this code is completely restructured, but should do the same thing. did not want to directly commit, since it may not work at all. compiles though.
Dependencies: AVEncoder mbed-src-AV
Fork of Test by
main.cpp
00001 #include "mbed.h" 00002 00003 #include "pid.h" 00004 #include "sensors.h" 00005 #include "mouse.h" 00006 00007 Ticker Systicker; 00008 Mouse m; 00009 00010 void systick() 00011 { 00012 m.scan(); // get the IR values. 00013 // these values are useful regardless of what mouse_state we are in. 00014 switch (m.mouse_state) 00015 { 00016 case STOPPED: 00017 m.offsetCalc(); 00018 m.pid_reset(); 00019 m.sensor_reset(); 00020 break; 00021 case FORWARD: 00022 if ( m.forward_wall_detected() ) 00023 { 00024 m.mouse_state = STOPPED; 00025 m.turn_direction = LEFT; 00026 return; 00027 } 00028 m.run_pid(); 00029 // TODO we need to start saving walls and stuff. 00030 break; 00031 default: 00032 // don't do anything. 00033 break; 00034 } 00035 } 00036 00037 void setup() 00038 { 00039 Mouse m; 00040 00041 Systicker.attach_us(&systick, 1000); 00042 wait(2); 00043 } 00044 00045 int main() { 00046 setup(); 00047 while(1) { 00048 switch (m.mouse_state) 00049 { 00050 case STOPPED: 00051 m.stop(); 00052 wait(1); 00053 if ( m.turn_direction == INVALID ) 00054 { 00055 m.mouse_state = FORWARD; 00056 } 00057 else 00058 { 00059 m.mouse_state = TURNING; 00060 } 00061 break; 00062 case FORWARD: 00063 // left_forward = left_speed / left_max_speed; 00064 // left_reverse = 0; 00065 // right_forward = right_speed / right_max_speed; 00066 // right_reverse = 0; 00067 break; 00068 case TURNING: 00069 m.turn(); 00070 m.mouse_state = STOPPED; 00071 m.turn_direction = INVALID; 00072 break; 00073 case MOVECELL: 00074 m.move_cell(); 00075 m.mouse_state = STOPPED; 00076 break; 00077 default: 00078 // idk lmao. show some error message? 00079 break; 00080 } 00081 } 00082 }
Generated on Tue Jul 12 2022 23:49:37 by
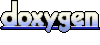