
Test serial console demonstrating various API functions of WiConnect library.
Embed:
(wiki syntax)
Show/hide line numbers
HttpGetTest.cpp
00001 /** 00002 * ACKme WiConnect Host Library is licensed under the BSD licence: 00003 * 00004 * Copyright (c)2014 ACKme Networks. 00005 * All rights reserved. 00006 * 00007 * Redistribution and use in source and binary forms, with or without modification, 00008 * are permitted provided that the following conditions are met: 00009 * 00010 * 1. Redistributions of source code must retain the above copyright notice, 00011 * this list of conditions and the following disclaimer. 00012 * 2. Redistributions in binary form must reproduce the above copyright notice, 00013 * this list of conditions and the following disclaimer in the documentation 00014 * and/or other materials provided with the distribution. 00015 * 3. The name of the author may not be used to endorse or promote products 00016 * derived from this software without specific prior written permission. 00017 * 00018 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS AND ANY EXPRESS OR IMPLIED 00019 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00020 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00021 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00022 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00023 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00024 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00025 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00026 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00027 * OF SUCH DAMAGE. 00028 */ 00029 00030 #include "tests/Tests.h" 00031 #include "Wiconnect.h" 00032 00033 00034 00035 static WiconnectResult addHeadersPrompt(Wiconnect *wiconnect, WiconnectSocket &socket); 00036 static WiconnectResult readResponse(WiconnectSocket &socket); 00037 00038 00039 00040 /*************************************************************************************************/ 00041 WiconnectResult socketHttpGetCommand(int argc, char **argv) 00042 { 00043 WiconnectResult result; 00044 Wiconnect *wiconnect = Wiconnect::getInstance(); 00045 WiconnectSocket socket(TEST_BUFFER_LENGTH, TEST_BUFFER); 00046 const char *url; 00047 bool addHeaders = false; 00048 int savedTimeout = wiconnect->getCommandDefaultTimeout(); 00049 00050 if(argc < 1) 00051 { 00052 LOG_ERROR("must specify url"); 00053 return WICONNECT_BAD_ARG; 00054 } 00055 url = argv[0]; 00056 00057 if(argc > 1) 00058 { 00059 addHeaders = true; 00060 } 00061 00062 wiconnect->setCommandDefaultTimeout(15000); 00063 if(!WICONNECT_FAILED(result, wiconnect->httpGet(socket, url, addHeaders))) 00064 { 00065 uint32_t status; 00066 if(addHeaders) 00067 { 00068 if(WICONNECT_FAILED(result, addHeadersPrompt(wiconnect, socket))) 00069 { 00070 goto exit; 00071 } 00072 else if(WICONNECT_FAILED(result, wiconnect->httpGetStatus(socket, &status))) 00073 { 00074 goto exit; 00075 } 00076 LOG_INFO("HTTP Status: %d", status); 00077 } 00078 00079 result = readResponse(socket); 00080 } 00081 00082 exit: 00083 wiconnect->setCommandDefaultTimeout(savedTimeout); 00084 00085 return result; 00086 } 00087 00088 00089 00090 00091 /*************************************************************************************************/ 00092 static WiconnectResult addHeadersPrompt(Wiconnect *wiconnect, WiconnectSocket &socket) 00093 { 00094 WiconnectResult result; 00095 00096 char buffer[128]; 00097 for(;;) 00098 { 00099 LOG_INFO("Enter header 'key,value\\n' (or 'done\\n' to issue request):"); 00100 char *ptr = buffer; 00101 00102 for(;;) 00103 { 00104 int c = consoleSerial.getc(); 00105 consoleSerial.putc(c); 00106 if(c == '\r') 00107 continue; 00108 if(c == '\n') 00109 { 00110 *ptr = 0; 00111 break; 00112 } 00113 *ptr++ = (char)c; 00114 } 00115 00116 if(strcmp(buffer, "done") == 0) 00117 { 00118 return WICONNECT_SUCCESS; 00119 } 00120 00121 char *value = strchr(buffer, ','); 00122 if(value == NULL) 00123 { 00124 LOG_ERROR("Mal-formed key,value pair. Must be: <key>,<value>"); 00125 continue; 00126 } 00127 00128 *value++ = 0; 00129 00130 if(WICONNECT_FAILED(result, wiconnect->httpAddHeader(socket, buffer, value))) 00131 { 00132 LOG_ERROR("Failed to add header key, value"); 00133 break; 00134 } 00135 } 00136 00137 return result; 00138 } 00139 00140 /*************************************************************************************************/ 00141 static WiconnectResult readResponse(WiconnectSocket &socket) 00142 { 00143 uint8_t c; 00144 00145 LOG_INFO("Response data:"); 00146 while(socket.getc(&c) == WICONNECT_SUCCESS) // NOTE: getc() is extremely inefficient here, 00147 // is only used to test its functionality 00148 { 00149 logWrite(&c, 1); 00150 } 00151 00152 return WICONNECT_SUCCESS; 00153 }
Generated on Thu Jul 14 2022 08:17:33 by
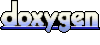