
Create a project for TT_Mxx.
Embed:
(wiki syntax)
Show/hide line numbers
MPL3115A2.h
00001 #ifndef MPL3115A2_H 00002 #define MPL3115A2_H 00003 00004 #include "mbed.h" 00005 00006 00007 #define MPL3115A2_DEFAULT_ADDRESS (0x60 << 1) 00008 00009 // Oversampling value and minimum time between sample 00010 #define OVERSAMPLE_RATIO_1 0 // 6 ms 00011 #define OVERSAMPLE_RATIO_2 1 // 10 ms 00012 #define OVERSAMPLE_RATIO_4 2 // 18 ms 00013 #define OVERSAMPLE_RATIO_8 3 // 34 ms 00014 #define OVERSAMPLE_RATIO_16 4 // 66 ms 00015 #define OVERSAMPLE_RATIO_32 5 // 130 ms 00016 #define OVERSAMPLE_RATIO_64 6 // 258 ms 00017 #define OVERSAMPLE_RATIO_128 7 // 512 ms 00018 00019 /* Mode */ 00020 #define ALTIMETER_MODE 1 00021 #define BAROMETRIC_MODE 2 00022 00023 /** 00024 * MPL3115A2 Altimeter example 00025 * 00026 * @code 00027 * #include "mbed.h" 00028 * #include "MPL3115A2.h" 00029 * 00030 * #define MPL3115A2_I2C_ADDRESS (0x60<<1) 00031 * MPL3115A2 wigo_sensor1(PTE0, PTE1, MPL3115A2_I2C_ADDRESS); 00032 * Serial pc(USBTX, USBRX); 00033 * 00034 * // pos [0] = altitude or pressure value 00035 * // pos [1] = temperature value 00036 * float sensor_data[2]; 00037 * 00038 * int main(void) { 00039 * 00040 * pc.baud( 230400); 00041 * pc.printf("MPL3115A2 Altimeter mode. [%d]\r\n", wigo_sensor1.getDeviceID()); 00042 * 00043 * wigo_sensor1.Oversample_Ratio( OVERSAMPLE_RATIO_32); 00044 * 00045 * while(1) { 00046 * // 00047 * if ( wigo_sensor1.isDataAvailable()) { 00048 * wigo_sensor1.getAllData( &sensor_data[0]); 00049 * pc.printf("\tAltitude: %f\tTemperature: %f\r\n", sensor_data[0], sensor_data[1]); 00050 * } 00051 * // 00052 * wait( 0.001); 00053 * } 00054 * 00055 * } 00056 * @endcode 00057 */ 00058 class MPL3115A2 00059 { 00060 public: 00061 /** 00062 * MPL3115A2 constructor 00063 * 00064 * @param sda SDA pin 00065 * @param sdl SCL pin 00066 * @param addr addr of the I2C peripheral 00067 * @param int1 InterruptIn 00068 * @param int2 InterruptIn 00069 * 00070 * Interrupt schema: 00071 * 00072 * * The Altitude Trigger use the IRQ1 - Altitude Trigger -> MPL3115A2_Int1.fall -> AltitudeTrg_IRQ -> MPL3115A2_usr1_fptr 00073 * 00074 * * The Data ready use the IRQ2 - Data Ready -> MPL3115A2_Int2.fall -> DataReady_IRQ -> MPL3115A2_usr2_fptr 00075 */ 00076 MPL3115A2(PinName sda, PinName scl, int addr, PinName int1, PinName int2); 00077 00078 /** 00079 * Get the value of the WHO_AM_I register 00080 * 00081 * @returns DEVICE_ID value == 0xC4 00082 */ 00083 uint8_t getDeviceID(); 00084 00085 /** 00086 * Return the STATUS register value 00087 * 00088 * @returns STATUS register value 00089 */ 00090 unsigned char getStatus( void); 00091 00092 /** 00093 * Get the altimeter value 00094 * 00095 * @returns altimeter value as float 00096 */ 00097 float getAltimeter( void); 00098 00099 /** 00100 * Get the altimeter value in raw mode 00101 * 00102 * @param dt pointer to unsigned char array 00103 * @returns 1 if data are available, 0 if not. 00104 */ 00105 unsigned int getAltimeterRaw( unsigned char *dt); 00106 00107 /** 00108 * Get the pressure value 00109 * 00110 * @returns pressure value as float 00111 */ 00112 float getPressure( void); 00113 00114 /** 00115 * Get the pressure value in raw mode 00116 * 00117 * @param dt pointer to unsigned char array 00118 * @returns 1 if data are available, 0 if not. 00119 */ 00120 unsigned int getPressureRaw( unsigned char *dt); 00121 00122 /** 00123 * Get the temperature value 00124 * 00125 * @returns temperature value as float 00126 */ 00127 float getTemperature( void); 00128 00129 /** 00130 * Get the temperature value in raw mode 00131 * 00132 * @param dt pointer to unsigned char array 00133 * @returns 1 if data are available, 0 if not. 00134 */ 00135 unsigned int getTemperatureRaw( unsigned char *dt); 00136 00137 /** 00138 * Set the Altimeter Mode 00139 * 00140 * @returns none 00141 */ 00142 void Altimeter_Mode( void); 00143 00144 /** 00145 * Set the Barometric Mode 00146 * 00147 * @returns none 00148 */ 00149 void Barometric_Mode( void); 00150 00151 /** 00152 * Get the altimeter or pressure and temperature values 00153 * 00154 * @param array of float f[2] 00155 * @returns 0 no data available, 1 for data available 00156 */ 00157 unsigned int getAllData( float *f); 00158 00159 /** 00160 * Get the altimeter or pressure and temperature values and the delta values 00161 * 00162 * @param array of float f[2], array of float d[2] 00163 * @returns 0 no data available, 1 for data available 00164 */ 00165 unsigned int getAllData( float *f, float *d); 00166 00167 /** 00168 * Get the altimeter or pressure and temperature captured maximum value 00169 * 00170 * @param array of float f[2] 00171 * @returns 0 no data available, 1 for data available 00172 */ 00173 void getAllMaximumData( float *f); 00174 00175 /** 00176 * Get the altimeter or pressure and temperature captured minimum value 00177 * 00178 * @param array of float f[2] 00179 * @returns 0 no data available, 1 for data available 00180 */ 00181 void getAllMinimumData( float *f); 00182 00183 /** 00184 * Get the altimeter or pressure, and temperature values in raw mode 00185 * 00186 * @param array of unsigned char[5] 00187 * @returns 1 if data are available, 0 if not. 00188 */ 00189 unsigned int getAllDataRaw( unsigned char *dt); 00190 00191 /** 00192 * Return if there are date available 00193 * 00194 * @return 0 for no data available, bit0 set for Temp data available, bit1 set for Press/Alti data available 00195 * bit2 set for both Temp and Press/Alti data available 00196 */ 00197 unsigned int isDataAvailable( void); 00198 00199 /** 00200 * Set the oversampling rate value 00201 * 00202 * @param oversampling values. See MPL3115A2.h 00203 * @return none 00204 */ 00205 void Oversample_Ratio( unsigned int ratio); 00206 00207 /** 00208 * Configure the sensor to streaming data using Interrupt 00209 * 00210 * @param user functin callback, oversampling values. See MPL3115A2.h 00211 * @return none 00212 */ 00213 void DataReady( void(*fptr)(void), unsigned char OS); 00214 00215 /** 00216 * Configure the sensor to generate an Interrupt crossing the center threshold 00217 * 00218 * @param user functin callback, level in meter 00219 * @return none 00220 */ 00221 void AltitudeTrigger( void(*fptr)(void), unsigned short level); 00222 00223 /** 00224 * Soft Reset 00225 * 00226 * @param none 00227 * @return none 00228 */ 00229 void Reset( void); 00230 00231 /** 00232 * Configure the Pressure offset. 00233 * Pressure user accessible offset trim value expressed as an 8-bit 2's complement number. 00234 * The user offset registers may be adjusted to enhance accuracy and optimize the system performance. 00235 * Range is from -512 to +508 Pa, 4 Pa per LSB. 00236 * In RAW output mode no scaling or offsets will be applied in the digital domain 00237 * 00238 * @param offset 00239 * @return none 00240 */ 00241 void SetPressureOffset( char offset); 00242 00243 /** 00244 * Configure the Temperature offset. 00245 * Temperature user accessible offset trim value expressed as an 8-bit 2's complement number. 00246 * The user offset registers may be adjusted to enhance accuracy and optimize the system performance. 00247 * Range is from -8 to +7.9375°C 0.0625°C per LSB. 00248 * In RAW output mode no scaling or offsets will be applied in the digital domain 00249 * 00250 * @param offset 00251 * @return none 00252 */ 00253 void SetTemperatureOffset( char offset); 00254 00255 /** 00256 * Configure the Altitude offset. 00257 * Altitude Data User Offset Register (OFF_H) is expressed as a 2’s complement number in meters. 00258 * The user offset register provides user adjustment to the vertical height of the Altitude output. 00259 * The range of values are from -128 to +127 meters. 00260 * In RAW output mode no scaling or offsets will be applied in the digital domain 00261 * 00262 * @param offset 00263 * @return none 00264 */ 00265 void SetAltitudeOffset( char offset); 00266 00267 /** 00268 * @brief Get current device's mode. 00269 * @return [ALTIMETER_MODE] or [BAROMETRIC_MODE] 00270 */ 00271 uint8_t getMode(); 00272 private: 00273 I2C m_i2c; 00274 int m_addr; 00275 InterruptIn MPL3115A2_Int1; 00276 InterruptIn MPL3115A2_Int2; 00277 00278 unsigned char MPL3115A2_mode; 00279 unsigned char MPL3115A2_oversampling; 00280 void DataReady_IRQ( void); 00281 void AltitudeTrg_IRQ( void); 00282 00283 /** Set the device in active mode 00284 */ 00285 void Active( void); 00286 00287 /** Set the device in standby mode 00288 */ 00289 void Standby( void); 00290 00291 /** Get the altimiter value from the sensor. 00292 * 00293 * @param reg the register from which read the data. 00294 * Can be: REG_ALTIMETER_MSB for altimeter value 00295 * REG_ALTI_MIN_MSB for the minimum value captured 00296 * REG_ALTI_MAX_MSB for the maximum value captured 00297 */ 00298 float getAltimeter( unsigned char reg); 00299 00300 /** Get the pressure value from the sensor. 00301 * 00302 * @param reg the register from which read the data. 00303 * Can be: REG_PRESSURE_MSB for altimeter value 00304 * REG_PRES_MIN_MSB for the minimum value captured 00305 * REG_PRES_MAX_MSB for the maximum value captured 00306 */ 00307 float getPressure( unsigned char reg); 00308 00309 /** Get the altimiter value from the sensor. 00310 * 00311 * @param reg the register from which read the data. 00312 * Can be: REG_TEMP_MSB for altimeter value 00313 * REG_TEMP_MIN_MSB for the minimum value captured 00314 * REG_TEMP_MAX_MSB for the maximum value captured 00315 */ 00316 float getTemperature( unsigned char reg); 00317 00318 void readRegs(int addr, uint8_t * data, int len); 00319 void writeRegs(uint8_t * data, int len); 00320 00321 }; 00322 00323 #endif 00324
Generated on Wed Jul 13 2022 20:20:49 by
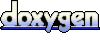