
For the 8x8x8 RGB LEDCube
Dependencies: 3DSnake CubeObj LEDCUBE_RGB_888 SPIShiftReg mbed
Fork of 888LEDCUBE_RGB by
FireWorkr.h
00001 #pragma once 00002 00003 #include "mbed.h" 00004 #include "CubeObj.h" 00005 #include<stdlib.h> 00006 00007 #define ParticleNUM 50 00008 00009 class Fireworks{ 00010 public: 00011 bool isWork; 00012 int sx, sy, bz; 00013 float z; 00014 COL cols[2]; 00015 00016 CubeObj ff[ParticleNUM]; 00017 00018 Fireworks(){ 00019 isWork = false; 00020 sx = 0; 00021 sy = 0; 00022 z = 0; 00023 } 00024 void SetColor(COL c1, COL c2){ 00025 cols[0] = c1; 00026 cols[1] = c2; 00027 } 00028 void explSet(int x, int y, int z, float s = 0.8){ 00029 for(int i = 0; i < ParticleNUM; i++){ 00030 if(i % 2 == 0)ff[i].set(s, y, z, cols[0]); 00031 else ff[i].set(x, y, z, cols[1]); 00032 ff[i].set( 00033 (float)(rand()%360)*(PI/180), 00034 (float)(rand()%360)*(PI/180), 00035 (float)(rand()%((int)350))/1000); 00036 } 00037 } 00038 void explosion(){ 00039 for(int i = 0; i < ParticleNUM; i++){ 00040 ff[i].go(); 00041 ff[i].friction(1.05); 00042 ff[i].gravity(0.0005); 00043 } 00044 } 00045 void put(int px, int py, int burnz){ 00046 if(!isWork){ 00047 sx = px; 00048 sy = py; 00049 bz = burnz; 00050 z = 0; 00051 isWork = true; 00052 explSet(sx, sy, bz); 00053 } 00054 } 00055 void Update(){ 00056 if(z > bz){ 00057 explosion(); 00058 z++; 00059 if(z >= bz*50)isWork = false; 00060 } 00061 else{ 00062 if(z <= bz)PutPixel(sx, sy, z, 7); 00063 z += 0.1f; 00064 } 00065 } 00066 };
Generated on Fri Jul 15 2022 03:19:27 by
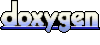