
Example application demonstrating the TCP server API of the WiConnect Host Library
Embed:
(wiki syntax)
Show/hide line numbers
example.cpp
00001 /** 00002 * @example tcp_server/example.cpp 00003 * 00004 * This is an example of using the TCP server API to 00005 * send/receive data from a remote TCP client. 00006 * 00007 * This example is intended to be used with 'tcp_client.py' 00008 * python script in the same directory as this example.cpp file. 00009 * 00010 * It works as follows: 00011 * 1. Instantiate the WiConnect Library 00012 * 2. Initiate Communication with WiFi Module 00013 * 3. Join the network 00014 * 4. Start the TCP server 00015 * 5. Wait for clients to connect 00016 * 6. Receive data from client 00017 * 7. Send data to client 00018 * 8. Close client connection 00019 * 9. Goto sleep 5 00020 * 00021 */ 00022 00023 00024 /****************************************************************************** 00025 * Example Variables 00026 */ 00027 00028 // The port the server listens on 00029 #define TCP_SERVER_PORT 7 00030 // The maximum simultaneous client connections 00031 // (note this example only supports 1) 00032 #define TCP_SERVER_MAX_CLIENTS 1 00033 00034 // This is the name of your WiFi network 00035 // Look for this name in your WiFi settings 00036 // (e.g. your phone's list of WiFi networks in the WiFi settings menu) 00037 // tip: add double-quotes around SSID to add spaces to name 00038 #define NETWORK_SSID "\"<YOUR NETWORK NAME HERE>\"" 00039 00040 // This is the password of your WiFi network 00041 // Leave as empty string (e.g "") to connect to OPEN network 00042 #define NETWORK_PASSWORD "\"<YOUR NETWORK PASSWORD HERE>\"" 00043 00044 00045 /****************************************************************************** 00046 * Includes 00047 */ 00048 00049 // include C library headers 00050 #include <stdio.h> // needed for printf 00051 00052 // include target specific defines 00053 #include "target_config.h" 00054 // include the Wiconnect Host Library API header 00055 #include "Wiconnect.h" 00056 00057 00058 00059 /****************************************************************************** 00060 * Local Functions 00061 */ 00062 00063 00064 /****************************************************************************** 00065 * Global Defines 00066 */ 00067 00068 // Transmit/receive buffers for the remote client socket 00069 static uint8_t clientRxBuffer[256], clientTxBuffer[256]; 00070 00071 // Serial used for printfs to terminal (i.e. NOT used for WiConnect) 00072 static Serial consoleSerial(STDIO_UART_TX, STDIO_UART_RX); 00073 00074 00075 00076 //------------------------------------------------------------------------- 00077 // STEP 1: Instantiate WiConnect Library 00078 //------------------------------------------------------------------------- 00079 00080 00081 // Setup wiconnect serial interface configuration 00082 // Here we only specify the rx buffer size and not rx buffer pointer, this means 00083 // The serial RX buffer will be dynamically allocated 00084 SerialConfig serialConfig(WICONNECT_RX_PIN, WICONNECT_TX_PIN, 256, NULL); 00085 00086 // Instantiate the Wiconnect library 00087 // Here we specify the buffer size ONLY which means we're using dynmaic allocation 00088 Wiconnect wiconnectIfc(serialConfig, 256, NULL, WICONNECT_RESET_PIN); 00089 00090 00091 00092 00093 00094 /****************************************************************************** 00095 * Starting point of application 00096 */ 00097 int main(int argc, char **argv) 00098 { 00099 WiconnectResult result; 00100 // Instantiate a client socket object with statically allocaed transmit/receive buffers 00101 // Note: this socket object isn't valid until tcpAccept() is called with in 00102 WiconnectSocket clientSocket(sizeof(clientRxBuffer), clientRxBuffer, sizeof(clientTxBuffer), clientTxBuffer); 00103 00104 consoleSerial.baud(115200); // console terminal to 115200 baud 00105 00106 00107 //------------------------------------------------------------------------- 00108 // STEP 2: Initiate Communication with WiFi Module 00109 //------------------------------------------------------------------------- 00110 00111 printf("Initializing WiConnect Library...