
UDP echo client using the WiConnect Library and mbed UDP Socket API.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 00002 #include "Wiconnect.h" 00003 #include "target_config.h" 00004 00005 #define NETWORK_SSID "<YOUR NETWORK SSID HERE>" 00006 #define NETWORK_PASSWORD "<YOUR NETWORK PASSWORD HERE>" 00007 00008 #define ECHO_SERVER_ADDRESS "<ECHO Server's IP Addres HERE>" 00009 #define ECHO_SERVER_PORT 7 00010 00011 00012 00013 static Serial consoleSerial(STDIO_UART_TX, STDIO_UART_RX); 00014 00015 static char in_buffer[256]; 00016 00017 int main() 00018 { 00019 WiconnectResult result; 00020 SerialConfig serialConfig(WICONNECT_RX_PIN, WICONNECT_TX_PIN, 256); 00021 Wiconnect wiconnect(serialConfig, 256, NULL, WICONNECT_RESET_PIN); 00022 00023 consoleSerial.baud(115200); 00024 printf("Initializing WiConnect...\r\n"); 00025 00026 if(WICONNECT_FAILED(result, wiconnect.init(true))) 00027 { 00028 printf("Failed to initialize Wiconnect: %s\r\n", Wiconnect::getWiconnectResultStr(result)); 00029 if(result == WICONNECT_FIRMWARE_OUTDATED) 00030 { 00031 printf("** The WiFi firmware is not supported. Run the ota example to update the firmware:\r\n"); 00032 printf("https://developer.mbed.org/teams/ACKme/code/wiconnect-ota_example\r\n\r\n"); 00033 } 00034 for(;;); 00035 } 00036 else if(WICONNECT_FAILED(result, wiconnect.join(NETWORK_SSID, NETWORK_PASSWORD))) 00037 { 00038 printf("Failed to join network: %s\r\n", Wiconnect::getWiconnectResultStr(result)); 00039 for(;;); 00040 } 00041 00042 00043 UDPSocket sock; 00044 sock.init(); 00045 00046 Endpoint echo_server; 00047 echo_server.set_address(ECHO_SERVER_ADDRESS, ECHO_SERVER_PORT); 00048 00049 00050 char out_buffer[] = "Hello World\n"; 00051 if(sock.sendTo(echo_server, out_buffer, sizeof(out_buffer)) == -1) 00052 { 00053 printf("Failed to send data\r\n"); 00054 for(;;); 00055 } 00056 00057 00058 int n = sock.receiveFrom(echo_server, in_buffer, sizeof(in_buffer)); 00059 if(n == -1) 00060 { 00061 printf("Failed to receive data\r\n"); 00062 for(;;); 00063 } 00064 in_buffer[n] = '\0'; 00065 printf("%s", in_buffer); 00066 00067 00068 sock.close(); 00069 wiconnect.deinit(); 00070 00071 printf("Finished!"); 00072 while(true) {} 00073 }
Generated on Thu Jul 14 2022 03:04:30 by
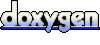