
Simple app demonstrating network join feature of WiConnect Host Library.
Embed:
(wiki syntax)
Show/hide line numbers
example.cpp
00001 /** 00002 * @example join/example.cpp 00003 * 00004 * This is an example of using the join network API to 00005 * join a WiFi network. 00006 * 00007 * It works as follows: 00008 * 1. Instantiate the WiConnect Library 00009 * 2. Initiate Communication with WiFi Module 00010 * 3. Join a network using the specified parameters 00011 * 4. That's it! 00012 * 00013 * 00014 */ 00015 00016 00017 /****************************************************************************** 00018 * Example Variables 00019 */ 00020 00021 // This is the name of your WiFi network 00022 // Look for this name in your WiFi settings 00023 // (e.g. your phone's list of WiFi networks in the WiFi settings menu) 00024 // tip: add double-quotes around SSID to add spaces to name 00025 #define NETWORK_SSID "\"<YOUR NETWORK NAME HERE>\"" 00026 00027 // This is the password of your WiFi network 00028 // Leave as empty string (e.g "") to connect to OPEN network 00029 #define NETWORK_PASSWORD "\"<YOUR NETWORK PASSWORD HERE>\"" 00030 00031 00032 /****************************************************************************** 00033 * Includes 00034 */ 00035 00036 // include C library headers 00037 #include <stdio.h> // needed for printf 00038 00039 // include target specific defines 00040 #include "target_config.h" 00041 // include the Wiconnect Host Library API header 00042 #include "Wiconnect.h" 00043 00044 00045 00046 /****************************************************************************** 00047 * Global Defines 00048 */ 00049 00050 00051 // Serial used for printfs to terminal (i.e. NOT used for WiConnect) 00052 static Serial consoleSerial(STDIO_UART_TX, STDIO_UART_RX); 00053 00054 00055 /****************************************************************************** 00056 * Starting point of application 00057 */ 00058 int main(int argc, char **argv) 00059 { 00060 WiconnectResult result; 00061 00062 consoleSerial.baud(115200); // console terminal to 115200 baud 00063 00064 //------------------------------------------------------------------------- 00065 // STEP 1: Instantiate WiConnect Library 00066 //------------------------------------------------------------------------- 00067 00068 // Setup wiconnect serial interface configuration 00069 // Here we only specify the rx buffer size and not rx buffer pointer, this means 00070 // The serial RX buffer will be dynamically allocated 00071 SerialConfig serialConfig(WICONNECT_RX_PIN, WICONNECT_TX_PIN, 256, NULL); 00072 00073 // Instantiate the Wiconnect library 00074 // Here we only specify the buffer size and not buffer pointer, this means 00075 // The internal buffer will be dynamically allocated 00076 Wiconnect wiconnect(serialConfig, 256, NULL, WICONNECT_RESET_PIN); 00077 00078 //------------------------------------------------------------------------- 00079 // STEP 2: Initiate Communication with WiFi Module 00080 //------------------------------------------------------------------------- 00081 00082 printf("Initializing WiConnect Library...\r\n"); 00083 00084 // Initialize communication with WiFi module 00085 if(WICONNECT_FAILED(result, wiconnect.init(true))) 00086 { 00087 if(result == WICONNECT_FIRMWARE_OUTDATED) 00088 { 00089 printf("** The WiFi firmware is not supported. Run the ota example to update the firmware:\r\n"); 00090 printf("https://developer.mbed.org/teams/ACKme/code/wiconnect-ota_example\r\n\r\n"); 00091 } 00092 else 00093 { 00094 printf("Failed to initialize communication with WiFi module!\r\n" 00095 "Make sure the wires are connected correctly\r\n"); 00096 } 00097 for(;;); // infinite loop 00098 } 00099 00100 00101 //------------------------------------------------------------------------- 00102 // STEP 3: Join a network using the specified parameters 00103 //------------------------------------------------------------------------- 00104 00105 printf("Joining network: %s....\r\n", NETWORK_SSID); 00106 00107 if(wiconnect.join(NETWORK_SSID, NETWORK_PASSWORD) != WICONNECT_SUCCESS) 00108 { 00109 printf("Failed to send join command\r\n"); 00110 for(;;); // infinite loop 00111 } 00112 00113 //------------------------------------------------------------------------- 00114 // STEP 4: Done! 00115 //------------------------------------------------------------------------- 00116 00117 printf("IP Address: %s\r\n", wiconnect.getIpAddress()); 00118 printf("Network join example has completed!\r\n"); 00119 00120 while(true){} // infinite loop 00121 } 00122
Generated on Sun Jul 17 2022 01:46:07 by
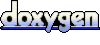