\r\n"); 00112 00113 // Initialize communication with WiFi module 00114 if(WICONNECT_FAILED(result, wiconnectIfc.init(true))) 00115 { 00116 printf("Failed to initialize communication with WiFi module: %s\r\n" 00117 "Make sure the wires are connected correctly\r\n", Wiconnect::getWiconnectResultStr(result)); 00118 if(result == WICONNECT_FIRMWARE_OUTDATED) 00119 { 00120 printf("** The WiFi firmware is not supported. Run the ota example to update the firmware:\r\n"); 00121 printf("https://developer.mbed.org/teams/ACKme/code/wiconnect-ota_example\r\n\r\n"); 00122 } 00123 for(;;); // infinite loop 00124 } 00125 00126 //------------------------------------------------------------------------- 00127 // STEP 3: Join the network 00128 //------------------------------------------------------------------------- 00129 00130 printf("Joining WiFi network: %s\r\n", NETWORK_SSID); 00131 00132 // Initialize communication with WiFi module 00133 if(WICONNECT_FAILED(result, wiconnectIfc.join(NETWORK_SSID, NETWORK_PASSWORD))) 00134 { 00135 printf("Failed to join network: %s\r\n", Wiconnect::getWiconnectResultStr(result)); 00136 for(;;); // infinite loop 00137 } 00138 00139 00140 //------------------------------------------------------------------------- 00141 // STEP 4: Start the TCP server 00142 //------------------------------------------------------------------------- 00143 00144 printf("Starting TCP server, listening on: %s:%d\r\n", wiconnectIfc.getIpAddress(), TCP_SERVER_PORT); 00145 00146 if(WICONNECT_FAILED(result, wiconnectIfc.tcpListen(TCP_SERVER_PORT, TCP_SERVER_MAX_CLIENTS))) 00147 { 00148 printf("Failed to start TCP server: %s\r\n", Wiconnect::getWiconnectResultStr(result)); 00149 for(;;); // infinite loop 00150 } 00151 00152 00153 for(;;) 00154 { 00155 //------------------------------------------------------------------------- 00156 // STEP 5: Wait for clients to connect 00157 //------------------------------------------------------------------------- 00158 00159 printf("Waiting for a client to connect...\r\n"); 00160 00161 if(WICONNECT_FAILED(result, wiconnectIfc.tcpAccept(clientSocket))) 00162 { 00163 printf("Failed to accept client: %s\r\n", Wiconnect::getWiconnectResultStr(result)); 00164 continue; 00165 } 00166 printf("Client connected: %s:%d\r\n", clientSocket.getHost(), clientSocket.getRemotePort()); 00167 00168 //------------------------------------------------------------------------- 00169 // STEP 6: Receive data from client 00170 //------------------------------------------------------------------------- 00171 00172 uint8_t *dataPtr; // pointer to client socket's internal RX buffer 00173 uint16_t readSize; // will contain number of bytes available in RX buffer 00174 if(WICONNECT_FAILED(result, clientSocket.read(&dataPtr, &readSize))) 00175 { 00176 printf("Failed to read data from client: %s\r\n", Wiconnect::getWiconnectResultStr(result)); 00177 clientSocket.close(); 00178 continue; 00179 } 00180 00181 printf("From client: %s\r\n", dataPtr); 00182 00183 00184 //------------------------------------------------------------------------- 00185 // STEP 7: Send data to client 00186 //------------------------------------------------------------------------- 00187 00188 if(WICONNECT_FAILED(result, clientSocket.puts("Hello client!!\r\n"))) 00189 { 00190 printf("Failed to send data to client: %s\r\n", Wiconnect::getWiconnectResultStr(result)); 00191 clientSocket.close(); 00192 continue; 00193 } 00194 00195 //------------------------------------------------------------------------- 00196 // STEP 8: Close client connection 00197 //------------------------------------------------------------------------- 00198 00199 clientSocket.close(); 00200 } 00201 00202 } 00203
Generated on Tue Jul 12 2022 21:46:48 by
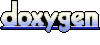