
Program and supporting libraries for cat laser tower bluetooth functionality and auto modes.
Dependencies: mbed sMotor HC_SR04_Ultrasonic_Library
main.cpp
00001 #include "mbed.h" 00002 #include "sMotor.h" 00003 #include "ultrasonic.h" 00004 00005 Serial pc(USBTX, USBRX); 00006 Serial Blue(p28,p27); 00007 sMotor motor(p9, p10, p11, p12); // creates new stepper motor: IN1, IN2, IN3, IN4 00008 DigitalOut laser(p20); 00009 Timer t; 00010 00011 int step_speed = 1100; // set default motor speed 00012 volatile int dir = 0; //0 is CW, 1 is CCW 00013 00014 volatile int C = 30; 00015 volatile int numstep; // defines basic turn distance -- 256 steps == 360 degrees 00016 volatile bool stationary = 1; 00017 volatile bool regular = 0; 00018 volatile bool isrand = 0; 00019 volatile bool manual = 0; 00020 volatile bool sonic = 0; 00021 volatile bool trigger = 0; 00022 volatile int bnum = 0; 00023 volatile int bhit; 00024 volatile int power = 1; 00025 //state used to remember previous characters read in a button message 00026 enum statetype {start = 0, got_exclm, got_B, got_num, got_hit}; 00027 statetype state = start; 00028 00029 //Interrupt routine to determine distance 00030 void dist(int distance) { 00031 //put code here to execute when the distance has changed 00032 printf("Distance %d mm\r\n", distance); 00033 if (distance < 500) { 00034 trigger = 1; 00035 printf("where the kitty cat?\r\n"); 00036 } 00037 00038 } 00039 //Define ultrasonic sensor 00040 ultrasonic mu(p6, p7, .5, 1, &dist); 00041 00042 //Interrupt routine to parse message with one new character per serial RX interrupt 00043 void parse_message() { 00044 switch (state) { 00045 case start: 00046 if (Blue.getc()=='!') state = got_exclm; 00047 else state = start; 00048 break; 00049 case got_exclm: 00050 if (Blue.getc() == 'B') state = got_B; 00051 else state = start; 00052 break; 00053 case got_B: 00054 bnum = Blue.getc(); 00055 state = got_num; 00056 break; 00057 case got_num: 00058 bhit = Blue.getc(); 00059 state = got_hit; 00060 break; 00061 case got_hit: 00062 if (Blue.getc() == char(~('!' + ' B' + bnum + bhit))) { 00063 switch (bnum) { 00064 case '1': //number button 1 00065 regular = isrand = manual = sonic = 0; 00066 stationary = 1; 00067 printf("! B %d %d: Button 1 hit!\r\n", bnum, bhit); 00068 break; 00069 case '2': //number button 2 00070 stationary = manual = isrand = sonic = 0; 00071 regular = 1; 00072 numstep = C; 00073 printf("! B %d %d: Button 2 hit!\r\n", bnum, bhit); 00074 break; 00075 case '3': //number button 3 00076 stationary = regular = manual = sonic = 0; 00077 isrand = 1; 00078 printf("! B %d %d: Button 3 hit!\r\n", bnum, bhit); 00079 break; 00080 case '4': //number button 4 00081 regular = isrand = manual = stationary = 0; 00082 sonic = 1; 00083 printf("! B %d %d: Button 4 hit! Going ultrasonic.\r\n", bnum, bhit); 00084 break; 00085 case '5': //up arrow 00086 power = 1; 00087 printf("! B %d %d: Up arrow hit!\r\n", bnum, bhit); 00088 break; 00089 case '6': //down arrow 00090 power = 0; 00091 printf("! B %d %d: Down arrow hit!\r\n", bnum, bhit); 00092 break; 00093 case '7': 00094 stationary = regular = isrand = sonic = 0; 00095 manual = 1; 00096 dir = 0; 00097 printf("! B %d %d: Left arrow hit!\r\n", bnum, bhit); 00098 break; 00099 case '8': 00100 stationary = regular = isrand = sonic = 0; 00101 manual = 1; 00102 dir = 1; 00103 printf("! B %d %d: Right arrow hit!\r\n", bnum, bhit); 00104 break; 00105 } 00106 } 00107 default: 00108 Blue.getc(); 00109 state = start; 00110 } 00111 } 00112 00113 int main() { 00114 00115 // Screen Menu 00116 printf("Default Speed: %d\n\r", step_speed); 00117 printf("Use Adafruit Bluefruit buttons to select mode\n\r"); 00118 00119 // attach interrupt function for each new Bluetooth serial character 00120 Blue.attach(&parse_message,Serial::RxIrq); 00121 00122 int temp1 = 0; 00123 int temp2 = 0; 00124 00125 while (1) { 00126 laser = power; 00127 00128 //IF STATIONARY, DONT DO ANYTHING, OTHERWISE: 00129 if (stationary != 1) { 00130 00131 //IF REGULAR, TURN BACK AND FORTH WITH C 00132 if (regular == 1) { 00133 dir = 1 - dir; 00134 motor.step(numstep, dir, step_speed); 00135 } 00136 //IF RANDOM, TURN RANDOMLY BASED ON C 00137 else if (isrand == 1) { 00138 temp2 = rand() % C+1; 00139 numstep = temp2 - temp1; 00140 if (numstep < 0) { 00141 dir = 0; 00142 } else if (numstep > 0) { 00143 dir = 1; 00144 } 00145 temp1 = temp2; 00146 numstep = abs(numstep); 00147 motor.step(numstep, dir, step_speed); 00148 } 00149 //IF MANUAL, USE DIRECTION TO TURN 00150 else if (manual == 1) { 00151 dir = dir; 00152 numstep = C; 00153 motor.step(numstep, dir, step_speed); 00154 manual = 0; 00155 stationary = 1; 00156 } 00157 //GOING ULTRASONIC 00158 else if (sonic == 1) { 00159 laser = 0; 00160 mu.startUpdates();//start measuring the distance 00161 while (sonic) { 00162 mu.checkDistance(); 00163 if (trigger) { 00164 t.start(); 00165 while (t.read() < 60.0 && sonic == 1) { 00166 laser = 1; 00167 printf("%f seconds\n", t.read()); 00168 numstep = rand() % C+1; 00169 dir = !dir; 00170 motor.step(numstep, dir, step_speed); 00171 } 00172 laser = 0; 00173 trigger = 0; 00174 t.stop(); 00175 t.reset(); 00176 } 00177 } 00178 mu.pauseUpdates();//stop measureing the distance 00179 } 00180 } 00181 } 00182 }
Generated on Tue Aug 30 2022 06:45:06 by
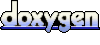