00032 00033 00034 00035 static WiconnectResult processData(WiconnectSocket &socket, uint32_t count, uint32_t delay, uint32_t size); 00036 00037 00038 00039 /*************************************************************************************************/ 00040 WiconnectResult socketTcpClientEchoCommand(int argc, char **argv) 00041 { 00042 WiconnectResult result; 00043 Wiconnect *wiconnect = Wiconnect::getInstance(); 00044 WiconnectSocket socket(TEST_BUFFER_LENGTH/2, TEST_BUFFER, TEST_BUFFER_LENGTH/2, TEST_BUFFER + TEST_BUFFER_LENGTH/2); 00045 const char *host; 00046 uint32_t port; 00047 uint32_t delay = 5; 00048 uint32_t count = 1000; 00049 uint32_t size = 256; 00050 00051 if(argc < 2) 00052 { 00053 LOG_ERROR("Must specify host and port"); 00054 return WICONNECT_BAD_ARG; 00055 } 00056 host = argv[0]; 00057 00058 if(!StringUtil::strToUint32((const char*)argv[1], &port)) 00059 { 00060 LOG_ERROR("Invalid port"); 00061 return WICONNECT_BAD_ARG; 00062 } 00063 if(argc > 2 && !StringUtil::strToUint32((const char*)argv[2], &count)) 00064 { 00065 LOG_ERROR("Invalid packet count"); 00066 return WICONNECT_BAD_ARG; 00067 } 00068 if(argc > 3 && !StringUtil::strToUint32((const char*)argv[3], &delay)) 00069 { 00070 LOG_ERROR("Invalid packet delay"); 00071 return WICONNECT_BAD_ARG; 00072 } 00073 if(argc > 4 && (!StringUtil::strToUint32((const char*)argv[4], &size) || size > sizeof(testBuffer)/2)) 00074 { 00075 LOG_ERROR("Invalid packet size"); 00076 return WICONNECT_BAD_ARG; 00077 } 00078 00079 if(WICONNECT_FAILED(result, wiconnect->tcpConnect(socket, host, port))) 00080 { 00081 LOG_ERROR("Failed to connect to server"); 00082 } 00083 else 00084 { 00085 result = processData(socket, count, delay, size); 00086 } 00087 00088 return result; 00089 } 00090 00091 00092 /*************************************************************************************************/ 00093 static WiconnectResult processData(WiconnectSocket &socket, uint32_t count, uint32_t delay, uint32_t size) 00094 { 00095 WiconnectResult result = WICONNECT_ERROR; 00096 uint8_t *txBuffer = socket.getTxBuffer(); 00097 const int txBufferLen = socket.getTxBufferSize(); 00098 uint8_t writeCounter = 32; 00099 uint8_t readCounter = 32; 00100 uint32_t bytesSent = 0; 00101 uint32_t bytesReceived = 0; 00102 00103 LOG_INFO("\r\n------------------\r\n" 00104 "Starting TCP Echo Test:\r\n" 00105 "Packet count: %d\r\n" 00106 "Packet size: %d\r\n" 00107 "Packet delay: %d\r\n" 00108 "(Press any key to terminate)", 00109 count, size, delay); 00110 00111 for(int i = 0; i < count || bytesReceived < bytesSent; ++i) 00112 { 00113 if(i < count) 00114 { 00115 uint8_t *ptr = txBuffer; 00116 int l = size; 00117 while(l--) 00118 { 00119 *ptr++ = writeCounter++; 00120 if(writeCounter == 128) 00121 writeCounter = 32; 00122 } 00123 00124 if(WICONNECT_FAILED(result, socket.write(size))) 00125 { 00126 break; 00127 } 00128 bytesSent += size; 00129 consoleSerial.putc('.'); 00130 } 00131 00132 if(consoleSerial.readable()) 00133 { 00134 int c = consoleSerial.getc(); 00135 break; 00136 } 00137 00138 delayMs(delay); 00139 00140 uint8_t *readPtr; 00141 uint16_t readLen; 00142 if(WICONNECT_FAILED(result, socket.read(&readPtr, &readLen))) 00143 { 00144 break; 00145 } 00146 bytesReceived += readLen; 00147 00148 while(readLen--) 00149 { 00150 if(*readPtr != readCounter) 00151 { 00152 LOG_ERROR("Invalid: %02X != %02X", *readPtr, readCounter); 00153 } 00154 ++readPtr; 00155 ++readCounter; 00156 if(readCounter == 128) 00157 readCounter = 32; 00158 } 00159 } 00160 00161 consoleSerial.write("\r\n"); 00162 LOG_INFO("------------------\r\n" 00163 "TCP Echo Test Complete:\r\n" 00164 "Bytes sent: %d\r\n" 00165 "Bytes received: %d\r\n", 00166 bytesSent, bytesReceived); 00167 00168 return result; 00169 }
Generated on Thu Jul 14 2022 08:17:33 by
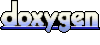